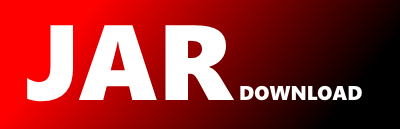
org.wildfly.swarm.config.elytron.ConfigurableHTTPServerMechanismFactory Maven / Gradle / Ivy
The newest version!
package org.wildfly.swarm.config.elytron;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.List;
import java.util.Arrays;
import java.util.stream.Collectors;
import java.util.Map;
/**
* A HTTP server factory definition that wraps another HTTP server factory and
* applies the specified configuration and filtering.
*/
@Address("/subsystem=elytron/configurable-http-server-mechanism-factory=*")
@ResourceType("configurable-http-server-mechanism-factory")
public class ConfigurableHTTPServerMechanismFactory>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("The HTTP mechanisms available from this factory instance.")
private List availableMechanisms;
@AttributeDocumentation("Filtering to be applied to enable / disable mechanisms based on the name.")
private List filters;
@AttributeDocumentation("The http server factory to be wrapped.")
private String httpServerMechanismFactory;
@AttributeDocumentation("Custom properties to be passed in to the http server factory calls.")
private Map properties;
public ConfigurableHTTPServerMechanismFactory(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* The HTTP mechanisms available from this factory instance.
*/
@ModelNodeBinding(detypedName = "available-mechanisms")
public List availableMechanisms() {
return this.availableMechanisms;
}
/**
* The HTTP mechanisms available from this factory instance.
*/
@SuppressWarnings("unchecked")
public T availableMechanisms(java.util.List value) {
Object oldValue = this.availableMechanisms;
this.availableMechanisms = value;
if (this.pcs != null)
this.pcs.firePropertyChange("availableMechanisms", oldValue, value);
return (T) this;
}
/**
* The HTTP mechanisms available from this factory instance.
*/
@SuppressWarnings("unchecked")
public T availableMechanism(String value) {
if (this.availableMechanisms == null) {
this.availableMechanisms = new java.util.ArrayList<>();
}
this.availableMechanisms.add(value);
return (T) this;
}
/**
* The HTTP mechanisms available from this factory instance.
*/
@SuppressWarnings("unchecked")
public T availableMechanisms(String... args) {
availableMechanisms(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* Filtering to be applied to enable / disable mechanisms based on the name.
*/
@ModelNodeBinding(detypedName = "filters")
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy