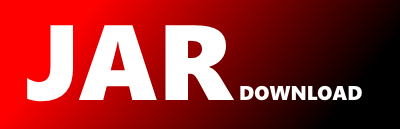
org.wso2.carbon.databridge.commons.StreamDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.wso2.carbon.databridge.commons Show documentation
Show all versions of org.wso2.carbon.databridge.commons Show documentation
org.wso2.carbon.agent.commons.thrift is the common thrift objects used in Agent and
Agent Server communication
The newest version!
/**
*
* Copyright (c) 2005-2010, WSO2 Inc. (http://www.wso2.org) All Rights Reserved.
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.wso2.carbon.databridge.commons;
import org.wso2.carbon.databridge.commons.exception.MalformedStreamDefinitionException;
import org.wso2.carbon.databridge.commons.utils.DataBridgeCommonsUtils;
import org.wso2.carbon.databridge.commons.utils.EventDefinitionConverterUtils;
import java.util.ArrayList;
import java.util.List;
public class StreamDefinition {
private String streamId;
private String name;
private String version = "1.0.0";
private String nickName;
private String description;
private List tags;
private List metaData;
private List correlationData;
private List payloadData;
private IndexDefinition indexDefinition;
@Deprecated
/**
* @deprecated As of release 4.0.7 as streamId will ba always generated by as -
*/
public StreamDefinition(String name, String version, String streamId)
throws MalformedStreamDefinitionException {
this(name, version);
}
public StreamDefinition(String name, String version)
throws MalformedStreamDefinitionException {
if (name.contains(DataBridgeCommonsUtils.STREAM_NAME_VERSION_SPLITTER)) {
throw new MalformedStreamDefinitionException(EBCommonsConstants.NAME + " " + name + " cannot contain '-' ");
}
this.name = name;
if (version.contains(DataBridgeCommonsUtils.STREAM_NAME_VERSION_SPLITTER)) {
throw new MalformedStreamDefinitionException(EBCommonsConstants.VERSION + " " + version + " cannot contain '-' ");
}
String versionPattern = "^\\d+\\.\\d+\\.\\d+$";
if (!version.matches(versionPattern)) {
throw new MalformedStreamDefinitionException(
EBCommonsConstants.VERSION + " " + version + " does not adhere to the format x.x.x ");
}
this.version = version;
generateSteamId();
}
public StreamDefinition(String name) {
this.name = name;
generateSteamId();
}
private void generateSteamId() {
if (streamId == null) {
this.streamId = DataBridgeCommonsUtils.generateStreamId(name, version);
}
}
public void setNickName(String nickName) {
this.nickName = nickName;
}
public void setDescription(String description) {
this.description = description;
}
public void setTags(List tags) {
this.tags = tags;
}
public void setMetaData(List metaData) {
this.metaData = metaData;
}
public void setCorrelationData(List correlationData) {
this.correlationData = correlationData;
}
public void setPayloadData(List payloadData) {
this.payloadData = payloadData;
}
public String getStreamId() {
return streamId;
}
public String getName() {
return name;
}
public String getVersion() {
return version;
}
public String getNickName() {
return nickName;
}
public String getDescription() {
return description;
}
public List getTags() {
return tags;
}
public List getMetaData() {
return metaData;
}
public List getCorrelationData() {
return correlationData;
}
public List getPayloadData() {
return payloadData;
}
public List getAttributeListForKey(String key) {
if (key.equals("metaData")) {
return metaData;
} else if (key.equals("correlationData")) {
return correlationData;
} else if (key.equals("payloadData")) {
return payloadData;
}
return null;
}
/**
* Stream Id is not used for comparing definitions
* This is because this method is used to identify duplicates
*
* @param o compared Object
* @return true if equal else false
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StreamDefinition that = (StreamDefinition) o;
if (correlationData != null ? !correlationData.equals(that.correlationData) : that.correlationData != null) {
return false;
}
if (metaData != null ? !metaData.equals(that.metaData) : that.metaData != null) {
return false;
}
if (payloadData != null ? !payloadData.equals(that.payloadData) : that.payloadData != null) {
return false;
}
if (!name.equals(that.name)) {
return false;
}
if (!version.equals(that.version)) {
return false;
}
return true;
}
/**
* Stream Id is not used for comparing definitions
* This is because this method is used to identify duplicates
*
* @return hash code
*/
@Override
public int hashCode() {
int result = name.hashCode();
result = 31 * result + version.hashCode();
result = 31 * result + (metaData != null ? metaData.hashCode() : 0);
result = 31 * result + (correlationData != null ? correlationData.hashCode() : 0);
result = 31 * result + (payloadData != null ? payloadData.hashCode() : 0);
return result;
}
public void addTag(String tag) {
if (tags == null) {
tags = new ArrayList();
}
tags.add(tag);
}
public void addMetaData(String name, AttributeType type) {
if (metaData == null) {
metaData = new ArrayList();
}
metaData.add(new Attribute(name, type));
}
public void addCorrelationData(String name, AttributeType type) {
if (correlationData == null) {
correlationData = new ArrayList();
}
correlationData.add(new Attribute(name, type));
}
public void addPayloadData(String name, AttributeType type) {
if (payloadData == null) {
payloadData = new ArrayList();
}
payloadData.add(new Attribute(name, type));
}
public IndexDefinition getIndexDefinition() {
return indexDefinition;
}
public void setIndexDefinition(IndexDefinition indexDefinition) {
this.indexDefinition = indexDefinition;
}
public void createIndexDefinition(String indexDefinition) {
this.indexDefinition = new IndexDefinition();
this.indexDefinition.setIndexData(indexDefinition, this);
}
public void createIndexDefinitionFromStore(String indexDefinition) {
this.indexDefinition = new IndexDefinition();
this.indexDefinition.setIndexDataFromStore(indexDefinition);
}
@Override
public String toString() {
return EventDefinitionConverterUtils.convertToBasicJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy