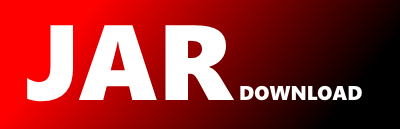
org.wildfly.clustering.faces.mojarra.util.LRUMapMarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wildfly-clustering-faces-mojarra Show documentation
Show all versions of wildfly-clustering-faces-mojarra Show documentation
Marshalling optimizations for Mojarra.
The newest version!
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.wildfly.clustering.faces.mojarra.util;
import java.io.IOException;
import java.lang.reflect.Field;
import java.security.PrivilegedAction;
import java.util.AbstractMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.infinispan.protostream.descriptors.WireType;
import org.wildfly.clustering.marshalling.protostream.ProtoStreamReader;
import org.wildfly.clustering.marshalling.protostream.ProtoStreamWriter;
import org.wildfly.clustering.marshalling.protostream.util.AbstractMapMarshaller;
import org.wildfly.security.manager.WildFlySecurityManager;
import com.sun.faces.util.LRUMap;
/**
* @author Paul Ferraro
*/
public class LRUMapMarshaller extends AbstractMapMarshaller> {
private static final int MAX_CAPACITY_INDEX = ENTRY_INDEX + 1;
private static final int DEFAULT_MAX_CAPACITY = 15;
private static final Field MAX_CAPACITY_FIELD = WildFlySecurityManager.doUnchecked(new PrivilegedAction() {
@Override
public Field run() {
for (Field field : LRUMap.class.getDeclaredFields()) {
if (field.getType() == Integer.TYPE) {
field.setAccessible(true);
return field;
}
}
throw new IllegalStateException();
}
});
@SuppressWarnings("unchecked")
public LRUMapMarshaller() {
super((Class>) (Class>) LRUMap.class);
}
@Override
public LRUMap
© 2015 - 2024 Weber Informatics LLC | Privacy Policy