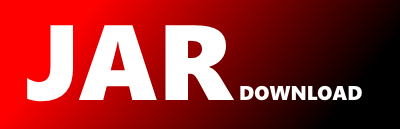
org.xnio._private.Messages_$logger Maven / Gradle / Ivy
Go to download
This artifact provides a single jar that contains all classes required to use remote Jakarta Enterprise Beans and Jakarta Messaging, including
all dependencies. It is intended for use by those not using maven, maven users should just import the Jakarta Enterprise Beans and
Jakarta Messaging BOM's instead (shaded JAR's cause lots of problems with maven, as it is very easy to inadvertently end up
with different versions on classes on the class path).
package org.xnio._private;
import java.nio.ReadOnlyBufferException;
import java.lang.IllegalStateException;
import java.io.InterruptedIOException;
import java.io.Serializable;
import org.xnio.channels.ConcurrentStreamChannelAccessException;
import org.jboss.logging.DelegatingBasicLogger;
import org.xnio.channels.ReadTimeoutException;
import java.lang.String;
import org.jboss.logging.Logger;
import javax.security.sasl.SaslException;
import org.xnio.channels.WriteTimeoutException;
import org.jboss.logging.BasicLogger;
import java.io.EOFException;
import java.lang.ClassLoader;
import java.nio.BufferOverflowException;
import org.xnio.channels.FixedLengthUnderflowException;
import java.lang.ClassNotFoundException;
import java.lang.IllegalArgumentException;
import java.nio.BufferUnderflowException;
import org.xnio.channels.FixedLengthOverflowException;
import java.lang.UnsupportedOperationException;
import org.xnio.IoFuture.Notifier;
import java.util.concurrent.TimeoutException;
import javax.net.ssl.SSLEngineResult.Status;
import javax.annotation.Generated;
import java.nio.channels.Channel;
import java.lang.SecurityException;
import java.io.IOException;
import java.io.CharConversionException;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.CancellationException;
import java.lang.Throwable;
import java.lang.Class;
import java.lang.Object;
import java.util.Arrays;
import org.xnio.channels.AcceptingChannel;
import javax.net.ssl.SSLEngineResult.HandshakeStatus;
/**
* Warning this class consists of generated code.
*/
@Generated(value = "org.jboss.logging.processor.generator.model.MessageLoggerImplementor", date = "2016-06-15T08:24:51-0500")
public class Messages_$logger extends DelegatingBasicLogger implements Messages,BasicLogger,Serializable {
private static final long serialVersionUID = 1L;
private static final String FQCN = Messages_$logger.class.getName();
public Messages_$logger(final Logger log) {
super(log);
}
@Override
public final void greeting(final String version) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.INFO, null, greeting$str(), version);
}
private static final String greeting = "XNIO version %s";
protected String greeting$str() {
return greeting;
}
private static final String nullParameter = "Method parameter '%s' cannot be null";
protected String nullParameter$str() {
return nullParameter;
}
@Override
public final IllegalArgumentException nullParameter(final String name) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(nullParameter$str(), name));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String minRange = "XNIO000001: Method parameter '%s' must be greater than %d";
protected String minRange$str() {
return minRange;
}
@Override
public final IllegalArgumentException minRange(final String paramName, final int minimumValue) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(minRange$str(), paramName, minimumValue));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String badSockType = "XNIO000002: Unsupported socket address %s";
protected String badSockType$str() {
return badSockType;
}
@Override
public final IllegalArgumentException badSockType(final Class extends java.net.SocketAddress> type) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(badSockType$str(), type));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String nullArrayIndex = "XNIO000003: Method parameter '%s' contains disallowed null value at index %d";
protected String nullArrayIndex$str() {
return nullArrayIndex;
}
@Override
public final IllegalArgumentException nullArrayIndex(final String paramName, final int index) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(nullArrayIndex$str(), paramName, index));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String mismatchSockType = "XNIO000004: Bind address %s is not the same type as destination address %s";
protected String mismatchSockType$str() {
return mismatchSockType;
}
@Override
public final IllegalArgumentException mismatchSockType(final Class extends java.net.SocketAddress> bindType, final Class extends java.net.SocketAddress> destType) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(mismatchSockType$str(), bindType, destType));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String noField = "XNIO000005: No such field named \"%s\" in %s";
protected String noField$str() {
return noField;
}
@Override
public final IllegalArgumentException noField(final String fieldName, final Class extends Object> clazz) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(noField$str(), fieldName, clazz));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String optionClassNotFound = "XNIO000006: Class \"%s\" not found in %s";
protected String optionClassNotFound$str() {
return optionClassNotFound;
}
@Override
public final IllegalArgumentException optionClassNotFound(final String className, final ClassLoader classLoader) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(optionClassNotFound$str(), className, classLoader));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String fieldNotAccessible = "XNIO000007: Field named \"%s\" is not accessible (public) in %s";
protected String fieldNotAccessible$str() {
return fieldNotAccessible;
}
@Override
public final IllegalArgumentException fieldNotAccessible(final String fieldName, final Class extends Object> clazz) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(fieldNotAccessible$str(), fieldName, clazz));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String fieldNotStatic = "XNIO000008: Field named \"%s\" is not static in %s";
protected String fieldNotStatic$str() {
return fieldNotStatic;
}
@Override
public final IllegalArgumentException fieldNotStatic(final String fieldName, final Class extends Object> clazz) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(fieldNotStatic$str(), fieldName, clazz));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String copyNegative = "XNIO000009: Copy with negative count is not supported";
protected String copyNegative$str() {
return copyNegative;
}
@Override
public final UnsupportedOperationException copyNegative() {
final UnsupportedOperationException result = new UnsupportedOperationException(String.format(copyNegative$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
@Override
public final void invalidOptionInProperty(final String optionName, final String name, final Throwable problem) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.WARN, null, invalidOptionInProperty$str(), optionName, name, problem);
}
private static final String invalidOptionInProperty = "XNIO000010: Invalid option '%s' in property '%s': %s";
protected String invalidOptionInProperty$str() {
return invalidOptionInProperty;
}
private static final String readOnlyBuffer = "XNIO000012: Attempt to write to a read-only buffer";
protected String readOnlyBuffer$str() {
return readOnlyBuffer;
}
@Override
public final ReadOnlyBufferException readOnlyBuffer() {
final ReadOnlyBufferException result = new ReadOnlyBufferException();
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String bufferUnderflow = "XNIO000013: Buffer underflow";
protected String bufferUnderflow$str() {
return bufferUnderflow;
}
@Override
public final BufferUnderflowException bufferUnderflow() {
final BufferUnderflowException result = new BufferUnderflowException();
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String bufferOverflow = "XNIO000014: Buffer overflow";
protected String bufferOverflow$str() {
return bufferOverflow;
}
@Override
public final BufferOverflowException bufferOverflow() {
final BufferOverflowException result = new BufferOverflowException();
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String parameterOutOfRange = "XNIO000015: Parameter '%s' is out of range";
protected String parameterOutOfRange$str() {
return parameterOutOfRange;
}
@Override
public final IllegalArgumentException parameterOutOfRange(final String name) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(parameterOutOfRange$str(), name));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String mixedDirectAndHeap = "XNIO000016: Mixed direct and heap buffers not allowed";
protected String mixedDirectAndHeap$str() {
return mixedDirectAndHeap;
}
@Override
public final IllegalArgumentException mixedDirectAndHeap() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(mixedDirectAndHeap$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String bufferFreed = "XNIO000017: Buffer was already freed";
protected String bufferFreed$str() {
return bufferFreed;
}
@Override
public final IllegalStateException bufferFreed() {
final IllegalStateException result = new IllegalStateException(String.format(bufferFreed$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String randomWrongThread = "XNIO000018: Access a thread-local random from the wrong thread";
protected String randomWrongThread$str() {
return randomWrongThread;
}
@Override
public final IllegalStateException randomWrongThread() {
final IllegalStateException result = new IllegalStateException(String.format(randomWrongThread$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String channelNotAvailable = "XNIO000019: Channel not available from connection";
protected String channelNotAvailable$str() {
return channelNotAvailable;
}
@Override
public final IllegalStateException channelNotAvailable() {
final IllegalStateException result = new IllegalStateException(String.format(channelNotAvailable$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String noOptionParser = "XNIO000020: No parser for this option value type";
protected String noOptionParser$str() {
return noOptionParser;
}
@Override
public final IllegalArgumentException noOptionParser() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(noOptionParser$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String invalidOptionPropertyFormat = "XNIO000021: Invalid format for property value '%s'";
protected String invalidOptionPropertyFormat$str() {
return invalidOptionPropertyFormat;
}
@Override
public final IllegalArgumentException invalidOptionPropertyFormat(final String value) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(invalidOptionPropertyFormat$str(), value));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String classNotFound = "XNIO000022: Class %s not found";
protected String classNotFound$str() {
return classNotFound;
}
@Override
public final IllegalArgumentException classNotFound(final String name, final ClassNotFoundException cause) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(classNotFound$str(), name), cause);
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String classNotInstance = "XNIO000023: Class %s is not an instance of %s";
protected String classNotInstance$str() {
return classNotInstance;
}
@Override
public final IllegalArgumentException classNotInstance(final String name, final Class extends Object> expectedType) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(classNotInstance$str(), name, expectedType));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String invalidOptionName = "XNIO000024: Invalid option name '%s'";
protected String invalidOptionName$str() {
return invalidOptionName;
}
@Override
public final IllegalArgumentException invalidOptionName(final String name) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(invalidOptionName$str(), name));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String invalidNullOption = "XNIO000025: Invalid null option '%s'";
protected String invalidNullOption$str() {
return invalidNullOption;
}
@Override
public final IllegalArgumentException invalidNullOption(final String name) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(invalidNullOption$str(), name));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String readAppendNotSupported = "XNIO000026: Read with append is not supported";
protected String readAppendNotSupported$str() {
return readAppendNotSupported;
}
@Override
public final IOException readAppendNotSupported() {
final IOException result = new IOException(String.format(readAppendNotSupported$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String openModeRequires7 = "XNIO000027: Requested file open mode requires Java 7 or higher";
protected String openModeRequires7$str() {
return openModeRequires7;
}
@Override
public final IOException openModeRequires7() {
final IOException result = new IOException(String.format(openModeRequires7$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String xnioThreadRequired = "XNIO000028: Current thread is not an XNIO I/O thread";
protected String xnioThreadRequired$str() {
return xnioThreadRequired;
}
@Override
public final IllegalStateException xnioThreadRequired() {
final IllegalStateException result = new IllegalStateException(String.format(xnioThreadRequired$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String badCompressionFormat = "XNIO000029: Compression format not supported";
protected String badCompressionFormat$str() {
return badCompressionFormat;
}
@Override
public final IllegalArgumentException badCompressionFormat() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(badCompressionFormat$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String differentWorkers = "XNIO000030: Both channels must come from the same worker";
protected String differentWorkers$str() {
return differentWorkers;
}
@Override
public final IllegalArgumentException differentWorkers() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(differentWorkers$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String oneChannelMustBeConnection = "XNIO000031: At least one channel must have a connection";
protected String oneChannelMustBeConnection$str() {
return oneChannelMustBeConnection;
}
@Override
public final IllegalArgumentException oneChannelMustBeConnection() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(oneChannelMustBeConnection$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String oneChannelMustBeSSL = "XNIO000032: At least one channel must be an SSL channel";
protected String oneChannelMustBeSSL$str() {
return oneChannelMustBeSSL;
}
@Override
public final IllegalArgumentException oneChannelMustBeSSL() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(oneChannelMustBeSSL$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String invalidQop = "XNIO000033: '%s' is not a valid QOP value";
protected String invalidQop$str() {
return invalidQop;
}
@Override
public final IllegalArgumentException invalidQop(final String name) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(invalidQop$str(), name));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String cantInstantiate = "XNIO000034: Failed to instantiate %s";
protected String cantInstantiate$str() {
return cantInstantiate;
}
@Override
public final IllegalArgumentException cantInstantiate(final Class extends Object> clazz, final Throwable cause) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(cantInstantiate$str(), clazz), cause);
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String concurrentAccess = "XNIO000035: Stream channel was accessed concurrently";
protected String concurrentAccess$str() {
return concurrentAccess;
}
@Override
public final ConcurrentStreamChannelAccessException concurrentAccess() {
final ConcurrentStreamChannelAccessException result = new ConcurrentStreamChannelAccessException(String.format(concurrentAccess$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String malformedInput = "XNIO000036: Malformed input";
protected String malformedInput$str() {
return malformedInput;
}
@Override
public final CharConversionException malformedInput() {
final CharConversionException result = new CharConversionException(String.format(malformedInput$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String unmappableCharacter = "XNIO000037: Unmappable character";
protected String unmappableCharacter$str() {
return unmappableCharacter;
}
@Override
public final CharConversionException unmappableCharacter() {
final CharConversionException result = new CharConversionException(String.format(unmappableCharacter$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String characterDecodingProblem = "XNIO000038: Character decoding problem";
protected String characterDecodingProblem$str() {
return characterDecodingProblem;
}
@Override
public final CharConversionException characterDecodingProblem() {
final CharConversionException result = new CharConversionException(String.format(characterDecodingProblem$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String missingSslProvider = "XNIO000100: 'https' URL scheme chosen but no SSL provider given";
protected String missingSslProvider$str() {
return missingSslProvider;
}
@Override
public final IllegalArgumentException missingSslProvider() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(missingSslProvider$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String invalidURLScheme = "XNIO000101: Unknown URL scheme '%s' given; must be one of 'http' or 'https'";
protected String invalidURLScheme$str() {
return invalidURLScheme;
}
@Override
public final IllegalArgumentException invalidURLScheme(final String scheme) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(invalidURLScheme$str(), scheme));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String extraChallenge = "XNIO000200: Unexpected extra SASL challenge data received";
protected String extraChallenge$str() {
return extraChallenge;
}
@Override
public final SaslException extraChallenge() {
final SaslException result = new SaslException(String.format(extraChallenge$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String extraResponse = "XNIO000201: Unexpected extra SASL response data received";
protected String extraResponse$str() {
return extraResponse;
}
@Override
public final SaslException extraResponse() {
final SaslException result = new SaslException(String.format(extraResponse$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String socketBufferTooSmall = "XNIO000300: Socket buffer is too small";
protected String socketBufferTooSmall$str() {
return socketBufferTooSmall;
}
@Override
public final IllegalArgumentException socketBufferTooSmall() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(socketBufferTooSmall$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String appBufferTooSmall = "XNIO000301: Application buffer is too small";
protected String appBufferTooSmall$str() {
return appBufferTooSmall;
}
@Override
public final IllegalArgumentException appBufferTooSmall() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(appBufferTooSmall$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String wrongBufferExpansion = "XNIO000302: SSLEngine required a bigger send buffer but our buffer was already big enough";
protected String wrongBufferExpansion$str() {
return wrongBufferExpansion;
}
@Override
public final IOException wrongBufferExpansion() {
final IOException result = new IOException(String.format(wrongBufferExpansion$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String unexpectedWrapResult = "XNIO000303: Unexpected wrap result status: %s";
protected String unexpectedWrapResult$str() {
return unexpectedWrapResult;
}
@Override
public final IOException unexpectedWrapResult(final Status status) {
final IOException result = new IOException(String.format(unexpectedWrapResult$str(), status));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String unexpectedHandshakeStatus = "XNIO000304: Unexpected handshake status: %s";
protected String unexpectedHandshakeStatus$str() {
return unexpectedHandshakeStatus;
}
@Override
public final IOException unexpectedHandshakeStatus(final HandshakeStatus status) {
final IOException result = new IOException(String.format(unexpectedHandshakeStatus$str(), status));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String unexpectedUnwrapResult = "XNIO000305: Unexpected unwrap result status: %s";
protected String unexpectedUnwrapResult$str() {
return unexpectedUnwrapResult;
}
@Override
public final IOException unexpectedUnwrapResult(final Status status) {
final IOException result = new IOException(String.format(unexpectedUnwrapResult$str(), status));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String notFromThisProvider = "XNIO000306: SSL connection is not from this provider";
protected String notFromThisProvider$str() {
return notFromThisProvider;
}
@Override
public final IllegalArgumentException notFromThisProvider() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(notFromThisProvider$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String readTimeout = "XNIO000800: Read timed out";
protected String readTimeout$str() {
return readTimeout;
}
@Override
public final ReadTimeoutException readTimeout() {
final ReadTimeoutException result = new ReadTimeoutException(String.format(readTimeout$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String writeTimeout = "XNIO000801: Write timed out";
protected String writeTimeout$str() {
return writeTimeout;
}
@Override
public final WriteTimeoutException writeTimeout() {
final WriteTimeoutException result = new WriteTimeoutException(String.format(writeTimeout$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String fixedOverflow = "XNIO000802: Write past the end of a fixed-length channel";
protected String fixedOverflow$str() {
return fixedOverflow;
}
@Override
public final FixedLengthOverflowException fixedOverflow() {
final FixedLengthOverflowException result = new FixedLengthOverflowException(String.format(fixedOverflow$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String fixedUnderflow = "XNIO000803: Close before all bytes were written to a fixed-length channel (%d bytes remaining)";
protected String fixedUnderflow$str() {
return fixedUnderflow;
}
@Override
public final FixedLengthUnderflowException fixedUnderflow(final long remaining) {
final FixedLengthUnderflowException result = new FixedLengthUnderflowException(String.format(fixedUnderflow$str(), remaining));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String recvInvalidMsgLength = "XNIO000804: Received an invalid message length of %d";
protected String recvInvalidMsgLength$str() {
return recvInvalidMsgLength;
}
@Override
public final IOException recvInvalidMsgLength(final int length) {
final IOException result = new IOException(String.format(recvInvalidMsgLength$str(), length));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String writeShutDown = "XNIO000805: Writes have been shut down";
protected String writeShutDown$str() {
return writeShutDown;
}
@Override
public final EOFException writeShutDown() {
final EOFException result = new EOFException(String.format(writeShutDown$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String txMsgTooLarge = "XNIO000806: Transmitted message is too large";
protected String txMsgTooLarge$str() {
return txMsgTooLarge;
}
@Override
public final IOException txMsgTooLarge() {
final IOException result = new IOException(String.format(txMsgTooLarge$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String unflushedData = "XNIO000807: Unflushed data truncated";
protected String unflushedData$str() {
return unflushedData;
}
@Override
public final IOException unflushedData() {
final IOException result = new IOException(String.format(unflushedData$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String interruptedIO = "XNIO000808: I/O operation was interrupted";
protected String interruptedIO$str() {
return interruptedIO;
}
@Override
public final InterruptedIOException interruptedIO() {
final InterruptedIOException result = new InterruptedIOException(String.format(interruptedIO$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
@Override
public final InterruptedIOException interruptedIO(final int bytesTransferred) {
final InterruptedIOException result = new InterruptedIOException(String.format(interruptedIO$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
result.bytesTransferred = bytesTransferred;
return result;
}
private static final String flushSmallBuffer = "XNIO000809: Cannot flush due to insufficient buffer space";
protected String flushSmallBuffer$str() {
return flushSmallBuffer;
}
@Override
public final IOException flushSmallBuffer() {
final IOException result = new IOException(String.format(flushSmallBuffer$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String deflaterState = "XNIO000810: Deflater doesn't need input, but won't produce output";
protected String deflaterState$str() {
return deflaterState;
}
@Override
public final IOException deflaterState() {
final IOException result = new IOException(String.format(deflaterState$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String inflaterNeedsDictionary = "XNIO000811: Inflater needs dictionary";
protected String inflaterNeedsDictionary$str() {
return inflaterNeedsDictionary;
}
@Override
public final IOException inflaterNeedsDictionary() {
final IOException result = new IOException(String.format(inflaterNeedsDictionary$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String connectionClosedEarly = "XNIO000812: Connection closed unexpectedly";
protected String connectionClosedEarly$str() {
return connectionClosedEarly;
}
@Override
public final EOFException connectionClosedEarly() {
final EOFException result = new EOFException(String.format(connectionClosedEarly$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String streamClosed = "XNIO000813: The stream is closed";
protected String streamClosed$str() {
return streamClosed;
}
@Override
public final IOException streamClosed() {
final IOException result = new IOException(String.format(streamClosed$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String markNotSet = "XNIO000814: Mark not set";
protected String markNotSet$str() {
return markNotSet;
}
@Override
public final IOException markNotSet() {
final IOException result = new IOException(String.format(markNotSet$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String redirect = "XNIO000816: Redirect encountered establishing connection";
protected String redirect$str() {
return redirect;
}
@Override
public final String redirect() {
return String.format(redirect$str());
}
private static final String unsupported = "XNIO000900: Method '%s' is not supported on this implementation";
protected String unsupported$str() {
return unsupported;
}
@Override
public final UnsupportedOperationException unsupported(final String methodName) {
final UnsupportedOperationException result = new UnsupportedOperationException(String.format(unsupported$str(), methodName));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String blockingNotAllowed = "XNIO001000: Blocking I/O is not allowed on the current thread";
protected String blockingNotAllowed$str() {
return blockingNotAllowed;
}
@Override
public final IllegalStateException blockingNotAllowed() {
final IllegalStateException result = new IllegalStateException(String.format(blockingNotAllowed$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String noProviderFound = "XNIO001001: No XNIO provider found";
protected String noProviderFound$str() {
return noProviderFound;
}
@Override
public final IllegalArgumentException noProviderFound() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(noProviderFound$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String opCancelled = "XNIO001002: Operation was cancelled";
protected String opCancelled$str() {
return opCancelled;
}
@Override
public final CancellationException opCancelled() {
final CancellationException result = new CancellationException(String.format(opCancelled$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
@Override
public final void notifierFailed(final Throwable cause, final Notifier extends Object, ? extends Object> notifier) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.WARN, cause, notifierFailed$str(), notifier);
}
private static final String notifierFailed = "XNIO001003: Running IoFuture notifier %s failed";
protected String notifierFailed$str() {
return notifierFailed;
}
private static final String opTimedOut = "XNIO001004: Operation timed out";
protected String opTimedOut$str() {
return opTimedOut;
}
@Override
public final TimeoutException opTimedOut() {
final TimeoutException result = new TimeoutException(String.format(opTimedOut$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
private static final String propReadForbidden = "XNIO001005: Not allowed to read non-XNIO properties";
protected String propReadForbidden$str() {
return propReadForbidden;
}
@Override
public final SecurityException propReadForbidden() {
final SecurityException result = new SecurityException(String.format(propReadForbidden$str()));
final StackTraceElement[] st = result.getStackTrace();
result.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
return result;
}
@Override
public final void failedToInvokeFileWatchCallback(final Throwable cause) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.ERROR, cause, failedToInvokeFileWatchCallback$str());
}
private static final String failedToInvokeFileWatchCallback = "XNIO001006: Failed to invoke file watch callback";
protected String failedToInvokeFileWatchCallback$str() {
return failedToInvokeFileWatchCallback;
}
@Override
public final void listenerException(final Throwable cause) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.ERROR, cause, listenerException$str());
}
private static final String listenerException = "XNIO001007: A channel event listener threw an exception";
protected String listenerException$str() {
return listenerException;
}
@Override
public final void exceptionHandlerException(final Throwable cause) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.ERROR, cause, exceptionHandlerException$str());
}
private static final String exceptionHandlerException = "XNIO001008: A channel exception handler threw an exception";
protected String exceptionHandlerException$str() {
return exceptionHandlerException;
}
@Override
public final void acceptFailed(final AcceptingChannel extends org.xnio.channels.ConnectedChannel> channel, final IOException reason) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.ERROR, null, acceptFailed$str(), channel, reason);
}
private static final String acceptFailed = "XNIO001009: Failed to accept a connection on %s: %s";
protected String acceptFailed$str() {
return acceptFailed;
}
@Override
public final void executorSubmitFailed(final RejectedExecutionException cause, final Channel channel) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.ERROR, null, executorSubmitFailed$str(), cause, channel);
}
private static final String executorSubmitFailed = "XNIO001010: Failed to submit task to executor: %s (closing %s)";
protected String executorSubmitFailed$str() {
return executorSubmitFailed;
}
@Override
public final void closingResource(final Object resource) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.TRACE, null, closingResource$str(), resource);
}
private static final String closingResource = "Closing resource %s";
protected String closingResource$str() {
return closingResource;
}
@Override
public final void resourceCloseFailed(final Throwable cause, final Object resource) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.TRACE, cause, resourceCloseFailed$str(), resource);
}
private static final String resourceCloseFailed = "Closing resource %s failed";
protected String resourceCloseFailed$str() {
return resourceCloseFailed;
}
@Override
public final void resourceReadShutdownFailed(final Throwable cause, final Object resource) {
super.log.logf(FQCN, org.jboss.logging.Logger.Level.TRACE, cause, resourceReadShutdownFailed$str(), resource);
}
private static final String resourceReadShutdownFailed = "Shutting down reads on %s failed";
protected String resourceReadShutdownFailed$str() {
return resourceReadShutdownFailed;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy