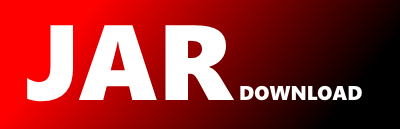
org.xbib.io.ftp.client.parser.ConfigurableFTPFileEntryParserImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ftp-client Show documentation
Show all versions of ftp-client Show documentation
Java FTP client, Java FTP NIO file system, Groovy FTP client
package org.xbib.io.ftp.client.parser;
import org.xbib.io.ftp.client.Configurable;
import org.xbib.io.ftp.client.FTPClientConfig;
import java.time.ZonedDateTime;
/**
* This abstract class implements the common timestamp parsing
* algorithm for all the concrete parsers. Classes derived from
* this one will parse file listings via a supplied regular expression
* that pulls out the date portion as a separate string which is
* passed to the underlying {@link FTPTimestampParser delegate} to
* handle parsing of the file timestamp.
* This class also implements the {@link Configurable Configurable}
* interface to allow the parser to be configured from the outside.
*/
public abstract class ConfigurableFTPFileEntryParserImpl
extends RegexFTPFileEntryParserImpl
implements Configurable {
private final FTPTimestampParser timestampParser;
/**
* constructor for this abstract class.
*
* @param regex Regular expression used main parsing of the
* file listing.
*/
public ConfigurableFTPFileEntryParserImpl(String regex) {
super(regex);
this.timestampParser = new ZonedDateTimeParser(); //new FTPTimestampParserImpl();
}
/**
* constructor for this abstract class.
*
* @param regex Regular expression used main parsing of the
* file listing.
* @param flags the flags to apply, see
* {@link java.util.regex.Pattern#compile(String, int) Pattern#compile(String, int)}. Use 0 for none.
*/
public ConfigurableFTPFileEntryParserImpl(String regex, int flags) {
super(regex, flags);
this.timestampParser = new ZonedDateTimeParser(); // new FTPTimestampParserImpl();
}
/**
* This method is called by the concrete parsers to delegate
* timestamp parsing to the timestamp parser.
*
* @param timestampStr the timestamp string pulled from the
* file listing by the regular expression parser, to be submitted
* to the timestampParser
for extracting the timestamp.
* @return a java.util.Calendar
containing results of the
* timestamp parse.
*/
public ZonedDateTime parseTimestamp(String timestampStr) {
return this.timestampParser.parseTimestamp(timestampStr);
}
/**
* Implementation of the {@link Configurable Configurable}
* interface. Configures this parser by delegating to the
* underlying Configurable FTPTimestampParser implementation, '
* passing it the supplied {@link FTPClientConfig FTPClientConfig}
* if that is non-null or a default configuration defined by
* each concrete subclass.
*
* @param config the configuration to be used to configure this parser.
* If it is null, a default configuration defined by
* each concrete subclass is used instead.
*/
@Override
public void configure(FTPClientConfig config) {
if (this.timestampParser instanceof Configurable) {
FTPClientConfig defaultCfg = getDefaultConfiguration();
if (config != null) {
if (null == config.getDefaultDateFormatStr()) {
config.setDefaultDateFormatStr(defaultCfg.getDefaultDateFormatStr());
}
if (null == config.getRecentDateFormatStr()) {
config.setRecentDateFormatStr(defaultCfg.getRecentDateFormatStr());
}
((Configurable) this.timestampParser).configure(config);
} else {
((Configurable) this.timestampParser).configure(defaultCfg);
}
}
}
/**
* Each concrete subclass must define this member to create
* a default configuration to be used when that subclass is
* instantiated without a {@link FTPClientConfig FTPClientConfig}
* parameter being specified.
*
* @return the default configuration for the subclass.
*/
protected abstract FTPClientConfig getDefaultConfiguration();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy