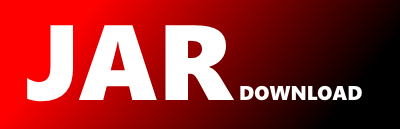
org.jgroups.tests.bla8a Maven / Gradle / Ivy
Go to download
This artifact provides a single jar that contains all classes required to use remote Jakarta Enterprise Beans and Jakarta Messaging, including
all dependencies. It is intended for use by those not using maven, maven users should just import the Jakarta Enterprise Beans and
Jakarta Messaging BOM's instead (shaded JAR's cause lots of problems with maven, as it is very easy to inadvertently end up
with different versions on classes on the class path).
package org.jgroups.tests;
/**
* @author Bela Ban
* @since x.y
*/
public class bla8a {
public static void main(String[] args) {
Shape s1=new Circle(3,4,5,6);
Shape s2=new FatCircle(3,4,5,6,7);
Shape s3=new Rectangle(3,4,5);
Shape s4=new Circle(3,4,5,6);
boolean rc=s1.equals(s2);
rc=s2.equals(s1);
rc=s1.equals(s3);
rc=s3.equals(s1);
rc=s1.equals(s4);
rc=s4.equals(s1);
}
protected static class Shape {
final int color;
public Shape(int color) {
this.color=color;
}
public boolean equals(Object obj) {
Class> my_class=getClass(), other_class=obj.getClass();
if(my_class != other_class) {
// throw new IllegalArgumentException(String.format("class mismatch: mine=%s, other=%s", my_class.getSimpleName(),other_class.getSimpleName()));
return false;
}
return color == ((Shape)obj).color;
}
}
protected static class Circle extends Shape {
final int x,y;
final int radius;
public Circle(int x, int y, int radius, int color) {
super(color);
this.x=x;
this.y=y;
this.radius=radius;
}
public boolean equals(Object obj) {
if(!super.equals(obj))
return false;
Circle oth=(Circle)obj;
return x == oth.x && y == oth.y && radius == oth.radius;
}
}
protected static class FatCircle extends Circle {
final int fatness;
public FatCircle(int x, int y, int radius, int fatness, int color) {
super(x, y, radius, color);
this.fatness=fatness;
}
public boolean equals(Object obj) {
if(!super.equals(obj))
return false;
FatCircle oth=(FatCircle)obj;
return fatness == oth.fatness;
}
}
protected static class Rectangle extends Shape {
final int x,y;
public Rectangle(int x, int y, int color) {
super(color);
this.x=x;
this.y=y;
}
public boolean equals(Object obj) {
if(!super.equals(obj))
return false;
Rectangle oth=(Rectangle)obj;
return x == oth.x && y == oth.y;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy