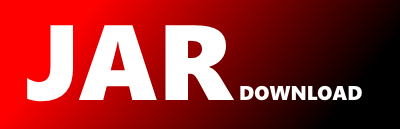
org.xipki.ca.gateway.RestResponse Maven / Gradle / Ivy
// Copyright (c) 2013-2023 xipki. All rights reserved.
// License Apache License 2.0
package org.xipki.ca.gateway;
import org.xipki.util.Base64;
import org.xipki.util.CollectionUtil;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.Map;
/**
*
* @author Lijun Liao (xipki)
* @since 6.0.0
*/
public class RestResponse {
private final int statusCode;
private final String contentType;
private final Map headers;
private final boolean base64;
private final byte[] body;
public RestResponse(int statusCode) {
this(statusCode, null, null, false, null);
}
public RestResponse(int statusCode, String contentType, Map headers, byte[] body) {
this(statusCode, contentType, headers, false, body);
}
public RestResponse(int statusCode, String contentType, Map headers, boolean base64, byte[] body) {
this.statusCode = statusCode;
this.base64 = base64;
this.contentType = contentType;
this.headers = headers;
this.body = body;
}
public int getStatusCode() {
return statusCode;
}
public String getContentType() {
return contentType;
}
public Map getHeaders() {
return headers;
}
public byte[] getBody() {
return body;
}
public void fillResponse(HttpServletResponse resp) throws IOException {
resp.setStatus(statusCode);
if (contentType != null) {
resp.setContentType(contentType);
}
if (CollectionUtil.isNotEmpty(headers)) {
for (Map.Entry m : headers.entrySet()) {
resp.setHeader(m.getKey(), m.getValue());
}
}
if (body == null) {
resp.setContentLength(0);
} else {
byte[] content;
if (base64) {
resp.setHeader("Content-Transfer-Encoding", "base64");
content = Base64.encodeToByte(body, true);
} else {
content = body;
}
resp.setContentLength(content.length);
resp.getOutputStream().write(content);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy