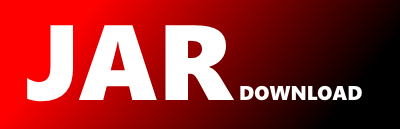
org.wildfly.plugin.tests.AbstractWildFlyServerMojoTest Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.wildfly.plugin.tests;
import static org.junit.Assert.fail;
import java.io.IOException;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.attribute.BasicFileAttributes;
import javax.inject.Inject;
import org.jboss.as.controller.client.ModelControllerClient;
import org.jboss.as.controller.client.helpers.Operations;
import org.jboss.dmr.ModelNode;
import org.junit.runner.RunWith;
import org.wildfly.plugin.tests.runner.WildFlyTestRunner;
/**
* @author James R. Perkins
*/
@RunWith(WildFlyTestRunner.class)
public abstract class AbstractWildFlyServerMojoTest extends AbstractWildFlyMojoTest {
@Inject
protected ModelControllerClient client;
protected ModelNode executeOperation(final ModelNode op) throws IOException {
final ModelNode result = client.execute(op);
if (!Operations.isSuccessfulOutcome(result)) {
fail(Operations.getFailureDescription(result).asString());
}
return result;
}
protected static void deleteRecursively(final Path path) throws IOException {
if (Files.exists(path)) {
if (Files.isDirectory(path)) {
Files.walkFileTree(path, new SimpleFileVisitor() {
@Override
public FileVisitResult visitFile(final Path file, final BasicFileAttributes attrs) throws IOException {
Files.deleteIfExists(file);
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult postVisitDirectory(final Path dir, final IOException exc) throws IOException {
Files.deleteIfExists(dir);
return FileVisitResult.CONTINUE;
}
});
} else {
Files.deleteIfExists(path);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy