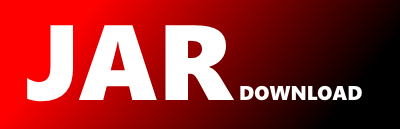
org.xerial.util.CollectionUtil Maven / Gradle / Ivy
/*--------------------------------------------------------------------------
* Copyright 2007 Taro L. Saito
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*--------------------------------------------------------------------------*/
//--------------------------------------
// XerialJ Project
//
// CollectionUtil.java
// Since: May 8, 2007
//
// $URL$
// $Author$
//--------------------------------------
package org.xerial.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.TreeSet;
public class CollectionUtil
{
/**
* Non constractable class
*/
private CollectionUtil()
{}
public static List toString(Collection collection)
{
ArrayList result = new ArrayList();
for (In input : collection)
{
if (input != null)
result.add(input.toString());
else
result.add(null);
}
return result;
}
public static List collectFromNonGenericCollection(Iterable collection, Functor functor)
{
ArrayList result = new ArrayList();
for (In input : collection)
{
result.add(functor.apply(input));
}
return result;
}
public static List select(Iterable collection, Predicate filter)
{
ArrayList result = new ArrayList();
for (In input : collection)
{
if (filter.apply(input))
result.add(input);
}
return result;
}
public static List collect(Iterable collection, Functor functor)
{
ArrayList result = new ArrayList();
for (In input : collection)
{
result.add(functor.apply(input));
}
return result;
}
public static List collect(In[] collection, Functor functor)
{
ArrayList result = new ArrayList();
for (In input : collection)
{
result.add(functor.apply(input));
}
return result;
}
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy