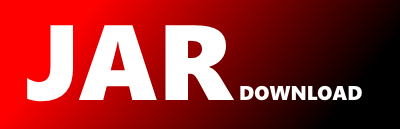
org.jboss.as.ee.naming.InjectedEENamespaceContextSelector Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.jboss.as.ee.naming;
import javax.naming.CompositeName;
import javax.naming.Context;
import javax.naming.NamingException;
import org.jboss.as.naming.NamingStore;
import org.jboss.as.naming.context.NamespaceContextSelector;
import org.jboss.as.server.deployment.DelegatingSupplier;
/**
* A simple EE-style namespace context selector which uses injected services for the contexts.
*
* @author David M. Lloyd
* @author Richard Opalka
*/
public final class InjectedEENamespaceContextSelector extends NamespaceContextSelector {
private static final CompositeName EMPTY_NAME = new CompositeName();
private final DelegatingSupplier jbossContext = new DelegatingSupplier<>();
private final DelegatingSupplier globalContext = new DelegatingSupplier<>();
private final DelegatingSupplier appContext = new DelegatingSupplier<>();
private final DelegatingSupplier moduleContext = new DelegatingSupplier<>();
private final DelegatingSupplier compContext = new DelegatingSupplier<>();
private final DelegatingSupplier exportedContext = new DelegatingSupplier<>();
public InjectedEENamespaceContextSelector() {
}
public DelegatingSupplier getAppContextSupplier() {
return appContext;
}
public DelegatingSupplier getModuleContextSupplier() {
return moduleContext;
}
public DelegatingSupplier getCompContextSupplier() {
return compContext;
}
public DelegatingSupplier getJbossContextSupplier() {
return jbossContext;
}
public DelegatingSupplier getGlobalContextSupplier() {
return globalContext;
}
public DelegatingSupplier getExportedContextSupplier() {
return exportedContext;
}
private NamingStore getNamingStore(final String identifier) {
if (identifier.equals("jboss")) {
return jbossContext.get();
} else if (identifier.equals("global")) {
return globalContext.get();
} else if (identifier.equals("app")) {
return appContext.get();
} else if (identifier.equals("module")) {
return moduleContext.get();
} else if (identifier.equals("comp")) {
return compContext.get();
} else if (identifier.equals("jboss/exported")) {
return exportedContext.get();
} else {
return null;
}
}
public Context getContext(final String identifier) {
NamingStore namingStore = getNamingStore(identifier);
if (namingStore != null) {
try {
return (Context) namingStore.lookup(EMPTY_NAME);
} catch (NamingException e) {
throw new IllegalStateException(e);
}
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy