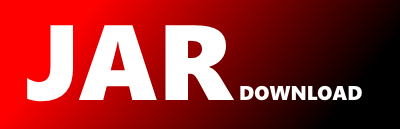
org.wildfly.galleon.plugin.WfInstallPlugin Maven / Gradle / Ivy
/*
* Copyright 2016-2020 Red Hat, Inc. and/or its affiliates
* and other contributors as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.wildfly.galleon.plugin;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URL;
import java.net.URLClassLoader;
import java.nio.file.FileAlreadyExistsException;
import java.nio.file.FileSystem;
import java.nio.file.FileSystems;
import java.nio.file.FileVisitOption;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.StandardCopyOption;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import javax.xml.transform.stream.StreamSource;
import nu.xom.Elements;
import org.jboss.galleon.Errors;
import org.jboss.galleon.MessageWriter;
import org.jboss.galleon.ProvisioningException;
import org.jboss.galleon.ProvisioningManager;
import org.jboss.galleon.ProvisioningOption;
import org.jboss.galleon.config.ConfigId;
import org.jboss.galleon.config.ConfigModel;
import org.jboss.galleon.config.FeaturePackConfig;
import org.jboss.galleon.config.ProvisioningConfig;
import org.jboss.galleon.diff.FsDiff;
import org.jboss.galleon.layout.ProvisioningLayoutFactory;
import org.jboss.galleon.plugin.InstallPlugin;
import org.jboss.galleon.plugin.ProvisioningPluginWithOptions;
import org.jboss.galleon.progresstracking.ProgressCallback;
import org.jboss.galleon.progresstracking.ProgressTracker;
import org.jboss.galleon.runtime.FeaturePackRuntime;
import org.jboss.galleon.runtime.PackageRuntime;
import org.jboss.galleon.runtime.ProvisioningRuntime;
import org.jboss.galleon.universe.FeaturePackLocation.FPID;
import org.jboss.galleon.universe.FeaturePackLocation.ProducerSpec;
import org.jboss.galleon.universe.maven.MavenArtifact;
import org.jboss.galleon.universe.maven.MavenUniverseException;
import org.jboss.galleon.universe.maven.repo.MavenRepoManager;
import org.jboss.galleon.util.IoUtils;
import org.jboss.galleon.util.CollectionUtils;
import org.jboss.galleon.util.ZipUtils;
import org.wildfly.galleon.plugin.config.AssembleShadedArtifact;
import org.wildfly.galleon.plugin.config.CopyArtifact;
import org.wildfly.galleon.plugin.config.CopyPath;
import org.wildfly.galleon.plugin.config.DeletePath;
import org.wildfly.galleon.plugin.config.ExampleFpConfigs;
import org.wildfly.galleon.plugin.config.LineEndingsTask;
import org.wildfly.galleon.plugin.config.XslTransform;
/**
* WildFly install plugin. Handles all WildFly specifics that occur during provisioning.
* @author Alexey Loubyansky
*/
public class WfInstallPlugin extends ProvisioningPluginWithOptions implements InstallPlugin {
// If tooling used for provisioning has wildfly channels setup, the artifacts must be resolved from the channel
public static final String REQUIRES_CHANNEL_FOR_ARTIFACT_RESOLUTION_PROPERTY = "org.wildfly.plugins.galleon.all.artifact.requires.channel.resolution";
private static final String TRACK_MODULES_BUILD = "JBMODULES";
private static final String TRACK_COPY_CONFIGS = "JBCOPYCONFIGS";
private static final String TRACK_ARTIFACTS_RESOLVE = "JB_ARTIFACTS_RESOLVE";
private Optional artifactRecorder;
public interface ArtifactResolver {
void resolve(MavenArtifact artifact) throws ProvisioningException;
}
private static final String CONFIG_GEN_METHOD = "generate";
private static final String CONFIG_GEN_GA = "org.wildfly.galleon-plugins:wildfly-config-gen";
private static final String GALLEON_PLUGINS_GA = "org.wildfly.galleon-plugins:wildfly-galleon-plugins";
private static final String CONFIG_GEN_CLASS = "org.wildfly.galleon.plugin.config.generator.WfConfigGenerator";
private static final String CLI_SCRIPT_RUNNER_CLASS = "org.wildfly.galleon.plugin.config.generator.CliScriptRunner";
private static final String CLI_SCRIPT_RUNNER_METHOD = "runCliScript";
private static final String JBOSS_MODULES_GA = "org.jboss.modules:jboss-modules";
private static final String MAVEN_REPO_LOCAL = "maven.repo.local";
private static final String WILDFLY_CLI_GA = "org.wildfly.core:wildfly-cli";
private static final String WILDFLY_LAUNCHER_GA = "org.wildfly.core:wildfly-launcher";
private static final ProvisioningOption OPTION_MVN_DIST = ProvisioningOption.builder("jboss-maven-dist")
.setBooleanValueSet()
.build();
public static final ProvisioningOption OPTION_DUMP_CONFIG_SCRIPTS = ProvisioningOption.builder("jboss-dump-config-scripts").setPersistent(false).build();
private static final ProvisioningOption OPTION_FORK_EMBEDDED = ProvisioningOption.builder("jboss-fork-embedded")
.setBooleanValueSet()
.build();
/**
* If present, indicates whether the existing System Properties will be reset to the default set provided by
* ForkedEmbeddedUtil.RESETTABLE_EMBEDDED_SYS_PROPERTIES. The format of this configuration is a comma separated list of
* system properties to add or remove from this default Set.
*
* If the property starts with '-', it means the property will be removed from the set, otherwise, the property will be added.
* Values are added or removed from the default Set in the same order as they have been specified in this configuration option.
*
* @see @see org.wildfly.galleon.plugin.server.ForkedEmbeddedUtil
*/
private static final ProvisioningOption OPTION_RESET_EMBEDDED_SYSTEM_PROPERTIES = ProvisioningOption.builder("jboss-reset-embedded-system-properties")
.build();
private static final ProvisioningOption OPTION_MVN_REPO = ProvisioningOption.builder("jboss-maven-repo")
.setPersistent(false)
.build();
private static final ProvisioningOption OPTION_OVERRIDDEN_ARTIFACTS = ProvisioningOption.builder("jboss-overridden-artifacts").setPersistent(true).build();
private static final ProvisioningOption OPTION_BULK_RESOLVE_ARTIFACTS = ProvisioningOption.builder("jboss-bulk-resolve-artifacts").setBooleanValueSet().build();
private static final ProvisioningOption OPTION_RECORD_ARTIFACTS = ProvisioningOption.builder("jboss-resolved-artifacts-cache")
.setDefaultValue(".installation" + File.separator + ".cache")
.build();
private ProvisioningRuntime runtime;
MessageWriter log;
private Map mergedArtifactVersions = new HashMap<>();
private final Map overriddenArtifactVersions = new HashMap<>();
private Map> fpArtifactVersions = new HashMap<>();
private Map> fpTasksProps = Collections.emptyMap();
private Map mergedTaskProps = new HashMap<>();
private PropertyResolver mergedTaskPropsResolver;
private boolean thinServer;
private Set schemaGroups = Collections.emptySet();
private List finalizingTasks = Collections.emptyList();
private List finalizingTasksPkgs = Collections.emptyList();
private DocumentBuilderFactory docBuilderFactory;
private TransformerFactory xsltFactory;
private Map xslTransformers = Collections.emptyMap();
private Map exampleConfigs = Collections.emptyMap();
private ProgressTracker pkgProgressTracker;
