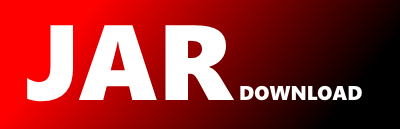
org.jboss.as.ee.component.ViewService Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.jboss.as.ee.component;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.jboss.as.ee.logging.EeLogger;
import org.jboss.as.ee.utils.DescriptorUtils;
import org.jboss.as.naming.ManagedReference;
import org.jboss.invocation.Interceptor;
import org.jboss.invocation.InterceptorContext;
import org.jboss.invocation.InterceptorFactory;
import org.jboss.invocation.Interceptors;
import org.jboss.invocation.SimpleInterceptorFactoryContext;
import org.jboss.invocation.proxy.ProxyFactory;
import org.jboss.msc.inject.Injector;
import org.jboss.msc.service.Service;
import org.jboss.msc.service.StartContext;
import org.jboss.msc.service.StartException;
import org.jboss.msc.service.StopContext;
import org.jboss.msc.value.InjectedValue;
import org.wildfly.security.manager.WildFlySecurityManager;
import static org.jboss.as.ee.logging.EeLogger.ROOT_LOGGER;
/**
* @author David M. Lloyd
*/
public final class ViewService implements Service {
private final InjectedValue componentInjector = new InjectedValue();
private final Map viewInterceptorFactories;
private final Map clientInterceptorFactories;
private final InterceptorFactory clientPostConstruct;
private final InterceptorFactory clientPreDestroy;
private final ProxyFactory> proxyFactory;
private final Class> viewClass;
private final Set asyncMethods;
private final ViewInstanceFactory viewInstanceFactory;
private final Map, Object> privateData;
private volatile ComponentView view;
private volatile Interceptor clientPostConstructInterceptor;
private volatile Interceptor clientPreDestroyInterceptor;
private volatile Map clientInterceptors;
public ViewService(final ViewConfiguration viewConfiguration) {
viewClass = viewConfiguration.getViewClass();
final ProxyFactory> proxyFactory = viewConfiguration.getProxyFactory();
this.proxyFactory = proxyFactory;
final List methods = proxyFactory.getCachedMethods();
final int methodCount = methods.size();
clientPostConstruct = Interceptors.getChainedInterceptorFactory(viewConfiguration.getClientPostConstructInterceptors());
clientPreDestroy = Interceptors.getChainedInterceptorFactory(viewConfiguration.getClientPreDestroyInterceptors());
final IdentityHashMap viewInterceptorFactories = new IdentityHashMap(methodCount);
final IdentityHashMap clientInterceptorFactories = new IdentityHashMap(methodCount);
for (final Method method : methods) {
if (method.getName().equals("finalize") && method.getParameterCount() == 0) {
viewInterceptorFactories.put(method, Interceptors.getTerminalInterceptorFactory());
} else {
viewInterceptorFactories.put(method, Interceptors.getChainedInterceptorFactory(viewConfiguration.getViewInterceptors(method)));
clientInterceptorFactories.put(method, Interceptors.getChainedInterceptorFactory(viewConfiguration.getClientInterceptors(method)));
}
}
this.viewInterceptorFactories = viewInterceptorFactories;
this.clientInterceptorFactories = clientInterceptorFactories;
this.asyncMethods = viewConfiguration.getAsyncMethods();
if (viewConfiguration.getViewInstanceFactory() == null) {
viewInstanceFactory = new DefaultViewInstanceFactory();
} else {
viewInstanceFactory = viewConfiguration.getViewInstanceFactory();
}
if(viewConfiguration.getPrivateData().isEmpty()) {
privateData = Collections.emptyMap();
} else {
privateData = viewConfiguration.getPrivateData();
}
}
public void start(final StartContext context) throws StartException {
// Construct the view
View view = new View(privateData);
view.initializeInterceptors();
this.view = view;
final SimpleInterceptorFactoryContext factoryContext = new SimpleInterceptorFactoryContext();
final Component component = view.getComponent();
factoryContext.getContextData().put(Component.class, component);
factoryContext.getContextData().put(ComponentView.class, view);
clientPostConstructInterceptor = clientPostConstruct.create(factoryContext);
clientPreDestroyInterceptor = clientPreDestroy.create(factoryContext);
final Map clientInterceptorFactories = ViewService.this.clientInterceptorFactories;
clientInterceptors = new IdentityHashMap(clientInterceptorFactories.size());
for (Map.Entry entry : clientInterceptorFactories.entrySet()) {
clientInterceptors.put(entry.getKey(), entry.getValue().create(factoryContext));
}
}
public void stop(final StopContext context) {
view = null;
}
public Injector getComponentInjector() {
return componentInjector;
}
public ComponentView getValue() throws IllegalStateException, IllegalArgumentException {
return view;
}
class View implements ComponentView {
private final Component component;
private final Map viewInterceptors;
private final Map methods;
private final Map, Object> privateData;
View(final Map, Object> privateData) {
this.privateData = privateData;
component = componentInjector.getValue();
//we need to build the view interceptor chain
this.viewInterceptors = new IdentityHashMap();
this.methods = new HashMap();
}
void initializeInterceptors() {
final SimpleInterceptorFactoryContext factoryContext = new SimpleInterceptorFactoryContext();
final Map viewInterceptorFactories = ViewService.this.viewInterceptorFactories;
factoryContext.getContextData().put(Component.class, component);
//we don't have this code in the constructor so we avoid passing around
//a half constructed instance
factoryContext.getContextData().put(ComponentView.class, this);
for (Map.Entry entry : viewInterceptorFactories.entrySet()) {
Method method = entry.getKey();
viewInterceptors.put(method, entry.getValue().create(factoryContext));
methods.put(new MethodDescription(method.getName(), DescriptorUtils.methodDescriptor(method)), method);
}
}
public ManagedReference createInstance() throws Exception {
return createInstance(Collections.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy