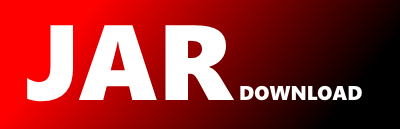
org.khelekore.prtree.Circle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of georewrite Show documentation
Show all versions of georewrite Show documentation
重写geoserver发送到h2的sql,以实现自定义数据源
package org.khelekore.prtree;
import java.util.ArrayList;
import java.util.List;
/** A simple circular data structure.
*
* @param the element type
*/
class Circle {
private final List data;
private int currentPos;
public Circle (int size) {
data = new ArrayList<> (size);
}
public void add (T t) {
data.add (t);
}
public T get (int pos) {
pos %= data.size ();
return data.get (pos);
}
public int getNumElements () {
return data.size ();
}
public void reset () {
currentPos = 0;
}
public T getNext () {
T ret = data.get (currentPos++);
currentPos %= data.size ();
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy