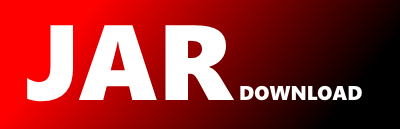
org.xerial.lens.Lens Maven / Gradle / Ivy
/*--------------------------------------------------------------------------
* Copyright 2009 Taro L. Saito
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*--------------------------------------------------------------------------*/
//--------------------------------------
// XerialJ
//
// Lens.java
// Since: Feb 23, 2009 5:50:49 PM
//
// $URL$
// $Author$
//--------------------------------------
package org.xerial.lens;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
import java.sql.ResultSet;
import java.util.List;
import java.util.Map;
import org.antlr.runtime.tree.Tree;
import org.xerial.core.XerialException;
import org.xerial.lens.tree.ANTLRTreeParser;
import org.xerial.lens.tree.JSONTreeParser;
import org.xerial.lens.tree.MapTreeParser;
import org.xerial.lens.tree.TreeParser;
import org.xerial.lens.tree.XMLTreeParser;
import org.xerial.silk.SilkParser;
import org.xerial.util.bean.BeanHandler;
import org.xerial.util.bean.TypeInfo;
/**
* Lens is an O-X mapping utility. O stands for Objects, and X for structured
* data including XML, JSON, Silk, etc.
*
*
* gene.silk
*
*
*
* -coordinate(group:utgb, species:human, revision:hg18, name:chr1)
* - gene
* -id: 1
* -name: gene1
* -start: 100
* -sequence: ACGGTTAGCGTATT
* -coordinate(group:utgb, species:human, revision:hg18, name:chr2)
* - gene
* -id: 2
* -name: gene2
* -start: 250
* -exon(start, end)*: [[250, 255], [260, 265]]
* -sequence: TAGCGTATTAAATT
*
*
*
* gene-alt.silk (Alternative data description)
*
*
*
* -(group:utgb, species:human, revision:hg18)
* - coordinate(name:chr1)
* - gene(id:1, name: gene1, start: 100, sequence: ACGGTTAGCGTATT)
* -coordinate(name:chr2)
* - gene(id:2, name: gene2, start: 250, exon(start, end): [[250, 255], [260, 265]], sequence: TAGCGTATTAAATT)
*
*
*
* class Coordinate
* {
* public String group;
* public String species;
* public String revision;
* public String name;
* }
*
* class Gene
* {
* public int id;
* public String name;
* public long start;
* public String sequence;
* public List<Exon> exonList;
* }
*
* class Exon
* {
* public start;
* public end;
* }
*
*
*
*
*
* Query Class
*
*
*
* class GeneList {
* // map of List value type corresponds to two-argument adder
* public Map<Coordinate, List<Gene>> geneTable;
*
* // for adding unknown parameters
* public Map<String, String> properties;
*
* }
*
*
*
*
* class GeneList {
*
* // map of List value type corresponds to two-argument adder
* public Map<Coordinate, List<Gene>> geneTable;
*
* // add gene to the geneList table (if you use public Map field, no need to write the following method)
* public void add(Coordinate coordinate, Gene gene) {
* List<Gene> geneList = geneTable.get(coordinate);
* if (geneList == null) {
* geneList = new ArrayList<Gene>();
* geneTable.put(coordinate, geneList);
* }
*
* geneList.add(gene);
* }
*
* // for adding unknown parameters
* public Map<String, String> properties;
*
* public void put(String key, String value) {
* this.properties.put(key, value);
* }
*
* }
*
*
*
*
* @author leo
*
*/
public class Lens {
/**
* Translate the specified Silk file into an instance of the target object.
*
* @param
* @param silkFileResource
* resource path to the input Silk file
* @param targetClass
* target class to which the input Silk format will be converted
*
* @return translated object of the targetClass type
* @throws IOException
* when failed to read resources
* @throws XerialException
* when failed to parse the input Silk file
*/
public static Result loadSilk(Class targetType, URL silkResource)
throws IOException, XerialException {
return loadSilk(TypeInfo.createInstance(targetType), silkResource);
}
public static Result loadSilk(Result result, URL silkResource) throws IOException,
XerialException {
if (silkResource == null)
throw new NullPointerException("silk resouce is null");
return load(result, new SilkParser(silkResource));
}
public static Result loadSilk(Result result, Reader silkReader)
throws XerialException, IOException {
return load(result, new SilkParser(silkReader));
}
public static Result loadSilk(Class resultType, Reader silkReader)
throws XerialException, IOException {
return loadSilk(TypeInfo.createInstance(resultType), silkReader);
}
public static Result loadXML(Result result, URL xmlResource) throws IOException,
XerialException {
if (xmlResource == null)
throw new NullPointerException("XML resource is null");
return load(result, new XMLTreeParser(new BufferedReader(new InputStreamReader(xmlResource
.openStream()))));
}
public static Result loadXML(Class result, URL xmlResource)
throws IOException, XerialException {
return loadXML(TypeInfo.createInstance(result), xmlResource);
}
public static Result loadXML(Class result, Reader xmlReader)
throws IOException, XerialException {
return loadXML(TypeInfo.createInstance(result), xmlReader);
}
public static Result loadXML(Result result, Reader xmlReader) throws IOException,
XerialException {
return load(result, new XMLTreeParser(xmlReader));
}
public static Result loadJSON(Class targetType, Reader jsonReader)
throws XerialException, IOException {
return loadJSON(TypeInfo.createInstance(targetType), jsonReader);
}
public static Result loadJSON(Result result, Reader jsonReader)
throws XerialException, IOException {
return load(result, new JSONTreeParser(jsonReader));
}
public static Result loadANTLRParseTree(Class resultType, Tree tree,
final String[] parserTokenNames) throws XerialException {
return loadANTLRParseTree(TypeInfo.createInstance(resultType), tree, parserTokenNames);
}
public static Result loadANTLRParseTree(Result result, Tree tree,
final String[] parserTokenNames) throws XerialException {
return load(result, new ANTLRTreeParser(parserTokenNames, tree));
}
public static Result loadMap(Class resultType, Map< ? , ? > map)
throws XerialException {
return loadMap(TypeInfo.createInstance(resultType), map);
}
public static Result loadMap(Result result, Map< ? , ? > map) throws XerialException {
return load(result, new MapTreeParser(map));
}
public static void loadJDBCResultSet(Class resultType, ResultSet rs,
BeanHandler handler) throws Exception {
JDBCLens jl = new JDBCLens(resultType);
jl.mapAll(rs, handler);
}
public static List loadJDBCResultSet(Class resultType, ResultSet rs)
throws Exception {
JDBCLens jl = new JDBCLens(resultType);
return jl.mapAll(rs);
}
public static Result load(Class targetType, TreeParser parser)
throws XerialException {
return load(TypeInfo.createInstance(targetType), parser);
}
public static Result load(Result result, TreeParser parser) throws XerialException {
ObjectMapper mapper = ObjectMapper.getMapper(result.getClass());
return mapper.map(result, parser);
}
public static String toJSON(Object obj) {
return ObjectLens.toJSON(obj);
}
public static String toSilk(Object obj) {
return ObjectLens.toSilk(obj);
}
/**
* Find the target nodes of the specified type from the input Silk data. The
* given object handler will be used to report the found target objects.
*
* @param
* @param silkResource
* @param targetNodeName
* @param targetType
* @param handler
* @throws IOException
* @throws XerialException
*/
public static void findFromSilk(URL silkResource, String targetNodeName,
Class targetType, ObjectHandler handler) throws IOException,
XerialException {
find(targetType, targetNodeName, handler, new SilkParser(silkResource));
}
public static void findFromSilk(Reader input, String targetNodeName,
Class targetType, ObjectHandler handler) throws XerialException,
IOException {
find(targetType, targetNodeName, handler, new SilkParser(input));
}
public static void findFromXML(Reader input, String targetNodeName,
Class targetType, ObjectHandler handler) throws XerialException {
find(targetType, targetNodeName, handler, new XMLTreeParser(input));
}
public static void findFromJSON(Reader input, String targetNodeName,
Class targetType, ObjectHandler handler) throws XerialException,
IOException {
find(targetType, targetNodeName, handler, new JSONTreeParser(input));
}
public static void find(Class bindingType, ObjectHandler handler,
TreeParser parser) throws XerialException {
find(bindingType, bindingType.getSimpleName(), handler, parser);
}
public static void find(Class bindingType, String targetNodeName,
ObjectHandler handler, TreeParser parser) throws XerialException {
ObjectMapper mapper = new ObjectMapper(bindingType, targetNodeName);
mapper.find(handler, targetNodeName, parser);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy