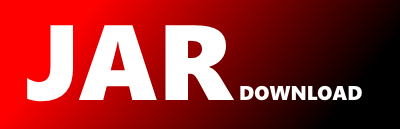
com.fasterxml.jackson.databind.ser.impl.ReadOnlyClassToSerializerMap Maven / Gradle / Ivy
package com.fasterxml.jackson.databind.ser.impl;
import java.util.*;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.util.TypeKey;
/**
* Optimized lookup table for accessing two types of serializers; typed
* and non-typed. Only accessed from a single thread, so no synchronization
* needed for accessors.
*
* Note that before 2.6 this class was much smaller, and referred most
* operations to separate JsonSerializerMap
, but in 2.6
* functions were combined.
*/
public final class ReadOnlyClassToSerializerMap
{
private final Bucket[] _buckets;
private final int _size;
private final int _mask;
public ReadOnlyClassToSerializerMap(Map> serializers)
{
int size = findSize(serializers.size());
_size = size;
_mask = (size-1);
Bucket[] buckets = new Bucket[size];
for (Map.Entry> entry : serializers.entrySet()) {
TypeKey key = entry.getKey();
int index = key.hashCode() & _mask;
buckets[index] = new Bucket(buckets[index], key, entry.getValue());
}
_buckets = buckets;
}
private final static int findSize(int size)
{
// For small enough results (64 or less), we'll require <= 50% fill rate; otherwise 80%
int needed = (size <= 64) ? (size + size) : (size + (size >> 2));
int result = 8;
while (result < needed) {
result += result;
}
return result;
}
/**
* Factory method for constructing an instance.
*/
public static ReadOnlyClassToSerializerMap from(HashMap> src) {
return new ReadOnlyClassToSerializerMap(src);
}
/*
/**********************************************************
/* Public API
/**********************************************************
*/
public int size() { return _size; }
public JsonSerializer