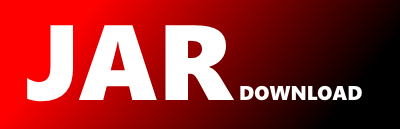
org.wildfly.swarm.config.undertow.configuration.ReverseProxy Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow.configuration;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host;
/**
* A reverse proxy handler
*/
@ResourceType("reverse-proxy")
public class ReverseProxy {
private String key;
private Integer cachedConnectionsPerThread;
private Integer connectionIdleTimeout;
private Integer connectionsPerThread;
private Integer maxRequestTime;
private Integer problemServerRetry;
private Integer requestQueueSize;
private String sessionCookieNames;
private ReverseProxyResources subresources = new ReverseProxyResources();
public ReverseProxy(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The number of connections that will be kept alive indefinitely
*/
@ModelNodeBinding(detypedName = "cached-connections-per-thread")
public Integer cachedConnectionsPerThread() {
return this.cachedConnectionsPerThread;
}
/**
* The number of connections that will be kept alive indefinitely
*/
@SuppressWarnings("unchecked")
public ReverseProxy cachedConnectionsPerThread(Integer value) {
this.cachedConnectionsPerThread = value;
return (ReverseProxy) this;
}
/**
* The amount of time a connection can be idle before it will be closed. Connections will not time out once the pool size is down to the configured minimum (as configured by cached-connections-per-thread)
*/
@ModelNodeBinding(detypedName = "connection-idle-timeout")
public Integer connectionIdleTimeout() {
return this.connectionIdleTimeout;
}
/**
* The amount of time a connection can be idle before it will be closed. Connections will not time out once the pool size is down to the configured minimum (as configured by cached-connections-per-thread)
*/
@SuppressWarnings("unchecked")
public ReverseProxy connectionIdleTimeout(Integer value) {
this.connectionIdleTimeout = value;
return (ReverseProxy) this;
}
/**
* The number of connections that will be maintained to backend servers, per IO thread. Defaults to 10.
*/
@ModelNodeBinding(detypedName = "connections-per-thread")
public Integer connectionsPerThread() {
return this.connectionsPerThread;
}
/**
* The number of connections that will be maintained to backend servers, per IO thread. Defaults to 10.
*/
@SuppressWarnings("unchecked")
public ReverseProxy connectionsPerThread(Integer value) {
this.connectionsPerThread = value;
return (ReverseProxy) this;
}
/**
* The maximum time that a proxy request can be active for, before being killed. Defaults to unlimited
*/
@ModelNodeBinding(detypedName = "max-request-time")
public Integer maxRequestTime() {
return this.maxRequestTime;
}
/**
* The maximum time that a proxy request can be active for, before being killed. Defaults to unlimited
*/
@SuppressWarnings("unchecked")
public ReverseProxy maxRequestTime(Integer value) {
this.maxRequestTime = value;
return (ReverseProxy) this;
}
/**
* Time in seconds to wait before attempting to reconnect to a server that is down
*/
@ModelNodeBinding(detypedName = "problem-server-retry")
public Integer problemServerRetry() {
return this.problemServerRetry;
}
/**
* Time in seconds to wait before attempting to reconnect to a server that is down
*/
@SuppressWarnings("unchecked")
public ReverseProxy problemServerRetry(Integer value) {
this.problemServerRetry = value;
return (ReverseProxy) this;
}
/**
* The number of requests that can be queued if the connection pool is full before requests are rejected with a 503
*/
@ModelNodeBinding(detypedName = "request-queue-size")
public Integer requestQueueSize() {
return this.requestQueueSize;
}
/**
* The number of requests that can be queued if the connection pool is full before requests are rejected with a 503
*/
@SuppressWarnings("unchecked")
public ReverseProxy requestQueueSize(Integer value) {
this.requestQueueSize = value;
return (ReverseProxy) this;
}
/**
* Comma separated list of session cookie names. Generally this will just be JSESSIONID.
*/
@ModelNodeBinding(detypedName = "session-cookie-names")
public String sessionCookieNames() {
return this.sessionCookieNames;
}
/**
* Comma separated list of session cookie names. Generally this will just be JSESSIONID.
*/
@SuppressWarnings("unchecked")
public ReverseProxy sessionCookieNames(String value) {
this.sessionCookieNames = value;
return (ReverseProxy) this;
}
public ReverseProxyResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host objects.
*/
@SuppressWarnings("unchecked")
public ReverseProxy hosts(
List value) {
this.subresources.hosts.addAll(value);
return (ReverseProxy) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host to add
* @return this
*/
@SuppressWarnings("unchecked")
public ReverseProxy host(Host value) {
this.subresources.hosts.add(value);
return (ReverseProxy) this;
}
/**
* Child mutators for ReverseProxy
*/
public class ReverseProxyResources {
/**
* A host that the reverse proxy will forward requests to
*/
private List hosts = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.undertow.configuration.reverse_proxy.Host resources
* @return the list of resources
*/
@Subresource
public List hosts() {
return this.hosts;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy