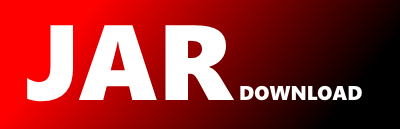
org.wisepersist.BaseEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wise-persist Show documentation
Show all versions of wise-persist Show documentation
WisePersist is a simple JPA wrapper which provides @Transactional and @NonTransactional annotations and pre-configured Guice module.
The newest version!
/*
* Copyright (c) 2014 WisePersist
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.wisepersist;
import com.google.common.base.Objects;
import java.io.Serializable;
import java.util.Date;
import javax.persistence.MappedSuperclass;
/**
* Abstract base class for entities.
*
* @param the type
*/
@MappedSuperclass
public abstract class BaseEntity> implements Serializable {
/**
* Utility method for equals()
methods.
*
* @param o1 one object
* @param o2 another object
* @return true
if they're both null
or both equal
*/
protected boolean areEqual(final Object o1, final Object o2) {
if (o1 == null) {
if (o2 != null) {
return false;
}
} else if (!o1.equals(o2)) {
return false;
}
return true;
}
/**
* Utility method for equals()
methods.
*
* @param s1 one string
* @param s2 another string
* @param ignoreCase if false
do case-sensitive comparison
* @return true
if they're both null
or both equal
*/
protected boolean areEqual(final String s1, final String s2, final boolean ignoreCase) {
// for use in custom equals() methods
if (s1 == null && s2 == null) {
return true;
}
if (s1 == null || s2 == null) {
return false;
}
return ignoreCase ? s1.equalsIgnoreCase(s2) : s1.equals(s2);
}
/**
* Utility method for equals()
methods.
*
* @param d1 one date
* @param d2 another date
* @return true
if they're both null
or both equal
*/
protected boolean areEqual(final Date d1, final Date d2) {
// for use in custom equals() methods
if (d1 == null && d2 == null) {
return true;
}
if (d1 == null || d2 == null) {
return false;
}
return d1.getTime() == d2.getTime();
}
/**
* Utility method for equals()
methods.
*
* @param f1 one float
* @param f2 another float
* @return true
if they're equal
*/
protected boolean areEqual(final float f1, final float f2) {
// for use in custom equals() methods
return Float.floatToIntBits(f1) == Float.floatToIntBits(f2);
}
/**
* Utility method for hashCode()
methods.
*
* @param values the values to use in calculation
* @return the hash code value
*/
protected int calculateHashCode(final Object... values) {
return Objects.hashCode(values);
}
/**
* {@inheritDoc}
*/
public final int hashCode() {
return calculateHashCode(getHashCodeData());
}
/**
* Get the data used to calculate hash code; use getters not fields in case the instance is a
* proxy.
*
* @return the data
*/
@SuppressWarnings("JpaAttributeTypeInspection")
protected abstract Object[] getHashCodeData();
/**
* Allows type-specific "this".
*
* @return this
*/
protected abstract T getThis();
/**
* Get the primary key.
*
* @return the pk, or null
if not set or if not supported
*/
public abstract Serializable getPk();
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public final boolean equals(final Object other) {
if (this == other) {
return true;
}
if (other == null || // looks into the target class of a proxy if necessary
!other.getClass().equals(this.getClass())) {
return false;
}
// if pks are both set, compare
if (getPk() != null) {
@SuppressWarnings("rawtypes")
Serializable otherPk = ((BaseEntity) other).getPk();
if (otherPk != null) {
return getPk().equals(otherPk);
}
}
return dataEquals((T) other);
}
/**
* Compare data only; null, class, and pk have been checked.
*
* @param other the other instance
* @return true
if equal
*/
protected abstract boolean dataEquals(T other);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy