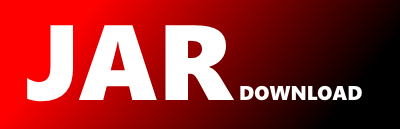
org.wildfly.swarm.config.undertow.configuration.ModCluster Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow.configuration;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer;
/**
* A mod-cluster front end load balancer
*/
@ResourceType("mod-cluster")
public class ModCluster {
private String key;
private Integer advertiseFrequency;
private String advertisePath;
private String advertiseProtocol;
private String advertiseSocketBinding;
private Integer brokenNodeTimeout;
private Integer cachedConnectionsPerThread;
private Integer connectionIdleTimeout;
private Integer connectionsPerThread;
private Integer healthCheckInterval;
private String managementAccessPredicate;
private String managementSocketBinding;
private Integer maxRequestTime;
private Integer requestQueueSize;
private String securityKey;
private String securityRealm;
private Boolean useAlias;
private String worker;
private ModClusterResources subresources = new ModClusterResources();
public ModCluster(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The frequency (in milliseconds) that mod-cluster advertises itself on the network
*/
@ModelNodeBinding(detypedName = "advertise-frequency")
public Integer advertiseFrequency() {
return this.advertiseFrequency;
}
/**
* The frequency (in milliseconds) that mod-cluster advertises itself on the network
*/
@SuppressWarnings("unchecked")
public ModCluster advertiseFrequency(Integer value) {
this.advertiseFrequency = value;
return (ModCluster) this;
}
/**
* The path that mod-cluster is registered under, defaults to /
*/
@ModelNodeBinding(detypedName = "advertise-path")
public String advertisePath() {
return this.advertisePath;
}
/**
* The path that mod-cluster is registered under, defaults to /
*/
@SuppressWarnings("unchecked")
public ModCluster advertisePath(String value) {
this.advertisePath = value;
return (ModCluster) this;
}
/**
* The protocol that is in use, defaults to HTTP
*/
@ModelNodeBinding(detypedName = "advertise-protocol")
public String advertiseProtocol() {
return this.advertiseProtocol;
}
/**
* The protocol that is in use, defaults to HTTP
*/
@SuppressWarnings("unchecked")
public ModCluster advertiseProtocol(String value) {
this.advertiseProtocol = value;
return (ModCluster) this;
}
/**
* The multicast group that is used to advertise
*/
@ModelNodeBinding(detypedName = "advertise-socket-binding")
public String advertiseSocketBinding() {
return this.advertiseSocketBinding;
}
/**
* The multicast group that is used to advertise
*/
@SuppressWarnings("unchecked")
public ModCluster advertiseSocketBinding(String value) {
this.advertiseSocketBinding = value;
return (ModCluster) this;
}
/**
* The amount of time that must elapse before a broken node is removed from the table
*/
@ModelNodeBinding(detypedName = "broken-node-timeout")
public Integer brokenNodeTimeout() {
return this.brokenNodeTimeout;
}
/**
* The amount of time that must elapse before a broken node is removed from the table
*/
@SuppressWarnings("unchecked")
public ModCluster brokenNodeTimeout(Integer value) {
this.brokenNodeTimeout = value;
return (ModCluster) this;
}
/**
* The number of connections that will be kept alive indefinitely
*/
@ModelNodeBinding(detypedName = "cached-connections-per-thread")
public Integer cachedConnectionsPerThread() {
return this.cachedConnectionsPerThread;
}
/**
* The number of connections that will be kept alive indefinitely
*/
@SuppressWarnings("unchecked")
public ModCluster cachedConnectionsPerThread(Integer value) {
this.cachedConnectionsPerThread = value;
return (ModCluster) this;
}
/**
* The amount of time a connection can be idle before it will be closed. Connections will not time out once the pool size is down to the configured minimum (as configured by cached-connections-per-thread)
*/
@ModelNodeBinding(detypedName = "connection-idle-timeout")
public Integer connectionIdleTimeout() {
return this.connectionIdleTimeout;
}
/**
* The amount of time a connection can be idle before it will be closed. Connections will not time out once the pool size is down to the configured minimum (as configured by cached-connections-per-thread)
*/
@SuppressWarnings("unchecked")
public ModCluster connectionIdleTimeout(Integer value) {
this.connectionIdleTimeout = value;
return (ModCluster) this;
}
/**
* The number of connections that will be maintained to backend servers, per IO thread. Defaults to 10.
*/
@ModelNodeBinding(detypedName = "connections-per-thread")
public Integer connectionsPerThread() {
return this.connectionsPerThread;
}
/**
* The number of connections that will be maintained to backend servers, per IO thread. Defaults to 10.
*/
@SuppressWarnings("unchecked")
public ModCluster connectionsPerThread(Integer value) {
this.connectionsPerThread = value;
return (ModCluster) this;
}
/**
* The frequency of health check pings to backend nodes
*/
@ModelNodeBinding(detypedName = "health-check-interval")
public Integer healthCheckInterval() {
return this.healthCheckInterval;
}
/**
* The frequency of health check pings to backend nodes
*/
@SuppressWarnings("unchecked")
public ModCluster healthCheckInterval(Integer value) {
this.healthCheckInterval = value;
return (ModCluster) this;
}
/**
* A predicate that is applied to incoming requests to determine if they can perform mod cluster management commands. Provides additional security on top of what is provided by limiting management to requests that originate from the management-socket-binding
*/
@ModelNodeBinding(detypedName = "management-access-predicate")
public String managementAccessPredicate() {
return this.managementAccessPredicate;
}
/**
* A predicate that is applied to incoming requests to determine if they can perform mod cluster management commands. Provides additional security on top of what is provided by limiting management to requests that originate from the management-socket-binding
*/
@SuppressWarnings("unchecked")
public ModCluster managementAccessPredicate(String value) {
this.managementAccessPredicate = value;
return (ModCluster) this;
}
/**
* The socket binding of the mod_cluster management port. When using mod_cluster two HTTP listeners should be defined, a public one to handle requests, and one bound to the internal network to handle mod cluster commands. This socket binding should correspond to the internal listener, and should not be publicly accessible
*/
@ModelNodeBinding(detypedName = "management-socket-binding")
public String managementSocketBinding() {
return this.managementSocketBinding;
}
/**
* The socket binding of the mod_cluster management port. When using mod_cluster two HTTP listeners should be defined, a public one to handle requests, and one bound to the internal network to handle mod cluster commands. This socket binding should correspond to the internal listener, and should not be publicly accessible
*/
@SuppressWarnings("unchecked")
public ModCluster managementSocketBinding(String value) {
this.managementSocketBinding = value;
return (ModCluster) this;
}
/**
* The max amount of time that a request to a backend node can take before it is killed
*/
@ModelNodeBinding(detypedName = "max-request-time")
public Integer maxRequestTime() {
return this.maxRequestTime;
}
/**
* The max amount of time that a request to a backend node can take before it is killed
*/
@SuppressWarnings("unchecked")
public ModCluster maxRequestTime(Integer value) {
this.maxRequestTime = value;
return (ModCluster) this;
}
/**
* The number of requests that can be queued if the connection pool is full before requests are rejected with a 503
*/
@ModelNodeBinding(detypedName = "request-queue-size")
public Integer requestQueueSize() {
return this.requestQueueSize;
}
/**
* The number of requests that can be queued if the connection pool is full before requests are rejected with a 503
*/
@SuppressWarnings("unchecked")
public ModCluster requestQueueSize(Integer value) {
this.requestQueueSize = value;
return (ModCluster) this;
}
/**
* The security key that is used for the mod-cluster group. All members must use the same security key.
*/
@ModelNodeBinding(detypedName = "security-key")
public String securityKey() {
return this.securityKey;
}
/**
* The security key that is used for the mod-cluster group. All members must use the same security key.
*/
@SuppressWarnings("unchecked")
public ModCluster securityKey(String value) {
this.securityKey = value;
return (ModCluster) this;
}
/**
* The security realm that provides the SSL configuration
*/
@ModelNodeBinding(detypedName = "security-realm")
public String securityRealm() {
return this.securityRealm;
}
/**
* The security realm that provides the SSL configuration
*/
@SuppressWarnings("unchecked")
public ModCluster securityRealm(String value) {
this.securityRealm = value;
return (ModCluster) this;
}
/**
* If an alias check is performed
*/
@ModelNodeBinding(detypedName = "use-alias")
public Boolean useAlias() {
return this.useAlias;
}
/**
* If an alias check is performed
*/
@SuppressWarnings("unchecked")
public ModCluster useAlias(Boolean value) {
this.useAlias = value;
return (ModCluster) this;
}
/**
* The XNIO worker that is used to send the advertise notifications
*/
@ModelNodeBinding(detypedName = "worker")
public String worker() {
return this.worker;
}
/**
* The XNIO worker that is used to send the advertise notifications
*/
@SuppressWarnings("unchecked")
public ModCluster worker(String value) {
this.worker = value;
return (ModCluster) this;
}
public ModClusterResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer objects.
*/
@SuppressWarnings("unchecked")
public ModCluster balancers(
List value) {
this.subresources.balancers.addAll(value);
return (ModCluster) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer to add
* @return this
*/
@SuppressWarnings("unchecked")
public ModCluster balancer(Balancer value) {
this.subresources.balancers.add(value);
return (ModCluster) this;
}
/**
* Child mutators for ModCluster
*/
public class ModClusterResources {
/**
* Runtime representation of a mod_cluster balancer
*/
private List balancers = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.undertow.configuration.mod_cluster.Balancer resources
* @return the list of resources
*/
@Subresource
public List balancers() {
return this.balancers;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy