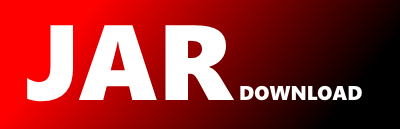
org.xerial.db.sql.ObjectStorage Maven / Gradle / Ivy
The newest version!
/*--------------------------------------------------------------------------
* Copyright 2007 Taro L. Saito
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*--------------------------------------------------------------------------*/
//--------------------------------------
// XerialJ
//
// ObjectStorage.java
// Since: Jun 26, 2008 2:33:25 PM
//
// $URL$
// $Author$
//--------------------------------------
package org.xerial.db.sql;
import java.util.Collection;
import java.util.List;
import org.xerial.db.DBException;
import org.xerial.util.Predicate;
/**
* Ruby on Rails-Style Relational Database Access Interface
*
* Conventions used in the {@link ObjectStorage}:
*
*
* - column name id (setId(), getId()) is considered as an integer primary key
* (auto-increment) value.
* - createdAt, updatedAt column names correspond to {@link DateTimeType} (
* {@link java.util.Date} ) of the creation, update time of the instance,
* respectively.
* - one-to-many relationship
*
*
*
*
* @author leo
*
*/
public interface ObjectStorage
{
/**
* Register a class type that can be used as save/load unit to the table.
* That is each row in the table corresponds to an instance of the given
* classType.
*
* If no such table exists in the database, this method creates a new table
* for the given class type.
*
* @param
* @param tableName
* the table Name
* @param classType
* class type corresponding to each row of the table
* @throws DBException
*/
public void register(String tableName, Class classType) throws DBException;
/**
* Register a class type that can be used as save/load unit to the table.
* That is each row in the table corresponds to an instance of the given
* classType.
*
* The table name is classType.getName().toLowerCase();
*
* If no such table exists in the database, this method creates a new table
* for the given class type.
*
* @param
* @param classType
* @throws DBException
*/
public void register(Class classType) throws DBException;
public void drop(Class classType) throws DBException;
/**
* Associate type T to type U with one-to-one relationship
*
* @param
* @param
* @param from
* @param to
* @throws DBException
*/
public void oneToOne(Class from, Class to) throws DBException;
/**
* Associate type T to type U with one-to-many relationship. That means T
* and U has the following table structure:
*
*
* T: (id, ...)
* U: (id, TId, ...)
*
*
* For example, when T = Student and U is report, the table structure will
* be as follows:
*
*
* Student (id, name, ...)
* Report (id, studentId, ...)
*
*
*
*
* @param
* @param
* @param from
* @param many
* @throws DBException
*/
//public void oneToMany(Class from, Class to) throws DBException;
public U getOne(T startPoint, Class associatedType) throws DBException;
public U getOne(T startPoint, Class associatedType, int idOfU) throws DBException;
public U getOne(Class startPointClass, int idOfT, Class associatedType) throws DBException;
public U getOne(Class startPointClass, int idOfT, Class associatedType, int idOfU) throws DBException;
public List getAll(T startPoint, Class associatedType) throws DBException;
public List getAllWithSorting(T startPoint, Class associatedType) throws DBException;
public List getAll(T startPoint, Class associatedType, QueryParam queryParam) throws DBException;
public List getAll(Class startPointClass, int idOfT, Class associtedType) throws DBException;
public List getAll(Class startPointClass, int idOfT, Class associtedType, QueryParam queryParam)
throws DBException;
/**
* Retrieves all object instances in the corresponding table
*
* @param
* @param classType
* the object type to be retrieved
* @return the list of objects of the specified type
* @throws DBException
*/
public List getAll(Class classType) throws DBException;
/**
* Retrieves object instances using an SQL statement.
*
* @param
* @param classType
* the object type to be retrieved
* @param sql
* the SQL statement
* @return
* @throws DBException
*/
public List getAll(Class classType, String sql) throws DBException;
/**
* Retrieves object instances that satisfy the predicate
*
* @param
* @param classType
* @param filterPredicate
* @return
* @throws DBException
*/
public List getAll(Class classType, Predicate filterPredicate) throws DBException;
/**
* Retrieves the object from the database that matches the given id value
*
* @param
* @param id
* the ID of the object
* @return the retrieved object, or null if not found
* @throws DBException
*/
public T get(Class classType, int id) throws DBException;
/**
* Retrieves an object using an SQL statement
*
* @param
* @param classType
* the object type to be retrieved
* @param sql
* the SQL statment
* @return the retrieved object. or null if not found
* @throws DBException
*/
public T get(Class classType, String sql) throws DBException;
/**
* Retrieves an object of the type T using {@link QueryParam}
*
* @param
* @param classType
* @param queryParam
* @return
* @throws DBException
*/
public T get(Class classType, QueryParam queryParam) throws DBException;
/**
* Retrieves a list of objects of the type T using {@link QueryParam}
*
* @param
* @param classType
* @param queryParam
* @return
* @throws DBException
*/
public List getAll(Class classType, QueryParam queryParam) throws DBException;
/**
* Count the number of rows of the class T
*
* @param
* @param classType
* @return
* @throws DBException
*/
public int count(Class classType) throws DBException;
/**
* Count the number of matching rows of the class T with the given condition
*
* @param
* @param classType
* @param queryParam
* @return
* @throws DBException
*/
public int count(Class classType, QueryParam queryParam) throws DBException;
public T getParent(U child, Class parentType) throws DBException;
public T getParent(Class parentClass, Class childClass, int idOfU) throws DBException;
/**
* Create a new object of the type U associated with the given parent object
*
* @param
* @param
* @param parentBean
* @param associatedObject
*/
public U create(T parentObject, U associatedObject) throws DBException;
/**
* Create a new row in the database. The returned object is the same
* instance with the given one but its ID attribute is set (using setId()
* method) to the ID of the newly created object.
*
* @param
* @param the
* object initial value of the object (id attribute is ignored)
* @return the given object instance whose ID is set.
*/
public T create(T object) throws DBException;
/**
* Save the object data in the database
*
* @param
* @param object
* the object to be stored in the database
*/
public void save(T object) throws DBException;
/**
* Save the all objects in the given collection
*
* @param
* @param classType
* the object type to be saved
* @param object
* the collection of the objects to be saved
* @throws DBException
*/
public void saveAll(Class classType, Collection object) throws DBException;
/**
* Delete the object
*
* @param
* @param object
* @throws DBException
*/
public void delete(T object) throws DBException;
/**
* Delete the object of the specified id
*
* @param
* @param objectType
* @param id
* @throws DBException
*/
public void delete(Class objectType, int id) throws DBException;
/**
* Create the object in many-many relationship (T <-- V --> U)
*
* @param
* @param
* @param
* @param parent
* @param parent2
* @param newObject
* @return
* @throws DBException
*/
public V create(T parent, U parent2, V newObject) throws DBException;
/**
* Create the object in many-many relationship (T <-- V --> U)
*
* @param
* @param
* @param
* @param parent
* @param idOfT
* @param parent2
* @param idOfU
* @param newObject
* @return
* @throws DBException
*/
public V create(Class parent, int idOfT, Class parent2, int idOfU, V newObject) throws DBException;
/**
* Get many-many relationship V between T and U
*
* @param
* @param
* @param
* @param parent
* @param parent2
* @param objectType
* @return
* @throws DBException
*/
public V get(Class parent, int idOfT, Class parent2, int idOfU, Class objectType)
throws DBException;
public int count(Class parent, int idOfT, Class objectType) throws DBException;
public int count(Class parent, int idOfT, Class parent2, int idOfU, Class objectType)
throws DBException;
// view query
public List getAllFromView(Class viewType, Class objectType)
throws DBException;
public List getAllFromView(Class viewType, String sql) throws DBException;
public View getFromView(Class viewType, Class objectType) throws DBException;
public View getFromView(Class viewType, String sql) throws DBException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy