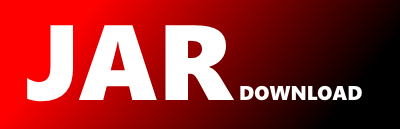
org.wso2.carbon.humantask.impl.TPresentationElementsImpl Maven / Gradle / Ivy
/*
* XML Type: tPresentationElements
* Namespace: http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803
* Java type: org.wso2.carbon.humantask.TPresentationElements
*
* Automatically generated - do not modify.
*/
package org.wso2.carbon.humantask.impl;
/**
* An XML tPresentationElements(@http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803).
*
* This is a complex type.
*/
public class TPresentationElementsImpl extends org.wso2.carbon.humantask.impl.TExtensibleElementsImpl implements org.wso2.carbon.humantask.TPresentationElements
{
private static final long serialVersionUID = 1L;
public TPresentationElementsImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName NAME$0 =
new javax.xml.namespace.QName("http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803", "name");
private static final javax.xml.namespace.QName PRESENTATIONPARAMETERS$2 =
new javax.xml.namespace.QName("http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803", "presentationParameters");
private static final javax.xml.namespace.QName SUBJECT$4 =
new javax.xml.namespace.QName("http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803", "subject");
private static final javax.xml.namespace.QName DESCRIPTION$6 =
new javax.xml.namespace.QName("http://docs.oasis-open.org/ns/bpel4people/ws-humantask/200803", "description");
/**
* Gets array of all "name" elements
*/
public org.wso2.carbon.humantask.TText[] getNameArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(NAME$0, targetList);
org.wso2.carbon.humantask.TText[] result = new org.wso2.carbon.humantask.TText[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "name" element
*/
public org.wso2.carbon.humantask.TText getNameArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().find_element_user(NAME$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "name" element
*/
public int sizeOfNameArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(NAME$0);
}
}
/**
* Sets array of all "name" element
*/
public void setNameArray(org.wso2.carbon.humantask.TText[] nameArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(nameArray, NAME$0);
}
}
/**
* Sets ith "name" element
*/
public void setNameArray(int i, org.wso2.carbon.humantask.TText name)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().find_element_user(NAME$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(name);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "name" element
*/
public org.wso2.carbon.humantask.TText insertNewName(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().insert_element_user(NAME$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "name" element
*/
public org.wso2.carbon.humantask.TText addNewName()
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().add_element_user(NAME$0);
return target;
}
}
/**
* Removes the ith "name" element
*/
public void removeName(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(NAME$0, i);
}
}
/**
* Gets the "presentationParameters" element
*/
public org.wso2.carbon.humantask.TPresentationParameters getPresentationParameters()
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TPresentationParameters target = null;
target = (org.wso2.carbon.humantask.TPresentationParameters)get_store().find_element_user(PRESENTATIONPARAMETERS$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "presentationParameters" element
*/
public boolean isSetPresentationParameters()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(PRESENTATIONPARAMETERS$2) != 0;
}
}
/**
* Sets the "presentationParameters" element
*/
public void setPresentationParameters(org.wso2.carbon.humantask.TPresentationParameters presentationParameters)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TPresentationParameters target = null;
target = (org.wso2.carbon.humantask.TPresentationParameters)get_store().find_element_user(PRESENTATIONPARAMETERS$2, 0);
if (target == null)
{
target = (org.wso2.carbon.humantask.TPresentationParameters)get_store().add_element_user(PRESENTATIONPARAMETERS$2);
}
target.set(presentationParameters);
}
}
/**
* Appends and returns a new empty "presentationParameters" element
*/
public org.wso2.carbon.humantask.TPresentationParameters addNewPresentationParameters()
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TPresentationParameters target = null;
target = (org.wso2.carbon.humantask.TPresentationParameters)get_store().add_element_user(PRESENTATIONPARAMETERS$2);
return target;
}
}
/**
* Unsets the "presentationParameters" element
*/
public void unsetPresentationParameters()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(PRESENTATIONPARAMETERS$2, 0);
}
}
/**
* Gets array of all "subject" elements
*/
public org.wso2.carbon.humantask.TText[] getSubjectArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(SUBJECT$4, targetList);
org.wso2.carbon.humantask.TText[] result = new org.wso2.carbon.humantask.TText[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "subject" element
*/
public org.wso2.carbon.humantask.TText getSubjectArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().find_element_user(SUBJECT$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "subject" element
*/
public int sizeOfSubjectArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(SUBJECT$4);
}
}
/**
* Sets array of all "subject" element
*/
public void setSubjectArray(org.wso2.carbon.humantask.TText[] subjectArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(subjectArray, SUBJECT$4);
}
}
/**
* Sets ith "subject" element
*/
public void setSubjectArray(int i, org.wso2.carbon.humantask.TText subject)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().find_element_user(SUBJECT$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(subject);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "subject" element
*/
public org.wso2.carbon.humantask.TText insertNewSubject(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().insert_element_user(SUBJECT$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "subject" element
*/
public org.wso2.carbon.humantask.TText addNewSubject()
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TText target = null;
target = (org.wso2.carbon.humantask.TText)get_store().add_element_user(SUBJECT$4);
return target;
}
}
/**
* Removes the ith "subject" element
*/
public void removeSubject(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(SUBJECT$4, i);
}
}
/**
* Gets array of all "description" elements
*/
public org.wso2.carbon.humantask.TDescription[] getDescriptionArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DESCRIPTION$6, targetList);
org.wso2.carbon.humantask.TDescription[] result = new org.wso2.carbon.humantask.TDescription[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "description" element
*/
public org.wso2.carbon.humantask.TDescription getDescriptionArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TDescription target = null;
target = (org.wso2.carbon.humantask.TDescription)get_store().find_element_user(DESCRIPTION$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "description" element
*/
public int sizeOfDescriptionArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DESCRIPTION$6);
}
}
/**
* Sets array of all "description" element
*/
public void setDescriptionArray(org.wso2.carbon.humantask.TDescription[] descriptionArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(descriptionArray, DESCRIPTION$6);
}
}
/**
* Sets ith "description" element
*/
public void setDescriptionArray(int i, org.wso2.carbon.humantask.TDescription description)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TDescription target = null;
target = (org.wso2.carbon.humantask.TDescription)get_store().find_element_user(DESCRIPTION$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(description);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "description" element
*/
public org.wso2.carbon.humantask.TDescription insertNewDescription(int i)
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TDescription target = null;
target = (org.wso2.carbon.humantask.TDescription)get_store().insert_element_user(DESCRIPTION$6, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "description" element
*/
public org.wso2.carbon.humantask.TDescription addNewDescription()
{
synchronized (monitor())
{
check_orphaned();
org.wso2.carbon.humantask.TDescription target = null;
target = (org.wso2.carbon.humantask.TDescription)get_store().add_element_user(DESCRIPTION$6);
return target;
}
}
/**
* Removes the ith "description" element
*/
public void removeDescription(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DESCRIPTION$6, i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy