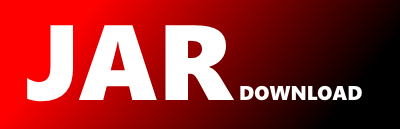
org.xblackcat.sjpu.util.function.DoublePredicateEx Maven / Gradle / Ivy
Show all versions of sjpu-utils Show documentation
package org.xblackcat.sjpu.util.function;
import java.util.Objects;
import java.util.function.BiFunction;
import java.util.function.DoublePredicate;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Represents a predicate (boolean-valued function) of one {@code double}-valued
* argument. This is the {@code double}-consuming primitive type specialization
* of {@link PredicateEx}.
*
*
This is a functional interface
* whose functional method is {@link #test(double)}.
*
* @param the type of exception could be thrown while performing operation
* @see PredicateEx
* @since 1.8
*/
@FunctionalInterface
public interface DoublePredicateEx {
/**
* Evaluates this predicate on the given argument.
*
* @param value the input argument
* @return {@code true} if the input argument matches the predicate,
* otherwise {@code false}
*/
boolean test(double value) throws E;
/**
* Returns a composed predicate that represents a short-circuiting logical
* AND of this predicate and another. When evaluating the composed
* predicate, if this predicate is {@code false}, then the {@code other}
* predicate is not evaluated.
*
*
Any exceptions thrown during evaluation of either predicate are relayed
* to the caller; if evaluation of this predicate throws an exception, the
* {@code other} predicate will not be evaluated.
*
* @param other a predicate that will be logically-ANDed with this
* predicate
* @return a composed predicate that represents the short-circuiting logical
* AND of this predicate and the {@code other} predicate
* @throws NullPointerException if other is null
*/
default DoublePredicateEx and(DoublePredicateEx other) {
Objects.requireNonNull(other);
return (value) -> test(value) && other.test(value);
}
/**
* Returns a predicate that represents the logical negation of this
* predicate.
*
* @return a predicate that represents the logical negation of this
* predicate
*/
default DoublePredicateEx negate() {
return (value) -> !test(value);
}
/**
* Returns a composed predicate that represents a short-circuiting logical
* OR of this predicate and another. When evaluating the composed
* predicate, if this predicate is {@code true}, then the {@code other}
* predicate is not evaluated.
*
*
Any exceptions thrown during evaluation of either predicate are relayed
* to the caller; if evaluation of this predicate throws an exception, the
* {@code other} predicate will not be evaluated.
*
* @param other a predicate that will be logically-ORed with this
* predicate
* @return a composed predicate that represents the short-circuiting logical
* OR of this predicate and the {@code other} predicate
* @throws NullPointerException if other is null
*/
default DoublePredicateEx or(DoublePredicateEx other) {
Objects.requireNonNull(other);
return (value) -> test(value) || other.test(value);
}
default BooleanSupplierEx fix(double value) {
return () -> test(value);
}
default DoublePredicateEx cover(String exceptionText, BiFunction cover) {
return cover(() -> exceptionText, cover);
}
default DoublePredicateEx cover(BiFunction cover) {
return cover(Throwable::getMessage, cover);
}
default DoublePredicateEx cover(Supplier text, BiFunction cover) {
return cover(e -> text.get(), cover);
}
default DoublePredicateEx cover(Function text, BiFunction cover) {
return t -> {
try {
return test(t);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
default DoublePredicate unchecked(String exceptionText, BiFunction cover) {
return unchecked(() -> exceptionText, cover);
}
default DoublePredicate unchecked() {
return unchecked(CoveringException::new);
}
default DoublePredicate unchecked(BiFunction cover) {
return unchecked(Throwable::getMessage, cover);
}
default DoublePredicate unchecked(Supplier text, BiFunction cover) {
return unchecked(e -> text.get(), cover);
}
default DoublePredicate unchecked(
Function text,
BiFunction cover
) {
return t -> {
try {
return test(t);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
}