private MavenRepoManager maven;
private Map jbossModules = new LinkedHashMap<>();
private Path generatedMavenRepo;
private AbstractArtifactInstaller artifactInstaller;
private ArtifactResolver artifactResolver;
private boolean channelArtifactResolution;
private boolean bulkResolveArtifacts;
private final Map artifactCache = new HashMap<>();
private final Map moduleTemplateCache = new HashMap<>();
private final Map resolvedVersionsProperties = new HashMap<>();
private Map channelResolutionModes = new LinkedHashMap<>();
private Map gaToProducer = new HashMap<>();
private final Map shadedPackages = new HashMap<>();
@Override
protected List initPluginOptions() {
return Arrays.asList(OPTION_MVN_DIST, OPTION_DUMP_CONFIG_SCRIPTS,
OPTION_FORK_EMBEDDED, OPTION_MVN_REPO,
OPTION_RESET_EMBEDDED_SYSTEM_PROPERTIES,
OPTION_OVERRIDDEN_ARTIFACTS, OPTION_BULK_RESOLVE_ARTIFACTS,
OPTION_RECORD_ARTIFACTS);
}
public ProvisioningRuntime getRuntime() {
return runtime;
}
private boolean isThinServer() throws ProvisioningException {
return getBooleanOption(OPTION_MVN_DIST);
}
private Path getGeneratedMavenRepo() throws ProvisioningException {
if (!runtime.isOptionSet(OPTION_MVN_REPO)) {
return null;
}
final String value = runtime.getOptionValue(OPTION_MVN_REPO);
return value == null ? null : Paths.get(value);
}
private Map getOverriddenArtifacts() throws ProvisioningException {
if (!runtime.isOptionSet(OPTION_OVERRIDDEN_ARTIFACTS)) {
return Collections.emptyMap();
}
if (channelArtifactResolution) {
throw new ProvisioningException("Option " + OPTION_OVERRIDDEN_ARTIFACTS + " can't be used when channels are enabled.");
}
final String value = runtime.getOptionValue(OPTION_OVERRIDDEN_ARTIFACTS);
return value == null ? Collections.emptyMap() : Utils.toArtifactsMap(value);
}
private boolean isBulkResolveArtifacts() throws ProvisioningException {
return getBooleanOption(OPTION_BULK_RESOLVE_ARTIFACTS);
}
private boolean isForkEmbedded(ProvisioningRuntime runtime) throws ProvisioningException {
return getBooleanOption(OPTION_FORK_EMBEDDED);
}
private String isResetEmbeddedSystemProperties() throws ProvisioningException {
if (!runtime.isOptionSet(OPTION_RESET_EMBEDDED_SYSTEM_PROPERTIES)) {
return null;
}
final String value = runtime.getOptionValue(OPTION_RESET_EMBEDDED_SYSTEM_PROPERTIES);
return value == null ? "" : value;
}
private boolean getBooleanOption(ProvisioningOption option) throws ProvisioningException {
if (!runtime.isOptionSet(option)) {
return false;
}
final String value = runtime.getOptionValue(option);
return value == null ? true : Boolean.parseBoolean(value);
}
@Override
public void preInstall(ProvisioningRuntime runtime) throws ProvisioningException {
final FsDiff fsDiff = runtime.getFsDiff();
if(fsDiff == null) {
return;
}
final String runningMode = fsDiff.getEntry("standalone/tmp/startup-marker") != null ? WfConstants.STANDALONE
: fsDiff.getEntry("domain/tmp/startup-marker") != null ? WfConstants.DOMAIN : null;
if (runningMode != null) {
throw new ProvisioningException("The server appears to be running (" + runningMode + " mode).");
}
}
/* (non-Javadoc)
* @see org.jboss.galleon.util.plugin.ProvisioningPlugin#execute()
*/
@Override
public void postInstall(ProvisioningRuntime runtime) throws ProvisioningException {
final long startTime = runtime.isLogTime() ? System.nanoTime() : -1;
this.runtime = runtime;
log = runtime.getMessageWriter();
log.verbose("WildFly Galleon Installation Plugin");
if (runtime.isOptionSet(OPTION_RECORD_ARTIFACTS)) {
final String pathValue = runtime.getOptionValue(OPTION_RECORD_ARTIFACTS);
if (pathValue != null && !pathValue.isEmpty()) {
try {
log.verbose("Starting artifact log");
artifactRecorder = Optional.of(new ArtifactRecorder(runtime.getStagedDir(), Path.of(pathValue)));
} catch (IOException e) {
throw new ProvisioningException("Unable to create artifact.log", e);
}
}
} else {
artifactRecorder = Optional.empty();
}
this.bulkResolveArtifacts = isBulkResolveArtifacts();
thinServer = isThinServer();
generatedMavenRepo = getGeneratedMavenRepo();
if (generatedMavenRepo != null) {
IoUtils.recursiveDelete(generatedMavenRepo);
}
maven = (MavenRepoManager) runtime.getArtifactResolver(MavenRepoManager.REPOSITORY_ID);
// The Channel resolution depends on the tool in use.
// Generic Galleon provisioning doesn't support it.
try {
Class> clazz = Class.forName("org.wildfly.channel.spi.ChannelResolvable");
channelArtifactResolution = clazz.isAssignableFrom(maven.getClass());
} catch(ClassNotFoundException ex) {
log.verbose("Channel not present in classpath.");
}
log.verbose("Channel artifact resolution enabled=" + channelArtifactResolution);
// Overridden artifacts
overriddenArtifactVersions.putAll(getOverriddenArtifacts());
for(FeaturePackRuntime fp : runtime.getFeaturePacks()) {
final Path wfRes = fp.getResource(WfConstants.WILDFLY);
if(!Files.exists(wfRes)) {
continue;
}
final Path artifactProps = wfRes.resolve(WfConstants.ARTIFACT_VERSIONS_PROPS);
if(Files.exists(artifactProps)) {
final Map versionProps = Utils.readProperties(artifactProps);
for (Entry entry : overriddenArtifactVersions.entrySet()) {
if (versionProps.containsKey(entry.getKey())) {
versionProps.put(entry.getKey(), entry.getValue());
}
}
fpArtifactVersions.put(fp.getFPID().getProducer(), versionProps);
// Handle artifacts that are directly resolved from the plugin
// org.wildfly.core:wildfly-launcher
// org.jboss.modules:jboss-modules
// org.wildfly.core:wildfly-cli
// org.wildfly.galleon-plugins:wildfly-config-gen
// org.wildfly.galleon-plugins:wildfly-galleon-plugins
if (versionProps.containsKey(CONFIG_GEN_GA)) {
gaToProducer.put(CONFIG_GEN_GA, fp.getFPID().getProducer());
}
if (versionProps.containsKey(GALLEON_PLUGINS_GA)) {
gaToProducer.put(GALLEON_PLUGINS_GA, fp.getFPID().getProducer());
}
if (versionProps.containsKey(WILDFLY_CLI_GA)) {
gaToProducer.put(WILDFLY_CLI_GA, fp.getFPID().getProducer());
}
if (versionProps.containsKey(WILDFLY_LAUNCHER_GA)) {
gaToProducer.put(WILDFLY_LAUNCHER_GA, fp.getFPID().getProducer());
}
if (versionProps.containsKey(JBOSS_MODULES_GA)) {
gaToProducer.put(JBOSS_MODULES_GA, fp.getFPID().getProducer());
}
mergedArtifactVersions.putAll(versionProps);
}
final Path tasksPropsPath = wfRes.resolve(WfConstants.WILDFLY_TASKS_PROPS);
if(Files.exists(tasksPropsPath)) {
final Map fpProps = Utils.readProperties(tasksPropsPath);
fpTasksProps = CollectionUtils.put(fpTasksProps, fp.getFPID().getProducer(), fpProps);
mergedTaskProps.putAll(fpProps);
}
final Path channelsPropsPath = wfRes.resolve(WfConstants.WILDFLY_CHANNEL_PROPS);
if(Files.exists(channelsPropsPath)) {
final Map channelProps = Utils.readProperties(channelsPropsPath);
String mode = channelProps.get(WfConstants.WILDFLY_CHANNEL_RESOLUTION_PROP);
if (mode != null) {
channelResolutionModes = CollectionUtils.put(channelResolutionModes, fp.getFPID().getProducer(), WildFlyChannelResolutionMode.valueOf(mode));
}
}
if(fp.containsPackage(WfConstants.DOCS_SCHEMA)) {
final Path schemaGroupsTxt = fp.getPackage(WfConstants.DOCS_SCHEMA).getResource(
WfConstants.PM, WfConstants.WILDFLY, WfConstants.SCHEMA_GROUPS_TXT);
try(BufferedReader reader = Files.newBufferedReader(schemaGroupsTxt)) {
String line = reader.readLine();
while(line != null) {
schemaGroups = CollectionUtils.add(schemaGroups, line);
line = reader.readLine();
}
} catch (IOException e) {
throw new ProvisioningException(Errors.readFile(schemaGroupsTxt), e);
}
}
}
// Check that all overridden artifacts are actually known.
for (String key : overriddenArtifactVersions.keySet()) {
if (!mergedArtifactVersions.containsKey(key)) {
throw new ProvisioningException("Overridden artifacts " + key + " is not found in the set of known server artifacts");
}
}
mergedArtifactVersions.putAll(overriddenArtifactVersions);
mergedTaskPropsResolver = new MapPropertyResolver(mergedTaskProps);
// We must create resolver and installer at this point, prior to process the packges.
// The CopyArtifact tasks could need the resolver and installer we are instantiating there.
artifactResolver = this::resolveMaven;
artifactInstaller = new SimpleArtifactInstaller(artifactResolver, generatedMavenRepo, artifactRecorder);
// Resolution of provisioning artifacts that we would need in the generated licenses.
MavenArtifact configGen = Utils.toArtifactCoords(mergedArtifactVersions, CONFIG_GEN_GA,
false, channelArtifactResolution, requireChannel(gaToProducer.get(CONFIG_GEN_GA)));
artifactResolver.resolve(configGen);
MavenArtifact plugin = Utils.toArtifactCoords(mergedArtifactVersions, GALLEON_PLUGINS_GA,
false, channelArtifactResolution, requireChannel(gaToProducer.get(GALLEON_PLUGINS_GA)));
artifactResolver.resolve(plugin);
final ProvisioningLayoutFactory layoutFactory = runtime.getLayout().getFactory();
pkgProgressTracker = layoutFactory.getProgressTracker(ProvisioningLayoutFactory.TRACK_PACKAGES);
long pkgsTotal = 0;
for(FeaturePackRuntime fp : runtime.getFeaturePacks()) {
pkgsTotal += fp.getPackageNames().size();
}
pkgProgressTracker.starting(pkgsTotal);
// Must first retrieve the shaded that could be required by other packages
for(FeaturePackRuntime fp : runtime.getFeaturePacks()) {
processShaded(fp);
}
for(FeaturePackRuntime fp : runtime.getFeaturePacks()) {
processPackages(fp);
}
pkgProgressTracker.complete();
if (!jbossModules.isEmpty()) {
if (bulkResolveArtifacts) {
log.verbose("Preloading artifacts");
final ProgressTracker artifactTracker = layoutFactory.getProgressTracker(TRACK_ARTIFACTS_RESOLVE);
populateArtifactCache();
artifactTracker.starting(artifactCache.size());
resolveArtifactsInCache(artifactTracker);
artifactTracker.complete();
log.verbose("Finished preloading artifacts");
}
final ProgressTracker modulesTracker = layoutFactory.getProgressTracker(TRACK_MODULES_BUILD);
modulesTracker.starting(jbossModules.size());
for (Map.Entry entry : jbossModules.entrySet()) {
final PackageRuntime pkg = entry.getValue();
modulesTracker.processing(pkg);
try {
processModuleTemplate(pkg, entry.getKey());
} catch (IOException e) {
throw new ProvisioningException("Failed to process JBoss module XML template for feature-pack "
+ pkg.getFeaturePackRuntime().getFPID() + " package " + pkg.getName(), e);
}
modulesTracker.processed(pkg);
}
modulesTracker.complete();
}
final Path layersConf = runtime.getStagedDir().resolve(WfConstants.MODULES).resolve(WfConstants.LAYERS_CONF);
if (Files.exists(layersConf)) {
mergeLayerConfs(runtime);
}
generateConfigs(runtime);
// If the dir doesn't exist, no configuration has been generated, no need to execute CLI scripts.
if (Files.exists(runtime.getStagedDir())) {
for (FeaturePackRuntime fp : runtime.getFeaturePacks()) {
final Path finalizeCli = fp.getResource(WfConstants.WILDFLY, WfConstants.SCRIPTS, "finalize.cli");
if (Files.exists(finalizeCli)) {
final URL[] cp = new URL[2];
try {
MavenArtifact artifact = Utils.toArtifactCoords(mergedArtifactVersions, CONFIG_GEN_GA,
false, channelArtifactResolution, requireChannel(gaToProducer.get(CONFIG_GEN_GA)));
artifactResolver.resolve(artifact);
cp[0] = artifact.getPath().toUri().toURL();
artifact = Utils.toArtifactCoords(mergedArtifactVersions, WILDFLY_LAUNCHER_GA,
false, channelArtifactResolution, requireChannel(gaToProducer.get(WILDFLY_LAUNCHER_GA)));
artifactResolver.resolve(artifact);
cp[1] = artifact.getPath().toUri().toURL();
} catch (IOException e) {
throw new ProvisioningException("Failed to init classpath to run CLI finalize script for " + runtime.getStagedDir(), e);
}
final ClassLoader originalCl = Thread.currentThread().getContextClassLoader();
final URLClassLoader cliScriptCl = new URLClassLoader(cp, originalCl);
Path script;
try {
try {
byte[] content = Files.readAllBytes(finalizeCli);
Path tmpDir = runtime.getTmpPath();
if (!Files.exists(tmpDir)) {
Files.createDirectory(tmpDir);
}
script = tmpDir.resolve(finalizeCli.getFileName().toString());
Files.write(script, content);
} catch (IOException ex) {
throw new ProvisioningException(ex.getLocalizedMessage(), ex);
}
Thread.currentThread().setContextClassLoader(cliScriptCl);
try {
final Class> cliScriptRunnerCls = cliScriptCl.loadClass(CLI_SCRIPT_RUNNER_CLASS);
final Method m = cliScriptRunnerCls.getMethod(CLI_SCRIPT_RUNNER_METHOD, Path.class, Path.class, MessageWriter.class);
m.invoke(null, runtime.getStagedDir(), script, log);
} catch (Throwable e) {
throw new ProvisioningException("Failed to initialize CLI script runner " + CLI_SCRIPT_RUNNER_CLASS, e);
}
} finally {
Thread.currentThread().setContextClassLoader(originalCl);
try {
cliScriptCl.close();
} catch (IOException e) {
}
}
}
}
}
if(!finalizingTasks.isEmpty()) {
for(int i = 0; i < finalizingTasks.size(); ++i) {
finalizingTasks.get(i).execute(this, finalizingTasksPkgs.get(i));
}
}
if(!exampleConfigs.isEmpty()) {
provisionExampleConfigs();
}
if (artifactRecorder.isPresent()) {
try {
artifactRecorder.get().writeCacheManifest();
} catch (IOException e) {
throw new ProvisioningException("Unable to record provisioned artifacts", e);
}
}
if (startTime > 0) {
log.print(Errors.tookTime("Overall WildFly Galleon Plugin", startTime));
}
}
private void populateArtifactCache() throws ProvisioningException {
for (Entry entry : jbossModules.entrySet()) {
final PackageRuntime pkg = entry.getValue();
try {
findArtifacts(pkg, entry.getKey());
} catch (IOException e) {
throw new ProvisioningException("Failed to process JBoss module XML template for feature-pack "
+ pkg.getFeaturePackRuntime().getFPID() + " package " + pkg.getName(), e);
}
}
}
private void findArtifacts(PackageRuntime pkg, Path moduleXmlRelativePath) throws ProvisioningException, IOException {
final Path moduleTemplateFile = pkg.getResource(WfConstants.PM, WfConstants.WILDFLY, WfConstants.MODULE).resolve(moduleXmlRelativePath);
final Path targetPath = runtime.getStagedDir().resolve(moduleXmlRelativePath.toString());
final Map versionProps = fpArtifactVersions.get(pkg.getFeaturePackRuntime().getFPID().getProducer());
ModuleTemplate moduleTemplate = new ModuleTemplate(pkg, moduleTemplateFile, targetPath);
moduleTemplateCache.put(moduleTemplateFile, moduleTemplate);
if (!moduleTemplate.isModule()) {
return;
}
final Elements artifacts = moduleTemplate.getArtifacts();
if (artifacts == null) {
return;
}
final int artifactCount = artifacts.size();
for (int i = 0; i < artifactCount; i++) {
final AbstractModuleTemplateProcessor.ModuleArtifact moduleArtifact = new AbstractModuleTemplateProcessor.ModuleArtifact(moduleTemplate,
artifacts.get(i), versionProps, log, artifactInstaller, channelArtifactResolution,
requireChannel(pkg.getFeaturePackRuntime().getFPID().getProducer()));
final MavenArtifact mavenArtifact = moduleArtifact.getUnresolvedArtifact();
if (mavenArtifact != null) {
final MavenArtifact key = new MavenArtifact();
key.setGroupId(mavenArtifact.getGroupId());
key.setArtifactId(mavenArtifact.getArtifactId());
key.setExtension(mavenArtifact.getExtension());
key.setClassifier(mavenArtifact.getClassifier());
key.setVersion(mavenArtifact.getVersion());
key.setVersionRange(mavenArtifact.getVersionRange());
artifactCache.put(key, mavenArtifact);
}
}
}
private void resolveArtifactsInCache(ProgressTracker tracker) throws ProvisioningException {
try {
maven.resolveAll(addListener(artifactCache.values(), tracker));
} catch (MavenUniverseException e) {
throw new ProvisioningException("Failed to resolve artifact", e);
}
}
private Collection addListener(Collection values, ProgressTracker tracker) {
return values.stream().map((a)->new MonitorableArtifact(a, tracker)).collect(Collectors.toList());
}
private void setupLayerDirectory(Path layersConf, Path layersDir) throws ProvisioningException {
log.verbose("Creating layers directories if needed.");
try (BufferedReader reader = Files.newBufferedReader(layersConf)) {
Properties props = new Properties();
props.load(reader);
String layersProp = props.getProperty(WfConstants.LAYERS);
if (layersProp == null || (layersProp = layersProp.trim()).length() == 0) {
return;
}
final String[] layerNames = layersProp.split(",");
for (String layerName : layerNames) {
log.verbose("Found layer %s", layerName);
Path layerDir = layersDir.resolve(layerName);
if (!Files.exists(layerDir)) {
log.verbose("Creating directory %s", layerDir);
Files.createDirectories(layerDir);
}
}
} catch (IOException ex) {
throw new ProvisioningException("Failed to setup layers directory in " + layersDir, ex);
}
}
private void mergeLayerConfs(ProvisioningRuntime runtime) throws ProvisioningException {
final List layersConfs = Utils.collectLayersConf(runtime.getLayout());
// The list contains all layer confs, even the one that will be not provisioned.
// create directories for all of them.
for (Path p : layersConfs) {
setupLayerDirectory(p, runtime.getStagedDir().resolve(WfConstants.MODULES).
resolve(WfConstants.SYSTEM).resolve(WfConstants.LAYERS));
}
if(layersConfs.size() < 2) {
return;
}
Utils.mergeLayersConfs(layersConfs, runtime.getStagedDir());
}
private void provisionExampleConfigs() throws ProvisioningException {
final Path examplesTmp = runtime.getTmpPath("example-configs");
final ProvisioningLayoutFactory factory = runtime.getLayout().getFactory();
final ProgressTracker> examplesTracker = factory.getProgressTracker("JBEXTRACONFIGS");
final List trackedPhases = new ArrayList<>(List.of(ProvisioningLayoutFactory.TRACK_LAYOUT_BUILD, ProvisioningLayoutFactory.TRACK_PACKAGES,
TRACK_MODULES_BUILD, ProvisioningLayoutFactory.TRACK_CONFIGS));
if (isBulkResolveArtifacts()) {
trackedPhases.add(2, TRACK_ARTIFACTS_RESOLVE);
}
final ProgressCallback
© 2015 - 2024 Weber Informatics LLC | Privacy Policy