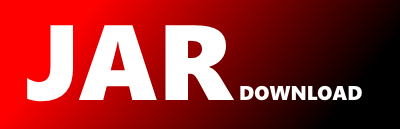
org.wiztools.commons.CollectionsUtil Maven / Gradle / Ivy
/*
* Copyright WizTools.org
* Licensed under the Apache License, Version 2.0:
* http://www.apache.org/licenses/LICENSE-2.0
*/
package org.wiztools.commons;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Set;
/**
*
* @author subwiz
*/
public final class CollectionsUtil {
public static final MultiValueMap EMPTY_MULTI_VALUE_MAP = new MultiValueMap() {
@Override
public void clear() {
throw new UnsupportedOperationException();
}
@Override
public boolean containsKey(Object key) {
return false;
}
@Override
public boolean containsValue(Object value) {
return false;
}
@Override
public Collection get(Object key) {
return null;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Set keySet() {
return Collections.EMPTY_SET;
}
@Override
public Collection put(Object key, Object value) {
throw new UnsupportedOperationException();
}
@Override
public Collection remove(Object key) {
throw new UnsupportedOperationException();
}
@Override
public int size() {
return 0;
}
@Override
public Collection values() {
return Collections.EMPTY_LIST;
}
@Override
public boolean equals(Object obj) {
if(obj == null){return false;}
if(!(obj instanceof MultiValueMap)){return false;}
MultiValueMap other = (MultiValueMap) obj;
return other.isEmpty();
}
@Override
public int hashCode() {
return super.hashCode();
}
};
/**
* Returns a empty MultiValueMap. Example usage:
*
* {@code
* MultiValueMap map = CollectionsUtil.emptyMultiValueMap();
* }
*
* @param
* @param
* @return Returns a empty MultiValueMap.
*/
public static final MultiValueMap emptyMultiValueMap() {
return (MultiValueMap) EMPTY_MULTI_VALUE_MAP;
}
/**
* Returns an unmodifiable version of the MultiValueMap.
* @param
* @param
* @param map
* @return Returns an unmodifiable version of the MultiValueMap.
*/
public static final MultiValueMap unmodifiableMultiValueMap(
final MultiValueMap map) {
if (map == null) {
throw new NullPointerException();
}
return new MultiValueMap() {
@Override
public void clear() {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public boolean containsKey(K key) {
return map.containsKey(key);
}
@Override
public boolean containsValue(V value) {
return map.containsValue(value);
}
@Override
public Collection get(K key) {
return Collections.unmodifiableCollection(map.get(key));
}
@Override
public boolean isEmpty() {
return map.isEmpty();
}
@Override
public Set keySet() {
return Collections.unmodifiableSet(map.keySet());
}
@Override
public Collection put(K key, V value) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Collection remove(K key) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public int size() {
return map.size();
}
@Override
public Collection values() {
Collection values = map.values();
if(values == null){
return null;
}
if(values instanceof List){
return Collections.unmodifiableList((List)values);
}
if(values instanceof Set){
return Collections.unmodifiableSet((Set)values);
}
// This does not override equals() and hashCode():
return Collections.unmodifiableCollection(values);
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (!(obj instanceof MultiValueMap)) {
return false;
}
final MultiValueMap other = (MultiValueMap) obj;
final Set mapKeySet = map.keySet();
final Set otherKeySet = other.keySet();
if (mapKeySet != otherKeySet && (mapKeySet == null || !mapKeySet.equals(otherKeySet))) {
return false;
}
final Collection mapValues = map.values();
final Collection otherValues = other.values();
if (mapValues != otherValues && (mapValues == null || !mapValues.equals(otherValues))) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 7;
hash = 47 * hash + (map != null ? map.hashCode() : 0);
return hash;
}
@Override
public String toString() {
return map.toString();
}
};
}
/**
* Accepts a varargs argument of type T, and returns a java.util.List representation of them.
* @param
* @param objs
* @return A List representation of all the varargs.
*/
public static List asList(T ... objs){
List l = new ArrayList<>();
l.addAll(Arrays.asList(objs));
return l;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy