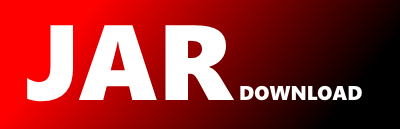
org.khelekore.prtree.Node Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of georewrite Show documentation
Show all versions of georewrite Show documentation
重写geoserver发送到h2的sql,以实现自定义数据源
package org.khelekore.prtree;
import java.util.List;
import java.util.PriorityQueue;
/** A node in a Priority R-Tree
* @param the data type of the elements stored in this node
*/
interface Node {
/** Get the size of the node, that is how many data elements it holds
* @return the number of elements (leafs or child nodes) that this node has
*/
int size ();
/** Get the MBR of this node
* @param converter the MBR converter to use for the actual objects
* @return the MBR for this node
*/
MBR getMBR (MBRConverter converter);
/** Visit this node and add the leafs to the found list and add
* any child nodes to the list of nodes to expand.
* @param mbr the query rectangle
* @param filter a secondary filter to apply to the expanded nodes
* @param converter the MBR converter to use for the actual objects
* @param found the List of leaf nodes
* @param nodesToExpand the List of nodes that needs to be expanded
*/
void expand (MBR mbr, NodeFilter filter, MBRConverter converter,
List found, List> nodesToExpand);
/** Find nodes that intersect with the given MBR.
* @param mbr the query rectangle
* @param converter the MBR converter to use for the actual objects
* @param result the List to add the found nodes to
* @param filter a secondary filter to apply to the expanded nodes
*/
void find (MBR mbr, MBRConverter converter,
List result, NodeFilter filter);
/** Expand the nearest neighbour search
* @param dc the DistanceCalculator to use when calculating distances
* @param filter the NodeFilter to use when getting node objects
* @param currentFinds the currently found results, this list will
* be populated
* @param maxHits the maximum number of entries to store
* @param queue where to store child nodes that needs expansion
* @param mdc the MinDistComparator to use
*/
void nnExpand (DistanceCalculator dc,
NodeFilter filter,
List> currentFinds,
int maxHits,
PriorityQueue> queue,
MinDistComparator> mdc);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy