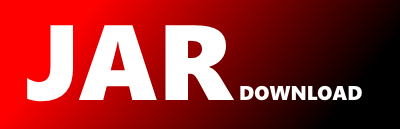
web.inbound.saveInbound.jsp Maven / Gradle / Ivy
The newest version!
<%@page import="org.wso2.carbon.inbound.ui.internal.ParamDTO" %>
<%@page import="java.util.Map" %>
<%@page import="java.util.ArrayList" %>
<%@page import="java.util.List" %>
<%@page import="java.lang.Long" %>
<%@page import="org.wso2.carbon.inbound.ui.internal.InboundManagementClient" %>
<%@page import="org.wso2.carbon.inbound.ui.internal.InboundClientConstants" %>
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1" %>
<%@ page import="org.owasp.encoder.Encode" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%@ taglib uri="http://wso2.org/projects/carbon/taglibs/carbontags.jar"
prefix="carbon" %>
<%
try {
InboundManagementClient client = InboundManagementClient.getInstance(config, session);
String classImpl = null;
String protocol = null;
if ((request.getParameter("inboundClass") == null || request.getParameter("inboundClass").equals(""))) {
protocol = Encode.forHtml(request.getParameter("inboundType"));
} else {
classImpl = request.getParameter("inboundClass");
}
List sParams = new ArrayList();
Map paramMap = request.getParameterMap();
for (String strKey : paramMap.keySet()) {
if (strKey.startsWith("transport.") || strKey.startsWith("java.naming.") || strKey.startsWith("inbound.") || strKey.startsWith("api.") || strKey.startsWith("dispatch.filter.")) {
String strVal = request.getParameter(strKey);
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, request.getParameter(strKey)));
}
} else if (strKey.startsWith("paramkey")) {
String paramKey = request.getParameter("paramkey" + strKey.replaceAll("paramkey", ""));
if (paramKey != null && !paramKey.trim().equals("")) {
sParams.add((new ParamDTO(paramKey, request.getParameter("paramval" + strKey.replaceAll("paramkey", "")))));
}
} else if (strKey.startsWith("inbound.behavior")) {
sParams.add((new ParamDTO("inbound.behavior", request.getParameter("inbound.behavior"))));
} else if (strKey.startsWith("interval")) {
sParams.add((new ParamDTO("interval", request.getParameter("interval"))));
} else if (strKey.startsWith("sequential")) {
sParams.add((new ParamDTO("sequential", request.getParameter("sequential"))));
} else if (strKey.startsWith("keystore")) {
sParams.add((new ParamDTO("keystore", request.getParameter(strKey))));
} else if (strKey.startsWith("truststore")) {
sParams.add((new ParamDTO("truststore", request.getParameter(strKey))));
} else if (strKey.startsWith("SSLVerifyClient")) {
sParams.add((new ParamDTO("SSLVerifyClient", request.getParameter(strKey))));
} else if (strKey.startsWith("HttpsProtocols")) {
sParams.add((new ParamDTO("HttpsProtocols", request.getParameter(strKey))));
} else if (strKey.startsWith("SSLProtocol")) {
sParams.add((new ParamDTO("SSLProtocol", request.getParameter(strKey))));
} else if (strKey.startsWith("CertificateRevocationVerifier")) {
sParams.add((new ParamDTO("CertificateRevocationVerifier", request.getParameter(strKey))));
} else if (strKey.startsWith("enableSSL")) {
sParams.add((new ParamDTO("enableSSL", request.getParameter(strKey))));
} else if (strKey.startsWith("coordination")) {
sParams.add((new ParamDTO("coordination", request.getParameter("coordination"))));
} else if (strKey.startsWith("pinnedServers")) {
sParams.add(new ParamDTO("pinnedServers", request.getParameter("pinnedServers")));
} else if (strKey.startsWith("waitTimeBeforeRead")) {
sParams.add(new ParamDTO("waitTimeBeforeRead", request.getParameter("waitTimeBeforeRead")));
} else if (strKey.startsWith("db_url")) {
sParams.add(new ParamDTO("db_url", request.getParameter("db_url")));
} else if (strKey.startsWith("concurrent.consumers")) {
sParams.add(new ParamDTO("concurrent.consumers", request.getParameter("concurrent.consumers")));
} else if (strKey.startsWith("PreferredCiphers")) {
sParams.add(new ParamDTO("PreferredCiphers", request.getParameter("PreferredCiphers")));
} else if (strKey.startsWith("zookeeper.") || strKey.startsWith("group.id") ||
strKey.startsWith("auto.") || strKey.startsWith("topic.filter") || strKey.equals("topics") || strKey.startsWith("filter.from") || strKey.startsWith("consumer.type")
|| strKey.startsWith("thread.count") || strKey.startsWith("simple.") || strKey.startsWith("content.type") || strKey.startsWith("offsets.") || strKey.startsWith("socket.") || strKey.startsWith("fetch.")
|| strKey.startsWith("consumer.") || strKey.startsWith("num.consumer.fetchers") || strKey.startsWith("queued.max.message.chunks") || strKey.startsWith("rebalance.") || strKey.startsWith("exclude.internal.topics")
|| strKey.startsWith("partition.assignment.strategy") || strKey.startsWith("client.id") || strKey.startsWith("dual.commit.enabled")) {
String strVal = request.getParameter(strKey).trim();
if (strKey.trim().equals("filter.from")) {
strKey = request.getParameter(strKey);
strVal = "true";
}
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, strVal));
}
} else if (strKey.startsWith("mqtt.") || strKey.startsWith("content.type")) {
String strVal = request.getParameter(strKey);
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, request.getParameter(strKey)));
}
} else if (strKey.startsWith("rabbitmq.")) {
String strVal = request.getParameter(strKey);
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, request.getParameter(strKey)));
}
} else if (strKey.startsWith("ws.")) {
String strVal = request.getParameter(strKey);
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, request.getParameter(strKey)));
}
} else if (strKey.startsWith("wss.")) {
String strVal = request.getParameter(strKey);
if (strVal != null && !strVal.equals("")) {
sParams.add(new ParamDTO(strKey, request.getParameter(strKey)));
}
} else if (strKey.startsWith("wso2mb.")) {
String strVal = request.getParameter(strKey);
if (strVal == null || strVal.equals("")) {
continue;
}
String factoryType = request.getParameter("transport.jms.ConnectionFactoryType");
String keyString = "";
if (factoryType.equalsIgnoreCase("topic")) {
keyString = "connectionfactory.TopicConnectionFactory";
} else {
keyString = "connectionfactory.QueueConnectionFactory";
}
sParams.add(new ParamDTO(keyString, strVal));
}
}
boolean added = client.addInboundEndpoint(Encode.forHtml(request.getParameter("inboundName")), request.getParameter("inboundSequence"), request.getParameter("inboundErrorSequence"), protocol, classImpl, request.getParameter("inboundSuspend"), sParams);
if (!added) {
%>
<%
} else {
%>
<% } %>
<%
} catch (Exception e) {
%>
<%
}
%>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy