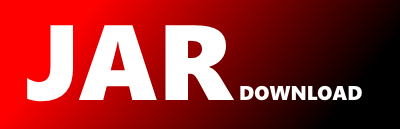
org.xbib.groovy.ftp.FTP Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of groovy-ftp Show documentation
Show all versions of groovy-ftp Show documentation
Java FTP client, Java FTP NIO file system, Groovy FTP client
package org.xbib.groovy.ftp;
import groovy.lang.Closure;
import java.net.URI;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Map;
/**
*/
public class FTP {
private final String url;
private final Map env;
private FTP(String url, Map env) {
this.url = url;
this.env = env;
}
public static FTP newInstance() {
return newInstance("ftp://localhost:21");
}
public static FTP newInstance(Map env) {
return newInstance("ftp://localhost:21", env);
}
public static FTP newInstance(String url) {
return newInstance(url, null);
}
public static FTP newInstance(String url, Map env) {
return new FTP(url, env);
}
public Boolean exists(String path) throws Exception {
return performWithContext(ctx -> Files.exists(ctx.fileSystem.getPath(path)));
}
public void each(String path, Closure> closure) throws Exception {
WithContext
© 2015 - 2025 Weber Informatics LLC | Privacy Policy