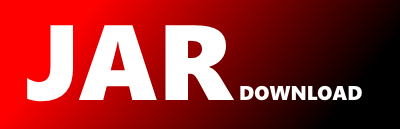
org.xbib.helianthus.common.http.HttpMessageAggregator Maven / Gradle / Ivy
package org.xbib.helianthus.common.http;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Subscription;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CompletableFuture;
abstract class HttpMessageAggregator implements Subscriber {
private final List contentList = new ArrayList<>();
private final CompletableFuture future;
private int contentLength;
private Subscription subscription;
protected HttpMessageAggregator(CompletableFuture future) {
this.future = future;
}
protected final CompletableFuture future() {
return future;
}
@Override
public void onSubscribe(Subscription s) {
subscription = s;
s.request(Long.MAX_VALUE);
}
protected final void add(HttpData data) {
final int dataLength = data.length();
if (dataLength > 0) {
if (contentLength > Integer.MAX_VALUE - dataLength) {
clear();
subscription.cancel();
throw new IllegalStateException("content length greater than Integer.MAX_VALUE");
}
contentList.add(data);
contentLength += dataLength;
}
}
protected final void clear() {
doClear();
contentList.clear();
}
protected void doClear() {
}
protected final HttpData finish() {
final HttpData content;
if (contentLength == 0) {
content = HttpData.EMPTY_DATA;
} else {
final byte[] merged = new byte[contentLength];
for (int i = 0, offset = 0; i < contentList.size(); i++) {
final HttpData data = contentList.set(i, null);
final int dataLength = data.length();
System.arraycopy(data.array(), data.offset(), merged, offset, dataLength);
offset += dataLength;
}
content = HttpData.of(merged);
}
return content;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy