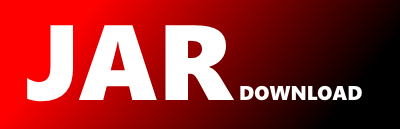
org.wiztools.commons.ArrayUtil Maven / Gradle / Ivy
/*
* Copyright WizTools.org
* Licensed under the Apache License, Version 2.0:
* http://www.apache.org/licenses/LICENSE-2.0
*/
package org.wiztools.commons;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author subwiz
*/
public final class ArrayUtil {
private ArrayUtil(){}
public static boolean contains(T[] array, T value) {
for(T v: array) {
if(v == null && value == null) return true;
if(v != null && v.equals(value)) return true;
}
return false;
}
/**
* Determines if the passed object is of type array.
* @param o The object to determine if it is an array.
* @return true if the passed object is an array.
* @throws NullPointerException when the passed object is null.
*/
public static boolean isArray(Object o) throws NullPointerException {
if(o == null)
throw new NullPointerException("Object is null: cannot determine if it is of array type.");
else {
return o.getClass().isArray();
}
}
/**
* Concatenates all the passed parameters.
* @param
* @param objs
* @return T[]
*/
public static T[] concat(T[] ... objs){
List out = new ArrayList();
int i = 0;
T[] pass = null;
for(T[] o: objs){
for(int j=0; j
* @param arr The input array of objects.
*/
public static void reverse(T[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final T tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(boolean[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final boolean tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(byte[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final byte tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(char[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final char tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(short[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final short tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(int[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final int tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(long[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final long tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(float[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final float tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
public static void reverse(double[] arr) {
for(int left=0, right=arr.length-1; left < right; left++, right--) {
final double tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy