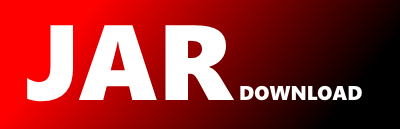
org.jboss.as.ee.metadata.MethodAnnotationAggregator Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.jboss.as.ee.metadata;
import org.jboss.as.ee.logging.EeLogger;
import org.jboss.as.ee.component.EEApplicationClasses;
import org.jboss.as.ee.component.EEModuleClassDescription;
import org.jboss.as.server.deployment.reflect.ClassReflectionIndex;
import org.jboss.as.server.deployment.reflect.DeploymentReflectionIndex;
import org.jboss.invocation.proxy.MethodIdentifier;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Class which can turn a pre-runtime description of annotations into a runtime description.
*
* This correctly handles overridden methods, so the annotations on overridden methods will not show up in the result
*
* @author Stuart Douglas
*/
public class MethodAnnotationAggregator {
public static RuntimeAnnotationInformation runtimeAnnotationInformation(final Class> componentClass, final EEApplicationClasses applicationClasses, final DeploymentReflectionIndex index, final Class annotationType) {
final HashSet methodIdentifiers = new HashSet();
final Map> methods = new HashMap>();
final Map> classAnnotations = new HashMap>();
Class> c = componentClass;
while (c != null && c != Object.class) {
final ClassReflectionIndex classIndex = index.getClassIndex(c);
final EEModuleClassDescription description = applicationClasses.getClassByName(c.getName());
if (description != null) {
ClassAnnotationInformation annotationData = description.getAnnotationInformation(annotationType);
if (annotationData != null) {
if (!annotationData.getClassLevelAnnotations().isEmpty()) {
classAnnotations.put(c.getName(), annotationData.getClassLevelAnnotations());
}
for (Map.Entry> entry : annotationData.getMethodLevelAnnotations().entrySet()) {
final Method method = classIndex.getMethod(entry.getKey());
if (method != null) {
//we do not have to worry about private methods being overridden
if (Modifier.isPrivate(method.getModifiers()) || !methodIdentifiers.contains(entry.getKey())) {
methods.put(method, entry.getValue());
}
} else {
//this should not happen
//but if it does, we give some info
throw EeLogger.ROOT_LOGGER.cannotResolveMethod(entry.getKey(), c, entry.getValue());
}
}
}
}
//we store all the method identifiers
//so we can check if a method is overriden
for (Method method : (Iterable)classIndex.getMethods()) {
//we do not have to worry about private methods being overridden
if (!Modifier.isPrivate(method.getModifiers())) {
methodIdentifiers.add(MethodIdentifier.getIdentifierForMethod(method));
}
}
c = c.getSuperclass();
}
return new RuntimeAnnotationInformation(classAnnotations, methods);
}
public static Set runtimeAnnotationPresent(final Class> componentClass, final EEApplicationClasses applicationClasses, final DeploymentReflectionIndex index, final Class annotationType) {
RuntimeAnnotationInformation
© 2015 - 2025 Weber Informatics LLC | Privacy Policy