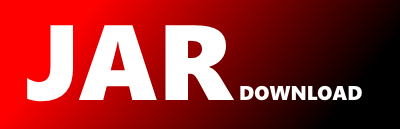
org.xillium.gear.auth.PermissionFilesAuthority Maven / Gradle / Ivy
package org.xillium.gear.auth;
import java.io.*;
import java.util.*;
import java.util.logging.*;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
/**
* An Authority that manages roles and permissions in permission files, which are either in the file system or on the class path.
*
* A permission file is a plain text document containing lines of permission specifications. Each permission specification is given
* in a single line with the format "ROLE_ID:FUNCTION:PERMISSION".
*/
public class PermissionFilesAuthority implements Authority {
private static final Logger _logger = Logger.getLogger(PermissionFilesAuthority.class.getName());
private final List _permissions = new ArrayList();
/**
* Constructs a PermissionFilesAuthority.
*
* @param locations - a list of permission file locations or location patterns as supported by
* org.springframework.core.io.support.PathMatchingResourcePatternResolver
*/
public PermissionFilesAuthority(List locations) {
PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
for (String location: locations) {
try {
for (Resource resource: resolver.getResources(location)) {
BufferedReader reader = new BufferedReader(new InputStreamReader(resource.getInputStream()));
try {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(":");
try {
_permissions.add(new Permission(parts[0], parts[1], Integer.parseInt(parts[2])));
} catch (Exception x) {
_logger.warning("Ignored invalid permission: " + line);
}
}
} finally {
reader.close();
}
}
} catch (Exception x) {}
}
}
/**
* Loads all role permissions into memory.
*/
public List loadRolesAndPermissions() throws Exception {
return _permissions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy