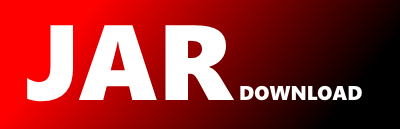
web.oauth.edit.jsp Maven / Gradle / Ivy
<%@ page import="org.apache.axis2.context.ConfigurationContext" %>
<%@ page import="org.apache.commons.lang.StringUtils" %>
<%@ page import="org.owasp.encoder.Encode" %>
<%@ page import="org.wso2.carbon.CarbonConstants" %>
<%@ page import="org.wso2.carbon.identity.oauth.common.OAuthConstants" %>
<%@ page import="org.wso2.carbon.identity.oauth.stub.dto.OAuthConsumerAppDTO" %>
<%@ page import="org.wso2.carbon.identity.oauth.stub.dto.TokenBindingMetaDataDTO" %>
<%@ page import="org.wso2.carbon.identity.oauth.ui.client.OAuthAdminClient" %>
<%@ page import="org.wso2.carbon.identity.oauth.ui.util.OAuthUIUtil" %>
<%@ page import="org.wso2.carbon.ui.CarbonUIMessage" %>
<%@ page import="org.wso2.carbon.ui.CarbonUIUtil" %>
<%@ page import="org.wso2.carbon.utils.ServerConstants" %>
<%@ page import="java.util.ArrayList" %>
<%@ page import="java.util.Arrays" %>
<%@ page import="java.util.Collections" %>
<%@ page import="java.util.List" %>
<%@ page import="java.util.ResourceBundle" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%@ taglib uri="http://wso2.org/projects/carbon/taglibs/carbontags.jar" prefix="carbon" %>
<%
boolean isHashDisabled = false;
String consumerkey = request.getParameter("consumerkey");
String appName = request.getParameter("appName");
OAuthConsumerAppDTO app = null;
String forwardTo = null;
String BUNDLE = "org.wso2.carbon.identity.oauth.ui.i18n.Resources";
String DEFAULT_TOKEN_TYPE = "default";
ResourceBundle resourceBundle = ResourceBundle.getBundle(BUNDLE, request.getLocale());
String id = null;
String secret = null;
// grants
boolean codeGrant = false;
boolean implicitGrant = false;
List allowedGrants = null;
String applicationSPName = null;
OAuthAdminClient client = null;
String action = null;
List grants = null;
String[] audiences = null;
String audienceTableStyle = "display:none";
List allowedScopeValidators = new ArrayList();
List scopeValidators = new ArrayList();
List tokenTypes = new ArrayList();
String[] supportedIdTokenEncryptionAlgorithms = null;
String[] supportedIdTokenEncryptionMethods = null;
String logoutUrl = null;
boolean isBackchannelLogoutEnabled = false;
boolean isFrontchannelLogoutEnabled = false;
boolean isRenewRefreshTokenEnabled = true;
TokenBindingMetaDataDTO[] supportedTokenBindingsMetaData = new TokenBindingMetaDataDTO[0];
try {
applicationSPName = request.getParameter("appName");
action = request.getParameter("action");
String cookie = (String) session.getAttribute(ServerConstants.ADMIN_SERVICE_COOKIE);
String backendServerURL = CarbonUIUtil.getServerURL(config.getServletContext(), session);
ConfigurationContext configContext =
(ConfigurationContext) config.getServletContext()
.getAttribute(CarbonConstants.CONFIGURATION_CONTEXT);
client = new OAuthAdminClient(cookie, backendServerURL, configContext);
isHashDisabled = client.isHashDisabled();
supportedIdTokenEncryptionAlgorithms =
client.getSupportedIDTokenAlgorithms().getSupportedIdTokenEncryptionAlgorithms();
supportedIdTokenEncryptionMethods =
client.getSupportedIDTokenAlgorithms().getSupportedIdTokenEncryptionMethods();
supportedTokenBindingsMetaData = client.getSupportedTokenBindingsMetaData();
if (consumerkey != null) {
app = client.getOAuthApplicationData(consumerkey);
} else {
app = client.getOAuthApplicationDataByAppName(appName);
}
OAuthConsumerAppDTO consumerApp = null;
if (OAuthConstants.ACTION_REGENERATE.equalsIgnoreCase(action)) {
String oauthAppState = client.getOauthApplicationState(consumerkey);
if (isHashDisabled) {
client.regenerateSecretKey(consumerkey);
} else {
consumerApp = client.regenerateAndRetrieveOauthSecretKey(consumerkey);
}
if (OAuthConstants.OauthAppStates.APP_STATE_REVOKED.equalsIgnoreCase(oauthAppState)) {
client.updateOauthApplicationState(consumerkey, OAuthConstants.OauthAppStates.APP_STATE_ACTIVE);
}
if (isHashDisabled) {
app.setOauthConsumerSecret(client.getOAuthApplicationData(consumerkey).getOauthConsumerSecret());
} else {
app.setOauthConsumerSecret(consumerApp.getOauthConsumerSecret());
}
CarbonUIMessage.sendCarbonUIMessage("Client Secret successfully updated for Client ID: " + consumerkey,
CarbonUIMessage.INFO, request);
} else if (OAuthConstants.ACTION_REVOKE.equalsIgnoreCase(action)) {
String oauthAppState = client.getOauthApplicationState(consumerkey);
if (OAuthConstants.OauthAppStates.APP_STATE_REVOKED.equalsIgnoreCase(oauthAppState)) {
CarbonUIMessage.sendCarbonUIMessage("Application is already revoked.",
CarbonUIMessage.INFO, request);
} else {
client.updateOauthApplicationState(consumerkey, OAuthConstants.OauthAppStates.APP_STATE_REVOKED);
CarbonUIMessage.sendCarbonUIMessage("Application successfully revoked.", CarbonUIMessage.INFO, request);
}
} else {
if (app.getCallbackUrl() == null) {
app.setCallbackUrl("");
}
if (app.getBackChannelLogoutUrl() == null) {
app.setBackChannelLogoutUrl("");
}
if (app.getFrontchannelLogoutUrl() == null) {
app.setFrontchannelLogoutUrl("");
}
if (app.getBackChannelLogoutUrl() != "") {
logoutUrl = app.getBackChannelLogoutUrl();
isBackchannelLogoutEnabled = true;
} else if (app.getFrontchannelLogoutUrl() != "") {
logoutUrl = app.getFrontchannelLogoutUrl();
isFrontchannelLogoutEnabled = true;
} else {
logoutUrl = "";
}
allowedGrants = new ArrayList(Arrays.asList(client.getAllowedOAuthGrantTypes()));
allowedScopeValidators = new ArrayList(Arrays.asList(client.getAllowedScopeValidators()));
// Sorting the list to display the scope validators in alphabetical order
Collections.sort(allowedScopeValidators);
tokenTypes = new ArrayList(Arrays.asList(client.getSupportedTokenTypes()));
if (app.getRenewRefreshTokenEnabled() == null) {
isRenewRefreshTokenEnabled = client.isRefreshTokenRenewalEnabled();
} else {
isRenewRefreshTokenEnabled = Boolean.parseBoolean(app.getRenewRefreshTokenEnabled());
}
if (OAuthConstants.OAuthVersions.VERSION_2.equals(app.getOAuthVersion())) {
id = resourceBundle.getString("consumerkey.oauth20");
secret = resourceBundle.getString("consumersecret.oauth20");
} else {
id = resourceBundle.getString("consumerkey.oauth10a");
secret = resourceBundle.getString("consumersecret.oauth10a");
}
// setting grants if oauth version 2.0
if (OAuthConstants.OAuthVersions.VERSION_2.equals(app.getOAuthVersion())) {
String appGrantTypes = app.getGrantTypes();
if (appGrantTypes != null) {
grants = new ArrayList(Arrays.asList(appGrantTypes.split(" ")));
}
if (grants != null) {
codeGrant = grants.contains("authorization_code");
implicitGrant = grants.contains("implicit");
} else {
grants = new ArrayList();
}
audiences = app.getAudiences();
String[] val = app.getScopeValidators();
if (val != null) {
scopeValidators = Arrays.asList(val);
}
} else {
grants = new ArrayList();
}
}
} catch (Exception e) {
String message = resourceBundle.getString("error.while.loading.user.application.data");
CarbonUIMessage.sendCarbonUIMessage(message, CarbonUIMessage.ERROR, request);
forwardTo = "../admin/error.jsp";
%>
<%
}
if ((action != null) && ("revoke".equalsIgnoreCase(action) || "regenerate".equalsIgnoreCase(action))) {
session.setAttribute("oauth-consum-secret", app.getOauthConsumerSecret());
%>
<% } else {
%>
<%
}
%>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy