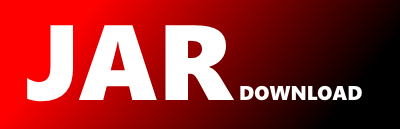
org.xblackcat.sjpu.util.function.BiConsumerEx Maven / Gradle / Ivy
Show all versions of sjpu-utils Show documentation
package org.xblackcat.sjpu.util.function;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Represents an operation that accepts two input arguments and returns no
* result. This is the two-arity specialization of {@link ConsumerEx}.
* Unlike most other functional interfaces, {@code BiConsumer} is expected
* to operate via side-effects.
*
*
This is a functional interface
* whose functional method is {@link #accept(Object, Object)}.
*
* @param the type of the first argument to the operation
* @param the type of the second argument to the operation
* @param the type of exception could be thrown while performing operation
* @see ConsumerEx
* @since 1.8
*/
@FunctionalInterface
public interface BiConsumerEx {
/**
* Performs this operation on the given arguments.
*
* @param t the first input argument
* @param u the second input argument
*/
void accept(T t, U u) throws E;
/**
* Returns a composed {@code BiConsumer} that performs, in sequence, this
* operation followed by the {@code after} operation. If performing either
* operation throws an exception, it is relayed to the caller of the
* composed operation. If performing this operation throws an exception,
* the {@code after} operation will not be performed.
*
* @param after the operation to perform after this operation
* @return a composed {@code BiConsumer} that performs in sequence this
* operation followed by the {@code after} operation
* @throws NullPointerException if {@code after} is null
*/
default BiConsumerEx andThen(BiConsumerEx super T, ? super U, E> after) {
Objects.requireNonNull(after);
return (l, U) -> {
accept(l, U);
after.accept(l, U);
};
}
default ConsumerEx fixRight(U u) {
return t -> accept(t, u);
}
default ConsumerEx fixLeft(T t) {
return u -> accept(t, u);
}
default BiConsumerEx cover(String exceptionText, BiFunction cover) {
return cover(() -> exceptionText, cover);
}
default BiConsumerEx cover(BiFunction cover) {
return cover(Throwable::getMessage, cover);
}
default BiConsumerEx cover(Supplier text, BiFunction cover) {
return cover(e -> text.get(), cover);
}
default BiConsumerEx cover(Function text, BiFunction cover) {
return (t, u) -> {
try {
accept(t, u);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
default BiConsumer unchecked(String exceptionText, BiFunction cover) {
return unchecked(() -> exceptionText, cover);
}
default BiConsumer unchecked() {
return unchecked(CoveringException::new);
}
default BiConsumer unchecked(BiFunction cover) {
return unchecked(Throwable::getMessage, cover);
}
default BiConsumer unchecked(Supplier text, BiFunction cover) {
return unchecked(e -> text.get(), cover);
}
default BiConsumer unchecked(
Function text,
BiFunction cover
) {
return (t, u) -> {
try {
accept(t, u);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
}