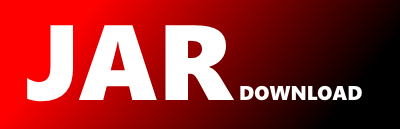
org.wowtools.dao.SqlUtil Maven / Gradle / Ivy
The newest version!
package org.wowtools.dao;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.persistence.EntityManager;
import javax.persistence.Query;
import java.sql.*;
import java.util.Collection;
import java.util.Date;
/**
* 直接用sql操作数据的工具类
*
* @author liuyu
*/
public class SqlUtil {
private static final Logger log = LoggerFactory.getLogger(SqlUtil.class);
/**
* ResultSet遍历器
*
* @author liuyu
*/
public static abstract class JdbcResultVisitor {
public void beforLoop(ResultSet rs) throws SQLException {
}
public abstract void visit(ResultSet rs) throws SQLException;
public void afterLoop(ResultSet rs) throws SQLException {
}
}
/**
* 简化的ResultSet遍历器,只有visit部分,以便使用labmbda表达式
*
* @author liuyu
*/
@FunctionalInterface
public interface SimpleJdbcResultVisitor {
void visit(ResultSet rs) throws SQLException;
}
private static String getLogSqlAndParams(String sql, Object[] paramValue) {
StringBuilder sb = new StringBuilder();
sb.append("SqlUtil executeUpdate:\t").append(sql);
sb.append("\nparams:");
for (Object p : paramValue) {
sb.append(p == null ? "null" : sb.toString()).append("\t");
}
return sb.toString();
}
/**
* 执行hql CUD命令
*
* @param sql sql
* @param paramValue 参数值
* @return the number of entities updated or deleted
*/
public static int executeUpdateByNativeQuery(EntityManager em, String sql, Object[] paramValue) {
if (log.isDebugEnabled()) {
log.debug(getLogSqlAndParams(sql, paramValue));
}
try {
Query query = em.createNativeQuery(sql);
int i = 1;
for (Object p : paramValue) {
query.setParameter(i, p);
i++;
}
return query.executeUpdate();
} catch (Exception e) {
log.debug(getLogSqlAndParams(sql, paramValue));
throw new RuntimeException("执行异常,sql:" + sql, e);
}
}
/**
* 执行sql CUD命令
*
* @param sql sql
* @param paramValue 参数值
* @return the number of entities updated or deleted
*/
public static int executeUpdate(Connection conn, String sql, Object... paramValue) {
return executeUpdate(conn, sql, true, paramValue);
}
/**
* 执行sql CUD命令
*
* @param sql sql
* @param paramValue 参数值
* @return the number of entities updated or deleted
*/
public static int executeUpdate(Connection conn, String sql, boolean closeConn, Object... paramValue) {
if (log.isDebugEnabled()) {
log.debug(getLogSqlAndParams(sql, paramValue));
}
PreparedStatement pstm = null;
try {
pstm = conn.prepareStatement(sql);
int i = 1;
for (Object arg : paramValue) {
pstm.setObject(i, toDbObj(arg));
i++;
}
return pstm.executeUpdate();
} catch (Exception e) {
String msg = e.getMessage();
if (msg.indexOf("ORA-00939") < 0) {
log.debug(getLogSqlAndParams(sql, paramValue));
throw new RuntimeException("执行异常,msg:" + e.getMessage() + ",sql:" + sql, e);
}
return 1;
} finally {
closePreparedStatement(pstm);
closeConnection(conn);
}
}
private static Object toDbObj(Object o) {
if (o instanceof Date) {
Date t = (Date) o;
return new Timestamp(t.getTime());
}
return o;
}
/**
* 批量执行CUD命令
*
* @param sql
* @param paramValues
* @return an array of update counts containing one element for each
* command in the batch. The elements of the array are ordered according
* to the order in which commands were added to the batch.
*/
public static int[] batchUpdate(Connection conn, String sql, Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy