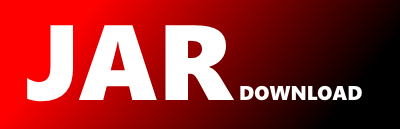
org.jboss.as.ee.component.EEModuleDescription Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.jboss.as.ee.component;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jboss.as.ee.logging.EeLogger;
import org.jboss.as.ee.component.interceptors.InterceptorClassDescription;
import org.jboss.as.ee.concurrent.ConcurrentContext;
import org.jboss.as.ee.naming.InjectedEENamespaceContextSelector;
import org.jboss.msc.service.ServiceName;
/**
* @author David M. Lloyd
*/
public final class EEModuleDescription implements ResourceInjectionTarget {
private final String applicationName;
private volatile String moduleName;
private final String earApplicationName;
//distinct name defaults to the empty string
private volatile String distinctName = "";
private final Map componentsByName = new HashMap();
private final Map> componentsByClassName = new HashMap>();
private final Map classDescriptions = new HashMap();
private final Map interceptorClassOverrides = new HashMap();
/**
* Additional interceptor environment that was defined in the deployment descriptor element.
*/
private final Map interceptorEnvironment = new HashMap();
/**
* A map of message destinations names to their resolved JNDI name
*/
private final Map messageDestinations = new HashMap();
private InjectedEENamespaceContextSelector namespaceContextSelector;
// Module Bindings
private final List bindingConfigurations = new ArrayList();
//injections that have been set in the components deployment descriptor
private final Map> resourceInjections = new HashMap>();
private final boolean appClient;
private ServiceName defaultClassIntrospectorServiceName = ReflectiveClassIntrospector.SERVICE_NAME;
private final ConcurrentContext concurrentContext;
private final EEDefaultResourceJndiNames defaultResourceJndiNames;
/**
* The default security domain for the module.
*/
private String defaultSecurityDomain;
/**
* The number of registered startup beans.
*/
private int startupBeansCount;
/**
* Construct a new instance.
*
* @param applicationName the application name (which is same as the module name if the .ear is absent)
* @param moduleName the module name
* @param earApplicationName The application name (which is null if the .ear is absent)
* @param appClient indicates if the process type is an app client
*/
public EEModuleDescription(final String applicationName, final String moduleName, final String earApplicationName, final boolean appClient) {
this.applicationName = applicationName;
this.moduleName = moduleName;
this.earApplicationName = earApplicationName;
this.appClient = appClient;
this.concurrentContext = new ConcurrentContext();
this.defaultResourceJndiNames = new EEDefaultResourceJndiNames();
}
/**
* Adds or retrieves an existing EEModuleClassDescription for the local module. This method should only be used
* for classes that reside within the current deployment unit, usually by annotation scanners that are attaching annotation
* information.
*
* This
*
* @param className The class name
* @return The new or existing {@link EEModuleClassDescription}
*/
public EEModuleClassDescription addOrGetLocalClassDescription(final String className) {
if (className == null) {
throw EeLogger.ROOT_LOGGER.nullVar("className", "module", moduleName);
}
EEModuleClassDescription ret = classDescriptions.get(className);
if (ret == null) {
classDescriptions.put(className, ret = new EEModuleClassDescription(className));
}
return ret;
}
/**
* Returns a class that is local to this module
*
* @param className The class
* @return The description, or null if not found
*/
EEModuleClassDescription getClassDescription(final String className) {
return classDescriptions.get(className);
}
/**
* Returns all class descriptions in this module
*
* @return All class descriptions
*/
public Collection getClassDescriptions() {
return classDescriptions.values();
}
public ServiceName getDefaultClassIntrospectorServiceName() {
return defaultClassIntrospectorServiceName;
}
public void setDefaultClassIntrospectorServiceName(ServiceName defaultClassIntrospectorServiceName) {
this.defaultClassIntrospectorServiceName = defaultClassIntrospectorServiceName;
}
/**
* Add a component to this module.
*
* @param description the component description
*/
public void addComponent(ComponentDescription description) {
final String componentName = description.getComponentName();
final String componentClassName = description.getComponentClassName();
if (componentName == null) {
throw EeLogger.ROOT_LOGGER.nullVar("componentName", "module", moduleName);
}
if (componentClassName == null) {
throw EeLogger.ROOT_LOGGER.nullVar("componentClassName","module", moduleName);
}
if (componentsByName.containsKey(componentName)) {
ComponentDescription existingComponent = componentsByName.get(componentName);
throw EeLogger.ROOT_LOGGER.componentAlreadyDefined(componentName, componentClassName,
existingComponent.getComponentClassName());
}
componentsByName.put(componentName, description);
List list = componentsByClassName.get(componentClassName);
if (list == null) {
componentsByClassName.put(componentClassName, list = new ArrayList(1));
}
list.add(description);
}
public void removeComponent(final String componentName, final String componentClassName) {
componentsByName.remove(componentName);
componentsByClassName.remove(componentClassName);
}
/**
* Returns the application name which can be the same as the module name, in the absence of a .ear top level
* deployment
*
* @return
* @see {@link #getEarApplicationName()}
*/
public String getApplicationName() {
//if no application name is set just return the module name
//this means that if the module name is changed the application name
//will change as well
if(applicationName == null) {
return moduleName;
}
return applicationName;
}
public String getModuleName() {
return moduleName;
}
public boolean hasComponent(final String name) {
return componentsByName.containsKey(name);
}
/**
* @return true if the process type is an app client
*/
public boolean isAppClient() {
return appClient;
}
public void setModuleName(String moduleName) {
this.moduleName = moduleName;
}
public ComponentDescription getComponentByName(String name) {
return componentsByName.get(name);
}
public List getComponentsByClassName(String className) {
final List ret = componentsByClassName.get(className);
return ret == null ? Collections.emptyList() : ret;
}
public Collection getComponentDescriptions() {
return componentsByName.values();
}
public InjectedEENamespaceContextSelector getNamespaceContextSelector() {
return namespaceContextSelector;
}
public void setNamespaceContextSelector(InjectedEENamespaceContextSelector namespaceContextSelector) {
this.namespaceContextSelector = namespaceContextSelector;
}
public String getDistinctName() {
return distinctName;
}
public void setDistinctName(String distinctName) {
if (distinctName == null) {
throw EeLogger.ROOT_LOGGER.nullVar("distinctName", "module", moduleName);
}
this.distinctName = distinctName;
}
/**
* Unlike the {@link #getApplicationName()} which follows the Jakarta EE spec semantics i.e. application name is the
* name of the top level deployment (even if it is just a jar and not an ear), this method returns the
* application name which follows the Jakarta Enterprise Beans spec semantics i.e. the application name is the
* .ear name or any configured value in application.xml. This method returns null in the absence of a .ear
*
* @return
*/
public String getEarApplicationName() {
return this.earApplicationName;
}
/**
* Get module level interceptor method overrides that are set up in ejb-jar.xml
*
* @param className The class name
* @return The overrides, or null if no overrides have been set up
*/
public InterceptorClassDescription getInterceptorClassOverride(final String className) {
return interceptorClassOverrides.get(className);
}
/**
* Adds a module level interceptor class override, it is merged with any existing overrides if they exist
*
* @param className The class name
* @param override The override
*/
public void addInterceptorMethodOverride(final String className, final InterceptorClassDescription override) {
interceptorClassOverrides.put(className, InterceptorClassDescription.merge(interceptorClassOverrides.get(className), override));
}
public List getBindingConfigurations() {
return bindingConfigurations;
}
public void addResourceInjection(final ResourceInjectionConfiguration injection) {
String className = injection.getTarget().getClassName();
Map map = resourceInjections.get(className);
if (map == null) {
resourceInjections.put(className, map = new HashMap());
}
map.put(injection.getTarget(), injection);
}
public Map getResourceInjections(final String className) {
Map injections = resourceInjections.get(className);
if (injections == null) {
return Collections.emptyMap();
} else {
return Collections.unmodifiableMap(injections);
}
}
public void addMessageDestination(final String name, final String jndiName) {
messageDestinations.put(name, jndiName);
}
public Map getMessageDestinations() {
return Collections.unmodifiableMap(messageDestinations);
}
public void addInterceptorEnvironment(final String interceptorClassName, final InterceptorEnvironment env) {
interceptorEnvironment.put(interceptorClassName, env);
}
public Map getInterceptorEnvironment() {
return interceptorEnvironment;
}
public ConcurrentContext getConcurrentContext() {
return concurrentContext;
}
public EEDefaultResourceJndiNames getDefaultResourceJndiNames() {
return defaultResourceJndiNames;
}
public String getDefaultSecurityDomain() {
return defaultSecurityDomain;
}
public void setDefaultSecurityDomain(String defaultSecurityDomain) {
this.defaultSecurityDomain = defaultSecurityDomain;
}
public int getStartupBeansCount() {
return this.startupBeansCount;
}
public int registerStartupBean() {
return ++this.startupBeansCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy