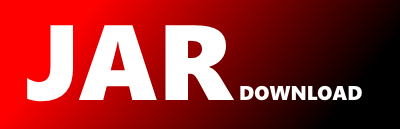
com.fasterxml.jackson.databind.ser.AnyGetterWriter Maven / Gradle / Ivy
Go to download
This artifact provides a single jar that contains all classes required to use remote Jakarta Enterprise Beans and Jakarta Messaging, including
all dependencies. It is intended for use by those not using maven, maven users should just import the Jakarta Enterprise Beans and
Jakarta Messaging BOM's instead (shaded JAR's cause lots of problems with maven, as it is very easy to inadvertently end up
with different versions on classes on the class path).
package com.fasterxml.jackson.databind.ser;
import java.util.Map;
import com.fasterxml.jackson.core.*;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.introspect.AnnotatedMember;
import com.fasterxml.jackson.databind.ser.std.MapSerializer;
/**
* Class similar to {@link BeanPropertyWriter}, but that will be used
* for serializing {@link com.fasterxml.jackson.annotation.JsonAnyGetter} annotated
* (Map) properties
*/
public class AnyGetterWriter
{
protected final BeanProperty _property;
/**
* Method (or field) that represents the "any getter"
*/
protected final AnnotatedMember _accessor;
protected JsonSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy