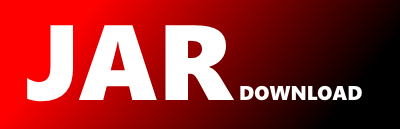
org.xbib.helianthus.internal.http.HttpObjectEncoder Maven / Gradle / Ivy
package org.xbib.helianthus.internal.http;
import io.netty.buffer.ByteBuf;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.http2.Http2Error;
import org.xbib.helianthus.common.ClosedSessionException;
import org.xbib.helianthus.common.http.HttpData;
import org.xbib.helianthus.common.http.HttpHeaders;
import org.xbib.helianthus.common.http.HttpObject;
/**
* Converts an {@link HttpObject} into a protocol-specific object and writes it into a {@link Channel}.
*/
public abstract class HttpObjectEncoder {
private volatile boolean closed;
private static ChannelFuture newFailedFuture(ChannelHandlerContext ctx) {
return ctx.newFailedFuture(ClosedSessionException.get());
}
protected static ByteBuf toByteBuf(ChannelHandlerContext ctx, HttpData data) {
final ByteBuf buf = ctx.alloc().directBuffer(data.length(), data.length());
buf.writeBytes(data.array(), data.offset(), data.length());
return buf;
}
/**
* Writes an {@link HttpHeaders}.
*/
public final ChannelFuture writeHeaders(ChannelHandlerContext ctx, int id, int streamId, HttpHeaders headers, boolean endStream) {
if (!ctx.channel().eventLoop().inEventLoop()) {
throw new IllegalStateException();
}
if (closed) {
return newFailedFuture(ctx);
}
return doWriteHeaders(ctx, id, streamId, headers, endStream);
}
protected abstract ChannelFuture doWriteHeaders(ChannelHandlerContext ctx, int id, int streamId, HttpHeaders headers, boolean endStream);
/**
* Writes an {@link HttpData}.
*/
public final ChannelFuture writeData(ChannelHandlerContext ctx, int id, int streamId, HttpData data, boolean endStream) {
if (!ctx.channel().eventLoop().inEventLoop()) {
throw new IllegalStateException();
}
if (closed) {
return newFailedFuture(ctx);
}
return doWriteData(ctx, id, streamId, data, endStream);
}
protected abstract ChannelFuture doWriteData(ChannelHandlerContext ctx, int id, int streamId, HttpData data,
boolean endStream);
/**
* Resets the specified stream. If the session protocol doesn't support multiplexing or the connection
* is in unrecoverable state, the connection will be closed. For example, in an HTTP/1 connection, this
* will lead the connection to be closed immediately or after the previous requests that are not reset.
*/
public final ChannelFuture writeReset(ChannelHandlerContext ctx, int id, int streamId, Http2Error error) {
if (closed) {
return newFailedFuture(ctx);
}
return doWriteReset(ctx, id, streamId, error);
}
protected abstract ChannelFuture doWriteReset(ChannelHandlerContext ctx, int id, int streamId, Http2Error error);
/**
* Releases the resources related with this encoder and fails any unfinished writes.
*/
public void close() {
if (closed) {
return;
}
closed = true;
doClose();
}
protected abstract void doClose();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy