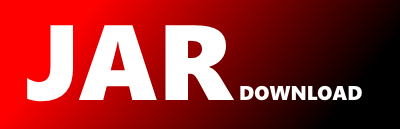
org.wildfly.swarm.config.ejb3.Ejb3 Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.apigen.invocation.Implicit;
import org.wildfly.apigen.invocation.Address;
import org.wildfly.apigen.invocation.ModelNodeBinding;
import java.util.List;
import org.wildfly.apigen.invocation.Subresource;
import org.wildfly.swarm.config.ejb3.subsystem.strictMaxBeanInstancePool.StrictMaxBeanInstancePool;
import org.wildfly.swarm.config.ejb3.subsystem.cache.Cache;
import org.wildfly.swarm.config.ejb3.subsystem.remotingProfile.RemotingProfile;
import org.wildfly.swarm.config.ejb3.subsystem.clusterPassivationStore.ClusterPassivationStore;
import org.wildfly.swarm.config.ejb3.subsystem.passivationStore.PassivationStore;
import org.wildfly.swarm.config.ejb3.subsystem.mdbDeliveryGroup.MdbDeliveryGroup;
import org.wildfly.swarm.config.ejb3.subsystem.threadPool.ThreadPool;
import org.wildfly.swarm.config.ejb3.subsystem.filePassivationStore.FilePassivationStore;
import org.wildfly.swarm.config.ejb3.subsystem.service.TimerService;
import org.wildfly.swarm.config.ejb3.subsystem.service.Remote;
import org.wildfly.swarm.config.ejb3.subsystem.service.Async;
import org.wildfly.swarm.config.ejb3.subsystem.service.Iiop;
/**
* The configuration of the ejb3 subsystem.
*/
@Address("/subsystem=ejb3")
@Implicit
public class Ejb3 {
private String key;
private String defaultClusteredSfsbCache;
private String defaultDistinctName;
private String defaultEntityBeanInstancePool;
private Boolean defaultEntityBeanOptimisticLocking;
private String defaultMdbInstancePool;
private Boolean defaultMissingMethodPermissionsDenyAccess;
private String defaultResourceAdapterName;
private String defaultSecurityDomain;
private String defaultSfsbCache;
private String defaultSfsbPassivationDisabledCache;
private Long defaultSingletonBeanAccessTimeout;
private String defaultSlsbInstancePool;
private Long defaultStatefulBeanAccessTimeout;
private Boolean disableDefaultEjbPermissions;
private Boolean enableStatistics;
private Boolean inVmRemoteInterfaceInvocationPassByValue;
private Boolean logSystemExceptions;
private Ejb3Resources subresources = new Ejb3Resources();
private TimerService timerService;
private Remote remote;
private Async async;
private Iiop iiop;
public Ejb3() {
this.key = "ejb3";
}
public String getKey() {
return this.key;
}
/**
* Name of the default stateful bean cache, which will be applicable to all clustered stateful EJBs, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-clustered-sfsb-cache")
public String defaultClusteredSfsbCache() {
return this.defaultClusteredSfsbCache;
}
/**
* Name of the default stateful bean cache, which will be applicable to all clustered stateful EJBs, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultClusteredSfsbCache(String value) {
this.defaultClusteredSfsbCache = value;
return (T) this;
}
/**
* The default distinct name that is applied to every EJB deployed on this server
*/
@ModelNodeBinding(detypedName = "default-distinct-name")
public String defaultDistinctName() {
return this.defaultDistinctName;
}
/**
* The default distinct name that is applied to every EJB deployed on this server
*/
@SuppressWarnings("unchecked")
public T defaultDistinctName(String value) {
this.defaultDistinctName = value;
return (T) this;
}
/**
* Name of the default entity bean instance pool, which will be applicable to all entity beans, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-entity-bean-instance-pool")
public String defaultEntityBeanInstancePool() {
return this.defaultEntityBeanInstancePool;
}
/**
* Name of the default entity bean instance pool, which will be applicable to all entity beans, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultEntityBeanInstancePool(String value) {
this.defaultEntityBeanInstancePool = value;
return (T) this;
}
/**
* If set to true entity beans will use optimistic locking by default
*/
@ModelNodeBinding(detypedName = "default-entity-bean-optimistic-locking")
public Boolean defaultEntityBeanOptimisticLocking() {
return this.defaultEntityBeanOptimisticLocking;
}
/**
* If set to true entity beans will use optimistic locking by default
*/
@SuppressWarnings("unchecked")
public T defaultEntityBeanOptimisticLocking(Boolean value) {
this.defaultEntityBeanOptimisticLocking = value;
return (T) this;
}
/**
* Name of the default MDB instance pool, which will be applicable to all MDBs, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-mdb-instance-pool")
public String defaultMdbInstancePool() {
return this.defaultMdbInstancePool;
}
/**
* Name of the default MDB instance pool, which will be applicable to all MDBs, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultMdbInstancePool(String value) {
this.defaultMdbInstancePool = value;
return (T) this;
}
/**
* If this is set to true then methods on an EJB with a security domain specified or with other methods with security metadata will have an implicit @DenyAll unless other security metadata is present
*/
@ModelNodeBinding(detypedName = "default-missing-method-permissions-deny-access")
public Boolean defaultMissingMethodPermissionsDenyAccess() {
return this.defaultMissingMethodPermissionsDenyAccess;
}
/**
* If this is set to true then methods on an EJB with a security domain specified or with other methods with security metadata will have an implicit @DenyAll unless other security metadata is present
*/
@SuppressWarnings("unchecked")
public T defaultMissingMethodPermissionsDenyAccess(Boolean value) {
this.defaultMissingMethodPermissionsDenyAccess = value;
return (T) this;
}
/**
* Name of the default resource adapter name that will be used by MDBs, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-resource-adapter-name")
public String defaultResourceAdapterName() {
return this.defaultResourceAdapterName;
}
/**
* Name of the default resource adapter name that will be used by MDBs, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultResourceAdapterName(String value) {
this.defaultResourceAdapterName = value;
return (T) this;
}
/**
* The default security domain that will be used for EJBs if the bean doesn't explicitly specify one
*/
@ModelNodeBinding(detypedName = "default-security-domain")
public String defaultSecurityDomain() {
return this.defaultSecurityDomain;
}
/**
* The default security domain that will be used for EJBs if the bean doesn't explicitly specify one
*/
@SuppressWarnings("unchecked")
public T defaultSecurityDomain(String value) {
this.defaultSecurityDomain = value;
return (T) this;
}
/**
* Name of the default stateful bean cache, which will be applicable to all stateful EJBs, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-sfsb-cache")
public String defaultSfsbCache() {
return this.defaultSfsbCache;
}
/**
* Name of the default stateful bean cache, which will be applicable to all stateful EJBs, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultSfsbCache(String value) {
this.defaultSfsbCache = value;
return (T) this;
}
/**
* Name of the default stateful bean cache, which will be applicable to all stateful EJBs which have passivation disabled. Each deployment or EJB can optionally override this cache name.
*/
@ModelNodeBinding(detypedName = "default-sfsb-passivation-disabled-cache")
public String defaultSfsbPassivationDisabledCache() {
return this.defaultSfsbPassivationDisabledCache;
}
/**
* Name of the default stateful bean cache, which will be applicable to all stateful EJBs which have passivation disabled. Each deployment or EJB can optionally override this cache name.
*/
@SuppressWarnings("unchecked")
public T defaultSfsbPassivationDisabledCache(String value) {
this.defaultSfsbPassivationDisabledCache = value;
return (T) this;
}
/**
* The default access timeout for singleton beans
*/
@ModelNodeBinding(detypedName = "default-singleton-bean-access-timeout")
public Long defaultSingletonBeanAccessTimeout() {
return this.defaultSingletonBeanAccessTimeout;
}
/**
* The default access timeout for singleton beans
*/
@SuppressWarnings("unchecked")
public T defaultSingletonBeanAccessTimeout(Long value) {
this.defaultSingletonBeanAccessTimeout = value;
return (T) this;
}
/**
* Name of the default stateless bean instance pool, which will be applicable to all stateless EJBs, unless overridden at the deployment or bean level
*/
@ModelNodeBinding(detypedName = "default-slsb-instance-pool")
public String defaultSlsbInstancePool() {
return this.defaultSlsbInstancePool;
}
/**
* Name of the default stateless bean instance pool, which will be applicable to all stateless EJBs, unless overridden at the deployment or bean level
*/
@SuppressWarnings("unchecked")
public T defaultSlsbInstancePool(String value) {
this.defaultSlsbInstancePool = value;
return (T) this;
}
/**
* The default access timeout for stateful beans
*/
@ModelNodeBinding(detypedName = "default-stateful-bean-access-timeout")
public Long defaultStatefulBeanAccessTimeout() {
return this.defaultStatefulBeanAccessTimeout;
}
/**
* The default access timeout for stateful beans
*/
@SuppressWarnings("unchecked")
public T defaultStatefulBeanAccessTimeout(Long value) {
this.defaultStatefulBeanAccessTimeout = value;
return (T) this;
}
/**
* This deprecated attribute has no effect and will be removed in a future release; it may never be set to a "false" value
*/
@ModelNodeBinding(detypedName = "disable-default-ejb-permissions")
public Boolean disableDefaultEjbPermissions() {
return this.disableDefaultEjbPermissions;
}
/**
* This deprecated attribute has no effect and will be removed in a future release; it may never be set to a "false" value
*/
@SuppressWarnings("unchecked")
public T disableDefaultEjbPermissions(Boolean value) {
this.disableDefaultEjbPermissions = value;
return (T) this;
}
/**
* If set to true, enable the collection of invocation statistics.
*/
@ModelNodeBinding(detypedName = "enable-statistics")
public Boolean enableStatistics() {
return this.enableStatistics;
}
/**
* If set to true, enable the collection of invocation statistics.
*/
@SuppressWarnings("unchecked")
public T enableStatistics(Boolean value) {
this.enableStatistics = value;
return (T) this;
}
/**
* If set to false, the parameters to invocations on remote interface of an EJB, will be passed by reference. Else, the parameters will be passed by value.
*/
@ModelNodeBinding(detypedName = "in-vm-remote-interface-invocation-pass-by-value")
public Boolean inVmRemoteInterfaceInvocationPassByValue() {
return this.inVmRemoteInterfaceInvocationPassByValue;
}
/**
* If set to false, the parameters to invocations on remote interface of an EJB, will be passed by reference. Else, the parameters will be passed by value.
*/
@SuppressWarnings("unchecked")
public T inVmRemoteInterfaceInvocationPassByValue(Boolean value) {
this.inVmRemoteInterfaceInvocationPassByValue = value;
return (T) this;
}
/**
* If this is true then all EJB system (not application) exceptions will be logged. The EJB spec mandates this behaviour, however it is not recommended as it will often result in exceptions being logged twice (once by the EJB and once by the calling code)
*/
@ModelNodeBinding(detypedName = "log-system-exceptions")
public Boolean logSystemExceptions() {
return this.logSystemExceptions;
}
/**
* If this is true then all EJB system (not application) exceptions will be logged. The EJB spec mandates this behaviour, however it is not recommended as it will often result in exceptions being logged twice (once by the EJB and once by the calling code)
*/
@SuppressWarnings("unchecked")
public T logSystemExceptions(Boolean value) {
this.logSystemExceptions = value;
return (T) this;
}
public Ejb3Resources subresources() {
return this.subresources;
}
/**
* Add all StrictMaxBeanInstancePool objects to this subresource
* @return this
* @param value List of StrictMaxBeanInstancePool objects.
*/
@SuppressWarnings("unchecked")
public T strictMaxBeanInstancePools(List value) {
this.subresources.strictMaxBeanInstancePools.addAll(value);
return (T) this;
}
/**
* Add the StrictMaxBeanInstancePool object to the list of subresources
* @param value The StrictMaxBeanInstancePool to add
* @return this
*/
@SuppressWarnings("unchecked")
public T strictMaxBeanInstancePool(StrictMaxBeanInstancePool value) {
this.subresources.strictMaxBeanInstancePools.add(value);
return (T) this;
}
/**
* Add all Cache objects to this subresource
* @return this
* @param value List of Cache objects.
*/
@SuppressWarnings("unchecked")
public T caches(List value) {
this.subresources.caches.addAll(value);
return (T) this;
}
/**
* Add the Cache object to the list of subresources
* @param value The Cache to add
* @return this
*/
@SuppressWarnings("unchecked")
public T cache(Cache value) {
this.subresources.caches.add(value);
return (T) this;
}
/**
* Add all RemotingProfile objects to this subresource
* @return this
* @param value List of RemotingProfile objects.
*/
@SuppressWarnings("unchecked")
public T remotingProfiles(List value) {
this.subresources.remotingProfiles.addAll(value);
return (T) this;
}
/**
* Add the RemotingProfile object to the list of subresources
* @param value The RemotingProfile to add
* @return this
*/
@SuppressWarnings("unchecked")
public T remotingProfile(RemotingProfile value) {
this.subresources.remotingProfiles.add(value);
return (T) this;
}
/**
* Add all ClusterPassivationStore objects to this subresource
* @return this
* @param value List of ClusterPassivationStore objects.
*/
@SuppressWarnings("unchecked")
public T clusterPassivationStores(List value) {
this.subresources.clusterPassivationStores.addAll(value);
return (T) this;
}
/**
* Add the ClusterPassivationStore object to the list of subresources
* @param value The ClusterPassivationStore to add
* @return this
*/
@SuppressWarnings("unchecked")
public T clusterPassivationStore(ClusterPassivationStore value) {
this.subresources.clusterPassivationStores.add(value);
return (T) this;
}
/**
* Add all PassivationStore objects to this subresource
* @return this
* @param value List of PassivationStore objects.
*/
@SuppressWarnings("unchecked")
public T passivationStores(List value) {
this.subresources.passivationStores.addAll(value);
return (T) this;
}
/**
* Add the PassivationStore object to the list of subresources
* @param value The PassivationStore to add
* @return this
*/
@SuppressWarnings("unchecked")
public T passivationStore(PassivationStore value) {
this.subresources.passivationStores.add(value);
return (T) this;
}
/**
* Add all MdbDeliveryGroup objects to this subresource
* @return this
* @param value List of MdbDeliveryGroup objects.
*/
@SuppressWarnings("unchecked")
public T mdbDeliveryGroups(List value) {
this.subresources.mdbDeliveryGroups.addAll(value);
return (T) this;
}
/**
* Add the MdbDeliveryGroup object to the list of subresources
* @param value The MdbDeliveryGroup to add
* @return this
*/
@SuppressWarnings("unchecked")
public T mdbDeliveryGroup(MdbDeliveryGroup value) {
this.subresources.mdbDeliveryGroups.add(value);
return (T) this;
}
/**
* Add all ThreadPool objects to this subresource
* @return this
* @param value List of ThreadPool objects.
*/
@SuppressWarnings("unchecked")
public T threadPools(List value) {
this.subresources.threadPools.addAll(value);
return (T) this;
}
/**
* Add the ThreadPool object to the list of subresources
* @param value The ThreadPool to add
* @return this
*/
@SuppressWarnings("unchecked")
public T threadPool(ThreadPool value) {
this.subresources.threadPools.add(value);
return (T) this;
}
/**
* Add all FilePassivationStore objects to this subresource
* @return this
* @param value List of FilePassivationStore objects.
*/
@SuppressWarnings("unchecked")
public T filePassivationStores(List value) {
this.subresources.filePassivationStores.addAll(value);
return (T) this;
}
/**
* Add the FilePassivationStore object to the list of subresources
* @param value The FilePassivationStore to add
* @return this
*/
@SuppressWarnings("unchecked")
public T filePassivationStore(FilePassivationStore value) {
this.subresources.filePassivationStores.add(value);
return (T) this;
}
/**
* Child mutators for Ejb3
*/
public class Ejb3Resources {
/**
* A bean instance pool with a strict upper limit
*/
private List strictMaxBeanInstancePools = new java.util.ArrayList<>();
/**
* A SFSB cache
*/
private List caches = new java.util.ArrayList<>();
/**
* A remoting profile
*/
private List remotingProfiles = new java.util.ArrayList<>();
/**
* A clustered passivation store
*/
private List clusterPassivationStores = new java.util.ArrayList<>();
/**
* A passivation store
*/
private List passivationStores = new java.util.ArrayList<>();
/**
* Delivery group to manage delivery for mdbs
*/
private List mdbDeliveryGroups = new java.util.ArrayList<>();
/**
* A thread pool executor with an unbounded queue. Such a thread pool has a core size and a queue with no upper bound. When a task is submitted, if the number of running threads is less than the core size, a new thread is created. Otherwise, the task is placed in queue. If too many tasks are allowed to be submitted to this type of executor, an out of memory condition may occur.
*/
private List threadPools = new java.util.ArrayList<>();
/**
* A file system based passivation store
*/
private List filePassivationStores = new java.util.ArrayList<>();
/**
* Get the list of StrictMaxBeanInstancePool resources
* @return the list of resources
*/
@Subresource
public List strictMaxBeanInstancePools() {
return this.strictMaxBeanInstancePools;
}
/**
* Get the list of Cache resources
* @return the list of resources
*/
@Subresource
public List caches() {
return this.caches;
}
/**
* Get the list of RemotingProfile resources
* @return the list of resources
*/
@Subresource
public List remotingProfiles() {
return this.remotingProfiles;
}
/**
* Get the list of ClusterPassivationStore resources
* @return the list of resources
*/
@Subresource
public List clusterPassivationStores() {
return this.clusterPassivationStores;
}
/**
* Get the list of PassivationStore resources
* @return the list of resources
*/
@Subresource
public List passivationStores() {
return this.passivationStores;
}
/**
* Get the list of MdbDeliveryGroup resources
* @return the list of resources
*/
@Subresource
public List mdbDeliveryGroups() {
return this.mdbDeliveryGroups;
}
/**
* Get the list of ThreadPool resources
* @return the list of resources
*/
@Subresource
public List threadPools() {
return this.threadPools;
}
/**
* Get the list of FilePassivationStore resources
* @return the list of resources
*/
@Subresource
public List filePassivationStores() {
return this.filePassivationStores;
}
}
/**
* The EJB timer service
*/
@Subresource
public TimerService timerService() {
return this.timerService;
}
/**
* The EJB timer service
*/
@SuppressWarnings("unchecked")
public T timerService(TimerService value) {
this.timerService = value;
return (T) this;
}
/**
* The EJB3 Remote Service
*/
@Subresource
public Remote remote() {
return this.remote;
}
/**
* The EJB3 Remote Service
*/
@SuppressWarnings("unchecked")
public T remote(Remote value) {
this.remote = value;
return (T) this;
}
/**
* The EJB3 Asynchronous Invocation Service
*/
@Subresource
public Async async() {
return this.async;
}
/**
* The EJB3 Asynchronous Invocation Service
*/
@SuppressWarnings("unchecked")
public T async(Async value) {
this.async = value;
return (T) this;
}
/**
* The IIOP service
*/
@Subresource
public Iiop iiop() {
return this.iiop;
}
/**
* The IIOP service
*/
@SuppressWarnings("unchecked")
public T iiop(Iiop value) {
this.iiop = value;
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy