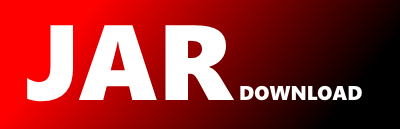
org.wildfly.swarm.config.undertow.ServletContainer Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.servlet_container.MimeMapping;
import org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile;
import org.wildfly.swarm.config.undertow.servlet_container.PersistentSessionsSetting;
import org.wildfly.swarm.config.undertow.servlet_container.JspSetting;
import org.wildfly.swarm.config.undertow.servlet_container.WebsocketsSetting;
import org.wildfly.swarm.config.undertow.servlet_container.SessionCookieSetting;
/**
* A servlet container
*/
@ResourceType("servlet-container")
public class ServletContainer {
private String key;
private Boolean allowNonStandardWrappers;
private String defaultBufferCache;
private String defaultEncoding;
private Integer defaultSessionTimeout;
private Boolean directoryListing;
private Boolean disableCachingForSecuredPages;
private Boolean eagerFilterInitialization;
private Boolean ignoreFlush;
private Integer maxSessions;
private Boolean proactiveAuthentication;
private Integer sessionIdLength;
private String stackTraceOnError;
private Boolean useListenerEncoding;
private ServletContainerResources subresources = new ServletContainerResources();
private PersistentSessionsSetting persistentSessionsSetting;
private JspSetting jspSetting;
private WebsocketsSetting websocketsSetting;
private SessionCookieSetting sessionCookieSetting;
public ServletContainer(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* If true then request and response wrappers that do not extend the standard wrapper classes can be used
*/
@ModelNodeBinding(detypedName = "allow-non-standard-wrappers")
public Boolean allowNonStandardWrappers() {
return this.allowNonStandardWrappers;
}
/**
* If true then request and response wrappers that do not extend the standard wrapper classes can be used
*/
@SuppressWarnings("unchecked")
public ServletContainer allowNonStandardWrappers(Boolean value) {
this.allowNonStandardWrappers = value;
return (ServletContainer) this;
}
/**
* The buffer cache to use for caching static resources
*/
@ModelNodeBinding(detypedName = "default-buffer-cache")
public String defaultBufferCache() {
return this.defaultBufferCache;
}
/**
* The buffer cache to use for caching static resources
*/
@SuppressWarnings("unchecked")
public ServletContainer defaultBufferCache(String value) {
this.defaultBufferCache = value;
return (ServletContainer) this;
}
/**
* Default encoding to use for all deployed applications
*/
@ModelNodeBinding(detypedName = "default-encoding")
public String defaultEncoding() {
return this.defaultEncoding;
}
/**
* Default encoding to use for all deployed applications
*/
@SuppressWarnings("unchecked")
public ServletContainer defaultEncoding(String value) {
this.defaultEncoding = value;
return (ServletContainer) this;
}
/**
* The default session timeout (in minutes) for all applications deployed in the container.
*/
@ModelNodeBinding(detypedName = "default-session-timeout")
public Integer defaultSessionTimeout() {
return this.defaultSessionTimeout;
}
/**
* The default session timeout (in minutes) for all applications deployed in the container.
*/
@SuppressWarnings("unchecked")
public ServletContainer defaultSessionTimeout(Integer value) {
this.defaultSessionTimeout = value;
return (ServletContainer) this;
}
/**
* If directory listing should be enabled for default servlets.
*/
@ModelNodeBinding(detypedName = "directory-listing")
public Boolean directoryListing() {
return this.directoryListing;
}
/**
* If directory listing should be enabled for default servlets.
*/
@SuppressWarnings("unchecked")
public ServletContainer directoryListing(Boolean value) {
this.directoryListing = value;
return (ServletContainer) this;
}
/**
* If Undertow should set headers to disable caching for secured paged. Disabling this can cause security problems, as sensitive pages may be cached by an intermediary.
*/
@ModelNodeBinding(detypedName = "disable-caching-for-secured-pages")
public Boolean disableCachingForSecuredPages() {
return this.disableCachingForSecuredPages;
}
/**
* If Undertow should set headers to disable caching for secured paged. Disabling this can cause security problems, as sensitive pages may be cached by an intermediary.
*/
@SuppressWarnings("unchecked")
public ServletContainer disableCachingForSecuredPages(Boolean value) {
this.disableCachingForSecuredPages = value;
return (ServletContainer) this;
}
/**
* If true undertow calls filter init() on deployment start rather than when first requested.
*/
@ModelNodeBinding(detypedName = "eager-filter-initialization")
public Boolean eagerFilterInitialization() {
return this.eagerFilterInitialization;
}
/**
* If true undertow calls filter init() on deployment start rather than when first requested.
*/
@SuppressWarnings("unchecked")
public ServletContainer eagerFilterInitialization(Boolean value) {
this.eagerFilterInitialization = value;
return (ServletContainer) this;
}
/**
* Ignore flushes on the servlet output stream. In most cases these just hurt performance for no good reason.
*/
@ModelNodeBinding(detypedName = "ignore-flush")
public Boolean ignoreFlush() {
return this.ignoreFlush;
}
/**
* Ignore flushes on the servlet output stream. In most cases these just hurt performance for no good reason.
*/
@SuppressWarnings("unchecked")
public ServletContainer ignoreFlush(Boolean value) {
this.ignoreFlush = value;
return (ServletContainer) this;
}
/**
* The maximum number of sessions that can be active at one time
*/
@ModelNodeBinding(detypedName = "max-sessions")
public Integer maxSessions() {
return this.maxSessions;
}
/**
* The maximum number of sessions that can be active at one time
*/
@SuppressWarnings("unchecked")
public ServletContainer maxSessions(Integer value) {
this.maxSessions = value;
return (ServletContainer) this;
}
/**
* If proactive authentication should be used. If this is true a user will always be authenticated if credentials are present.
*/
@ModelNodeBinding(detypedName = "proactive-authentication")
public Boolean proactiveAuthentication() {
return this.proactiveAuthentication;
}
/**
* If proactive authentication should be used. If this is true a user will always be authenticated if credentials are present.
*/
@SuppressWarnings("unchecked")
public ServletContainer proactiveAuthentication(Boolean value) {
this.proactiveAuthentication = value;
return (ServletContainer) this;
}
/**
* The length of the generated session ID. Longer session ID's are more secure.
*/
@ModelNodeBinding(detypedName = "session-id-length")
public Integer sessionIdLength() {
return this.sessionIdLength;
}
/**
* The length of the generated session ID. Longer session ID's are more secure.
*/
@SuppressWarnings("unchecked")
public ServletContainer sessionIdLength(Integer value) {
this.sessionIdLength = value;
return (ServletContainer) this;
}
/**
* If an error page with the stack trace should be generated on error. Values are all, none and local-only
*/
@ModelNodeBinding(detypedName = "stack-trace-on-error")
public String stackTraceOnError() {
return this.stackTraceOnError;
}
/**
* If an error page with the stack trace should be generated on error. Values are all, none and local-only
*/
@SuppressWarnings("unchecked")
public ServletContainer stackTraceOnError(String value) {
this.stackTraceOnError = value;
return (ServletContainer) this;
}
/**
* Use encoding defined on listener
*/
@ModelNodeBinding(detypedName = "use-listener-encoding")
public Boolean useListenerEncoding() {
return this.useListenerEncoding;
}
/**
* Use encoding defined on listener
*/
@SuppressWarnings("unchecked")
public ServletContainer useListenerEncoding(Boolean value) {
this.useListenerEncoding = value;
return (ServletContainer) this;
}
public ServletContainerResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.undertow.servlet_container.MimeMapping objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.servlet_container.MimeMapping objects.
*/
@SuppressWarnings("unchecked")
public ServletContainer mimeMappings(
List value) {
this.subresources.mimeMappings.addAll(value);
return (ServletContainer) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.servlet_container.MimeMapping object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.servlet_container.MimeMapping to add
* @return this
*/
@SuppressWarnings("unchecked")
public ServletContainer mimeMapping(MimeMapping value) {
this.subresources.mimeMappings.add(value);
return (ServletContainer) this;
}
/**
* Add all org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile objects.
*/
@SuppressWarnings("unchecked")
public ServletContainer welcomeFiles(
List value) {
this.subresources.welcomeFiles.addAll(value);
return (ServletContainer) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile to add
* @return this
*/
@SuppressWarnings("unchecked")
public ServletContainer welcomeFile(WelcomeFile value) {
this.subresources.welcomeFiles.add(value);
return (ServletContainer) this;
}
/**
* Child mutators for ServletContainer
*/
public class ServletContainerResources {
/**
* The servlet container mime mapping config
*/
private List mimeMappings = new java.util.ArrayList<>();
/**
* The welcome file
*/
private List welcomeFiles = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.undertow.servlet_container.MimeMapping resources
* @return the list of resources
*/
@Subresource
public List mimeMappings() {
return this.mimeMappings;
}
/**
* Get the list of org.wildfly.swarm.config.undertow.servlet_container.WelcomeFile resources
* @return the list of resources
*/
@Subresource
public List welcomeFiles() {
return this.welcomeFiles;
}
}
/**
* Session persistence sessions
*/
@Subresource
public PersistentSessionsSetting persistentSessionsSetting() {
return this.persistentSessionsSetting;
}
/**
* Session persistence sessions
*/
@SuppressWarnings("unchecked")
public ServletContainer persistentSessionsSetting(
PersistentSessionsSetting value) {
this.persistentSessionsSetting = value;
return (ServletContainer) this;
}
/**
* JSP container configuration.
*/
@Subresource
public JspSetting jspSetting() {
return this.jspSetting;
}
/**
* JSP container configuration.
*/
@SuppressWarnings("unchecked")
public ServletContainer jspSetting(JspSetting value) {
this.jspSetting = value;
return (ServletContainer) this;
}
/**
* If websockets are enabled for this container
*/
@Subresource
public WebsocketsSetting websocketsSetting() {
return this.websocketsSetting;
}
/**
* If websockets are enabled for this container
*/
@SuppressWarnings("unchecked")
public ServletContainer websocketsSetting(WebsocketsSetting value) {
this.websocketsSetting = value;
return (ServletContainer) this;
}
/**
* Session cookie configuration
*/
@Subresource
public SessionCookieSetting sessionCookieSetting() {
return this.sessionCookieSetting;
}
/**
* Session cookie configuration
*/
@SuppressWarnings("unchecked")
public ServletContainer sessionCookieSetting(SessionCookieSetting value) {
this.sessionCookieSetting = value;
return (ServletContainer) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy