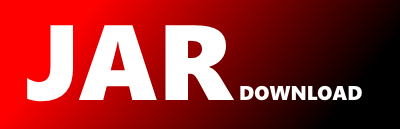
org.xbib.helianthus.common.AbstractRpcRequest Maven / Gradle / Ivy
package org.xbib.helianthus.common;
import static java.util.Objects.requireNonNull;
import java.util.List;
public class AbstractRpcRequest implements RpcRequest {
private final Class> serviceType;
private final String method;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy