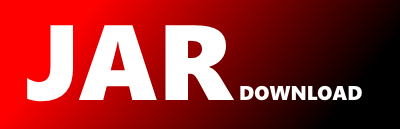
org.xipki.security.pkcs11.jaxb.ObjectFactory Maven / Gradle / Ivy
package org.xipki.security.pkcs11.jaxb;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.xipki.security.pkcs11.jaxb package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _PKCS11Conf_QNAME = new QName("http://xipki.org/security/pkcs11/conf/v2", "PKCS11Conf");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.xipki.security.pkcs11.jaxb
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ModuleType }
*
*/
public ModuleType createModuleType() {
return new ModuleType();
}
/**
* Create an instance of {@link PKCS11ConfType }
*
*/
public PKCS11ConfType createPKCS11ConfType() {
return new PKCS11ConfType();
}
/**
* Create an instance of {@link ModulesType }
*
*/
public ModulesType createModulesType() {
return new ModulesType();
}
/**
* Create an instance of {@link NativeLibraryType }
*
*/
public NativeLibraryType createNativeLibraryType() {
return new NativeLibraryType();
}
/**
* Create an instance of {@link SlotsType }
*
*/
public SlotsType createSlotsType() {
return new SlotsType();
}
/**
* Create an instance of {@link SlotType }
*
*/
public SlotType createSlotType() {
return new SlotType();
}
/**
* Create an instance of {@link PasswordSetsType }
*
*/
public PasswordSetsType createPasswordSetsType() {
return new PasswordSetsType();
}
/**
* Create an instance of {@link PasswordsType }
*
*/
public PasswordsType createPasswordsType() {
return new PasswordsType();
}
/**
* Create an instance of {@link MechanismSetsType }
*
*/
public MechanismSetsType createMechanismSetsType() {
return new MechanismSetsType();
}
/**
* Create an instance of {@link MechanismsType }
*
*/
public MechanismsType createMechanismsType() {
return new MechanismsType();
}
/**
* Create an instance of {@link ModuleType.NativeLibraries }
*
*/
public ModuleType.NativeLibraries createModuleTypeNativeLibraries() {
return new ModuleType.NativeLibraries();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PKCS11ConfType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://xipki.org/security/pkcs11/conf/v2", name = "PKCS11Conf")
public JAXBElement createPKCS11Conf(PKCS11ConfType value) {
return new JAXBElement(_PKCS11Conf_QNAME, PKCS11ConfType.class, null, value);
}
}