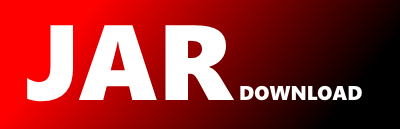
org.xj4.lifecycle.Lifecycle Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2008 Peach Jean Solutions
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xj4.lifecycle;
import java.lang.reflect.Field;
import java.util.List;
import org.xj4.XJ4TestClass;
/**
* A lifecycle that gets to operate on a field. Essentially provides class-level, method-level, and validation hooks.
* Implementations should consider extending {@link AbstractLifecycle}. The general idea is that a lifecycle class can
* replace boilerplate {@link org.junit.Before @Before} and {@link org.junit.After @After} methods for standard fields.
*
* @author Jared Bunting
*/
public interface Lifecycle {
/**
* Indicates if this lifecycle supports class level (static) fields. If so, {@link #start} and {@link #stop} methods
* will be called as if they were {@link org.junit.BeforeClass @BeforeClass} and
* {@link org.junit.AfterClass @AfterClass} methods respectively.
* @return true if the lifecycle supports class level fields
*/
public boolean supportsClassLevel();
/**
* Indicates if this lifecycle supports instance level fields. If so, {@link #start} and {@link #stop} methods will
* be called as if they were {@link org.junit.Before @Before} and {@link org.junit.After @After} methods respectively.
* @return true if the lifecycle supports instance level fields
*/
public boolean supportsInstanceLevel();
/**
* Performs the before portion of a field lifecycle. If this is a class level field, the target will be
* null
.
* @param testClass the test class
* @param field the managed field
* @param target the target class, or null if a class level field
* @param dependencies accessor for dependencies that have been specified with {@link Requires @Requires}.
*/
public void start(XJ4TestClass testClass, Field field, Object target, Dependencies dependencies);
/**
* Performs the after portion of a field lifecycle. If this is a class level field, the target will be
* null
.
* @param testClass the test class
* @param field the managed field
* @param target the target class, or null if a class level field
* @param dependencies accessor for dependencies that have been specified with {@link Requires @Requires}.
*/
public void stop(XJ4TestClass testClass, Field field, Object target, Dependencies dependencies);
/**
* Specifies the type of a field that this lifecycle will operate on. When {@link Manage @Manage} doesn't explicitly
* specify a lifecycle, this is used to discover appropriate lifecycles to use.
* @return the field type that this lifecycle will operate on
*/
public Class> requiredType();
/**
* Specifies whether or not a field managed by this lifecycle must have a value assigned to it prior to being managed.
* @return true if the field can be null, false if a value is required
*/
public boolean acceptsNull();
/**
* Performs validation of the field. This could mean many things to different lifecycles, but if conditions are such
* that this lifecycle can't properly manage the field, this method should add an error to the error list.
* @param errors the error list
* @param field the managed field
*/
public void validate(List errors, Field field);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy