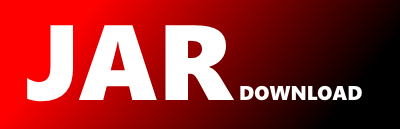
org.xbib.helianthus.common.stream.StreamWriter Maven / Gradle / Ivy
package org.xbib.helianthus.common.stream;
import org.reactivestreams.Subscriber;
import java.util.concurrent.CompletableFuture;
import java.util.function.Supplier;
/**
* Produces the objects to be published by a {@link StreamMessage}.
*
* @param the type of the stream element
*/
public interface StreamWriter {
/**
* Returns {@code true} if the {@link StreamMessage} is open.
*/
boolean isOpen();
/**
* Writes the specified object to the {@link StreamMessage}. The written object will be transferred to the
* {@link Subscriber}.
*/
boolean write(T o);
/**
* Writes the specified object {@link Supplier} to the {@link StreamMessage}. The object provided by the
* {@link Supplier} will be transferred to the {@link Subscriber}.
*/
boolean write(Supplier extends T> o);
/**
* Performs the specified {@code task} when there's enough demans from the {@link Subscriber}.
*
* @return the future that completes successfully when the {@code task} finishes or
* exceptionally when the {@link StreamMessage} is closed unexpectedly.
*/
CompletableFuture onDemand(Runnable task);
/**
* Closes the {@link StreamMessage} successfully. {@link Subscriber#onComplete()} will be invoked to
* signal that the {@link Subscriber} has consumed the stream completely.
*/
void close();
/**
* Closes the {@link StreamMessage} exceptionally. {@link Subscriber#onError(Throwable)} will be invoked to
* signal that the {@link Subscriber} did not consume the stream completely.
*/
void close(Throwable cause);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy