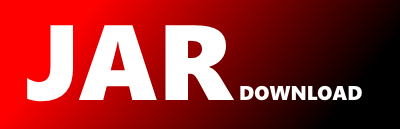
org.wisepersist.gradle.plugins.gwt.GwtTestExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-gradle-plugin Show documentation
Show all versions of gwt-gradle-plugin Show documentation
Gradle plugin to support GWT (http://www.gwtproject.org/) related tasks.
/**
* Copyright (C) 2013-2017 Steffen Schaefer
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.wisepersist.gradle.plugins.gwt;
import java.io.File;
import java.util.concurrent.Callable;
import org.gradle.api.internal.IConventionAware;
/**
* GWT specific extension for the Test task.
*/
public class GwtTestExtension extends GwtTestOptionsBase {
private File war;
private File deploy;
private File extra;
private File workDir;
private File gen;
private File cacheDir;
protected String getParameterString() {
final StringBuilder builder = new StringBuilder();
dirArgIfSet(builder, "-war", getWar());
dirArgIfSet(builder, "-deploy", getDeploy());
dirArgIfSet(builder, "-extra", getExtra());
dirArgIfSet(builder, "-workDir", getWorkDir());
dirArgIfSet(builder, "-gen", getGen());
argIfSet(builder, "-logLevel", getLogLevel());
argIfSet(builder, "-port",
Boolean.TRUE.equals(getAutoPort()) ? "auto" : getPort());
argIfSet(builder, "-whitelist", getWhitelist());
argIfSet(builder, "-blacklist", getBlacklist());
argIfSet(builder, "-logdir", getLogDir());
argIfSet(builder, "-codeServerPort",
Boolean.TRUE.equals(getAutoCodeServerPort()) ? "auto"
: getCodeServerPort());
argIfSet(builder, "-style", getStyle());
argIfEnabled(builder, getEa(), "-ea");
argIfEnabled(builder, getDisableClassMetadata(), "-XdisableClassMetadata");
argIfEnabled(builder, getDisableCastChecking(), "-XdisableCastChecking");
argIfEnabled(builder, getDraftCompile(), "-draftCompile");
argIfSet(builder, "-localWorkers", getLocalWorkers());
argIfEnabled(builder, getProd(), "-prod");
argIfSet(builder, "-testMethodTimeout", getTestMethodTimeout());
argIfSet(builder, "-testBeginTimeout", getTestBeginTimeout());
argIfSet(builder, "-runStyle", getRunStyle());
argIfEnabled(builder, getNotHeadless(), "-notHeadless");
argIfEnabled(builder, getStandardsMode(), "-standardsMode");
argIfEnabled(builder, getQuirksMode(), "-quirksMode");
argIfSet(builder, "-Xtries", getTries());
argIfSet(builder, "-userAgents", getUserAgents());
return builder.toString();
}
private void argIfEnabled(StringBuilder builder, Boolean condition,
String arg) {
if (Boolean.TRUE.equals(condition)) {
arg(builder, arg);
}
}
private void dirArgIfSet(StringBuilder builder, String arg, File dir) {
if (dir != null) {
dir.mkdirs();
arg(builder, arg, dir);
}
}
private void argIfSet(StringBuilder builder, String arg, Object value) {
if (value != null) {
arg(builder, arg, value);
}
}
private void arg(StringBuilder builder, Object... args) {
for (Object arg : args) {
if (builder.length() > 0) {
builder.append(' ');
}
builder.append(arg.toString());
}
}
protected void configure(final GwtPluginExtension extension,
final IConventionAware conventionAware) {
final GwtTestOptions testOptions = extension.getTest();
conventionAware.getConventionMapping().map("war",
(Callable) () -> extension.getDevWar());
conventionAware.getConventionMapping().map("extra",
(Callable) () -> extension.getExtraDir());
conventionAware.getConventionMapping().map("workDir",
(Callable) () -> extension.getWorkDir());
conventionAware.getConventionMapping().map("gen",
(Callable) () -> extension.getGenDir());
conventionAware.getConventionMapping().map("cacheDir",
(Callable) () -> extension.getCacheDir());
conventionAware.getConventionMapping().map("logLevel",
(Callable) () -> extension.getLogLevel());
conventionAware.getConventionMapping().map("port",
(Callable) () -> testOptions.getPort());
conventionAware.getConventionMapping().map("autoPort",
(Callable) () -> testOptions.getAutoPort());
conventionAware.getConventionMapping().map("whitelist",
(Callable) () -> testOptions.getWhitelist());
conventionAware.getConventionMapping().map("blacklist",
(Callable) () -> testOptions.getBlacklist());
conventionAware.getConventionMapping().map("logDir",
(Callable) () -> testOptions.getLogDir());
conventionAware.getConventionMapping().map("codeServerPort",
(Callable) () -> testOptions.getCodeServerPort());
conventionAware.getConventionMapping().map("autoCodeServerPort",
(Callable) () -> testOptions.getAutoCodeServerPort());
conventionAware.getConventionMapping().map("style",
(Callable