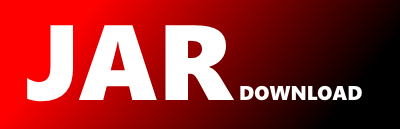
org.xblackcat.sjpu.util.function.IntToLongFunctionEx Maven / Gradle / Ivy
Show all versions of sjpu-utils Show documentation
package org.xblackcat.sjpu.util.function;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.IntToLongFunction;
import java.util.function.Supplier;
/**
* Represents a function that accepts an int-valued argument and produces a
* long-valued result. This is the {@code int}-to-{@code long} primitive
* specialization for {@link FunctionEx}.
*
*
This is a functional interface
* whose functional method is {@link #applyAsLong(int)}.
*
* @param the type of exception could be thrown while performing operation
* @see FunctionEx
* @since 1.8
*/
@FunctionalInterface
public interface IntToLongFunctionEx {
/**
* Applies this function to the given argument.
*
* @param value the function argument
* @return the function result
*/
long applyAsLong(int value) throws E;
default LongSupplierEx fix(int value) {
return () -> applyAsLong(value);
}
default IntToLongFunctionEx cover(String exceptionText, BiFunction cover) {
return cover(() -> exceptionText, cover);
}
default IntToLongFunctionEx cover(BiFunction cover) {
return cover(Throwable::getMessage, cover);
}
default IntToLongFunctionEx cover(Supplier text, BiFunction cover) {
return cover(e -> text.get(), cover);
}
default IntToLongFunctionEx cover(Function text, BiFunction cover) {
return t -> {
try {
return applyAsLong(t);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
default IntToLongFunction unchecked(String exceptionText, BiFunction cover) {
return unchecked(() -> exceptionText, cover);
}
default IntToLongFunction unchecked() {
return unchecked(CoveringException::new);
}
default IntToLongFunction unchecked(BiFunction cover) {
return unchecked(Throwable::getMessage, cover);
}
default IntToLongFunction unchecked(Supplier text, BiFunction cover) {
return unchecked(e -> text.get(), cover);
}
default IntToLongFunction unchecked(
Function text,
BiFunction cover
) {
return t -> {
try {
return applyAsLong(t);
} catch (Throwable e) {
throw cover.apply(text.apply(e), e);
}
};
}
}