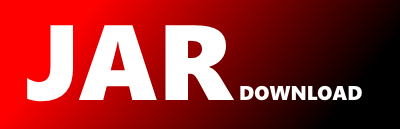
org.jboss.as.ee.component.BasicComponent Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.jboss.as.ee.component;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
import org.jboss.as.ee.logging.EeLogger;
import org.jboss.as.ee.component.interceptors.InvocationType;
import org.jboss.as.naming.ImmediateManagedReference;
import org.jboss.as.naming.ManagedReference;
import org.jboss.as.naming.context.NamespaceContextSelector;
import org.jboss.invocation.Interceptor;
import org.jboss.invocation.InterceptorContext;
import org.jboss.invocation.InterceptorFactory;
import org.jboss.invocation.InterceptorFactoryContext;
import org.jboss.invocation.SimpleInterceptorFactoryContext;
import org.jboss.msc.service.ServiceName;
/**
* A basic component implementation.
*
* @author John Bailey
* @author David M. Lloyd
*/
public class BasicComponent implements Component {
private final String componentName;
private final Class> componentClass;
private final InterceptorFactory postConstruct;
private final InterceptorFactory preDestroy;
private final Map interceptorFactoryMap;
private final NamespaceContextSelector namespaceContextSelector;
private final ServiceName createServiceName;
private volatile boolean gate;
private final AtomicBoolean stopping = new AtomicBoolean();
private Interceptor postConstructInterceptor;
private Interceptor preDestroyInterceptor;
private Map interceptorInstanceMap;
/**
* Construct a new instance.
*
* @param createService the create service which created this component
*/
public BasicComponent(final BasicComponentCreateService createService) {
componentName = createService.getComponentName();
componentClass = createService.getComponentClass();
postConstruct = createService.getPostConstruct();
preDestroy = createService.getPreDestroy();
interceptorFactoryMap = createService.getComponentInterceptors();
namespaceContextSelector = createService.getNamespaceContextSelector();
createServiceName = createService.getServiceName();
}
/**
* {@inheritDoc}
*/
public ComponentInstance createInstance() {
BasicComponentInstance instance = constructComponentInstance(null, true);
return instance;
}
/**
* Wraps an existing object instance in a ComponentInstance, and run the post construct interceptor chain on it.
*
* @param instance The instance to wrap
* @return The new ComponentInstance
*/
public ComponentInstance createInstance(Object instance) {
BasicComponentInstance obj = constructComponentInstance(new ImmediateManagedReference(instance), true);
obj.constructionFinished();
return obj;
}
@Override
public ComponentInstance getInstance(Object instance) {
BasicComponentInstance obj = constructComponentInstance(new ImmediateManagedReference(instance), false);
obj.constructionFinished();
return obj;
}
public void waitForComponentStart() {
if (!gate) {
EeLogger.ROOT_LOGGER.tracef("Waiting for component %s (%s)", componentName, componentClass);
// Block until successful start
synchronized (this) {
if (stopping.get()) {
throw EeLogger.ROOT_LOGGER.componentIsStopped();
}
while (!gate) {
// TODO: check for failure condition
try {
wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw EeLogger.ROOT_LOGGER.componentNotAvailable();
}
}
}
EeLogger.ROOT_LOGGER.tracef("Finished waiting for component %s (%s)", componentName, componentClass);
}
}
/**
* Construct the component instance. Upon return, the object instance should have injections and lifecycle
* invocations completed already.
*
*
* @param instance An instance to be wrapped, or null if a new instance should be created
* @return the component instance
*/
protected BasicComponentInstance constructComponentInstance(ManagedReference instance, boolean invokePostConstruct) {
return constructComponentInstance(instance, invokePostConstruct, Collections.emptyMap());
}
/**
* Construct the component instance. Upon return, the object instance should have injections and lifecycle
* invocations completed already.
*
*
* @param instance An instance to be wrapped, or null if a new instance should be created
* @return the component instance
*/
protected BasicComponentInstance constructComponentInstance(ManagedReference instance, boolean invokePostConstruct, final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy