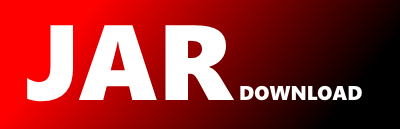
org.jboss.as.host.controller.ServerInventoryService Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2011, Red Hat, Inc., and individual contributors
* as indicated by the @author tags. See the copyright.txt file in the
* distribution for a full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.as.host.controller;
import static org.jboss.as.host.controller.HostControllerLogger.ROOT_LOGGER;
import java.net.InetSocketAddress;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.concurrent.RejectedExecutionException;
import org.jboss.as.controller.extension.ExtensionRegistry;
import org.jboss.as.domain.controller.DomainController;
import org.jboss.as.network.NetworkInterfaceBinding;
import org.jboss.as.remoting.management.ManagementChannelRegistryService;
import org.jboss.as.server.services.net.NetworkInterfaceService;
import org.jboss.msc.service.Service;
import org.jboss.msc.service.ServiceName;
import org.jboss.msc.service.ServiceTarget;
import org.jboss.msc.service.StartContext;
import org.jboss.msc.service.StartException;
import org.jboss.msc.service.StopContext;
import org.jboss.msc.value.InjectedValue;
import org.jboss.threads.AsyncFutureTask;
/**
* Service providing the {@link ServerInventory}
*
* @author Emanuel Muckenhuber
* @author David M. Lloyd
*/
class ServerInventoryService implements Service {
static final ServiceName SERVICE_NAME = ServiceName.JBOSS.append("host", "controller", "server-inventory");
private final InjectedValue client = new InjectedValue();
private final InjectedValue interfaceBinding = new InjectedValue();
private final InjectedValue serverCallback = new InjectedValue();
private final DomainController domainController;
private final HostControllerEnvironment environment;
private final HostRunningModeControl runningModeControl;
private final ExtensionRegistry extensionRegistry;
private final int port;
private final InjectedValue executorService = new InjectedValue();
private final FutureServerInventory futureInventory = new FutureServerInventory();
private ServerInventoryImpl serverInventory;
private ServerInventoryService(final DomainController domainController, final HostRunningModeControl runningModeControl,
final HostControllerEnvironment environment, final ExtensionRegistry extensionRegistry, final int port) {
this.extensionRegistry = extensionRegistry;
this.domainController = domainController;
this.runningModeControl = runningModeControl;
this.environment = environment;
this.port = port;
}
static Future install(final ServiceTarget serviceTarget, final DomainController domainController, final HostRunningModeControl runningModeControl, final HostControllerEnvironment environment,
final ExtensionRegistry extensionRegistry,
final String interfaceBinding, final int port){
final ServerInventoryService inventory = new ServerInventoryService(domainController, runningModeControl, environment, extensionRegistry, port);
serviceTarget.addService(ServerInventoryService.SERVICE_NAME, inventory)
.addDependency(HostControllerService.HC_EXECUTOR_SERVICE_NAME, ExecutorService.class, inventory.executorService)
.addDependency(ProcessControllerConnectionService.SERVICE_NAME, ProcessControllerConnectionService.class, inventory.getClient())
.addDependency(NetworkInterfaceService.JBOSS_NETWORK_INTERFACE.append(interfaceBinding), NetworkInterfaceBinding.class, inventory.interfaceBinding)
.addDependency(ServerInventoryCallbackService.SERVICE_NAME, ServerInventoryCallbackService.class, inventory.serverCallback)
.addDependency(ManagementChannelRegistryService.SERVICE_NAME)
.install();
return inventory.futureInventory;
}
/** {@inheritDoc} */
@Override
public synchronized void start(StartContext context) throws StartException {
ROOT_LOGGER.debug("Starting Host Controller Server Inventory");
try {
final ProcessControllerConnectionService processControllerConnectionService = client.getValue();
final InetSocketAddress binding = new InetSocketAddress(interfaceBinding.getValue().getAddress(), port);
serverInventory = new ServerInventoryImpl(domainController, environment, binding, processControllerConnectionService.getClient(), extensionRegistry);
processControllerConnectionService.setServerInventory(serverInventory);
serverCallback.getValue().setCallbackHandler(serverInventory.getServerCallbackHandler());
futureInventory.setInventory(serverInventory);
} catch (Exception e) {
futureInventory.setFailure(e);
throw new StartException(e);
}
}
/**
* Stops all servers.
*
* {@inheritDoc}
*/
@Override
public synchronized void stop(final StopContext context) {
final boolean shutdownServers = runningModeControl.getRestartMode() == RestartMode.SERVERS;
if (shutdownServers) {
Runnable task = new Runnable() {
@Override
public void run() {
try {
serverInventory.shutdown(true, -1, true); // TODO graceful shutdown
serverInventory = null;
// client.getValue().setServerInventory(null);
} finally {
serverCallback.getValue().setCallbackHandler(null);
context.complete();
}
}
};
try {
executorService.getValue().execute(task);
} catch (RejectedExecutionException e) {
task.run();
} finally {
context.asynchronous();
}
} else {
// We have to set the shutdown flag in any case
serverInventory.shutdown(false, -1, true);
serverInventory = null;
}
}
/** {@inheritDoc} */
@Override
public synchronized ServerInventory getValue() throws IllegalStateException, IllegalArgumentException {
final ServerInventory serverInventory = this.serverInventory;
if(serverInventory == null) {
throw new IllegalStateException();
}
return serverInventory;
}
InjectedValue getClient() {
return client;
}
private class FutureServerInventory extends AsyncFutureTask{
protected FutureServerInventory() {
super(null);
}
private void setInventory(ServerInventory inventory) {
super.setResult(inventory);
}
private void setFailure(final Throwable t) {
super.setFailed(t);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy