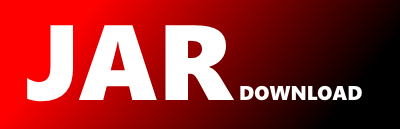
org.xipki.ca.gateway.CaNameSigners Maven / Gradle / Ivy
// Copyright (c) 2013-2023 xipki. All rights reserved.
// License Apache License 2.0
package org.xipki.ca.gateway;
import org.xipki.security.ConcurrentContentSigner;
import org.xipki.util.Args;
import org.xipki.util.CollectionUtil;
import org.xipki.util.exception.InvalidConfException;
import java.util.*;
/**
*
* @author Lijun Liao (xipki)
* @since 6.0.0
*/
public class CaNameSigners {
private final ConcurrentContentSigner defaultSigner;
private final Map signers;
public CaNameSigners(ConcurrentContentSigner defaultSigner, Map signers)
throws InvalidConfException {
if (defaultSigner == null && CollectionUtil.isEmpty(signers)) {
throw new InvalidConfException("At least one of defaultSigner and signers must be set");
}
this.defaultSigner = defaultSigner;
if (signers == null) {
this.signers = null;
} else {
this.signers = new HashMap<>(signers.size() * 3 / 2);
for (Map.Entry m : signers.entrySet()) {
String name = m.getKey().toLowerCase(Locale.ROOT);
if (this.signers.containsKey(name)) {
throw new InvalidConfException("at least two signers for the CA " + name + " are set");
}
this.signers.put(m.getKey().toLowerCase(Locale.ROOT), m.getValue());
}
}
}
public ConcurrentContentSigner getSigner(String caName) {
String loName = Args.toNonBlankLower(caName, "caName");
if (signers != null) {
ConcurrentContentSigner signer = signers.get(loName);
if (signer != null) {
return signer;
}
}
return defaultSigner;
}
public ConcurrentContentSigner getDefaultSigner() {
return defaultSigner;
}
public Set signerNames() {
return signers == null ? Collections.emptySet() : signers.keySet();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy