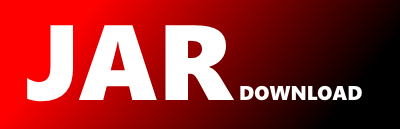
org.xlightweb.client.XHttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xlightweb-HTML5Preview
Show all versions of xlightweb-HTML5Preview
xLightweb HTML5 extension which supports ServerSentEvents ands WebSockets
The newest version!
/*
* Copyright (c) xlightweb.org, 2008 - 2010. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb.client;
import java.io.IOException;
import java.net.URI;
import javax.net.ssl.SSLContext;
import org.xlightweb.EventDataSource;
import org.xlightweb.IHttpRequestHandler;
import org.xlightweb.IEventDataSource;
import org.xlightweb.IEventHandler;
import org.xlightweb.IWebSocketConnection;
import org.xlightweb.IWebSocketHandler;
import org.xlightweb.WebSocketConnection;
/**
* Higher level client-side abstraction of the client side endpoint. Internally, the XHttpClient uses a pool
* of {@link HttpClientConnection} to perform the requests. Example:
*
*
* XHttpClient httpClient = new XHttpClient();
*
* // set some properties
* httpClient.setFollowsRedirect(true);
* httpClient.setAutoHandleCookies(false);
* // ...
*
* // perform a synchronous call
* IHttpResponse response = httpClient.call(new GetRequest("http://www.gmx.com/index.html"));
* System.out.println(response.getStatus());
*
* BlockingBodyDataSource bodyChannel = response.getBlockingBody();
* System.out.println(bodyChannel.readString());
*
*
* // perform an asynchronous request
* MyResponseHandler respHdl = new MyResponseHandler();
* httpClient.send(new HttpRequestHeader("GET", "http://www.gmx.com/index.html"), respHdl);
*
* //..
*
* httpClient.close();
*
*
* The HttpClient is thread-safe
*
* @author [email protected]
*/
public class XHttpClient extends HttpClient {
/**
* constructor
*
* @param interceptors interceptor
*/
public XHttpClient(IHttpRequestHandler... interceptors) {
super(interceptors);
}
/**
* constructor
*
* @param sslCtx the ssl context to use
*/
public XHttpClient(SSLContext sslCtx) {
super(sslCtx);
}
/**
* constructor
*
* @param sslCtx the ssl context to use
* @param interceptors the interceptors
*/
public XHttpClient(SSLContext sslCtx, IHttpRequestHandler... interceptors) {
super(sslCtx, interceptors);
}
public IEventDataSource openEventDataSource(String uriString, String... headerlines) throws IOException {
return openEventDataSource(uriString, true, headerlines);
}
public IEventDataSource openEventDataSource(String uriString, boolean isIgnoreCommentMessage) throws IOException {
return openEventDataSource(uriString, isIgnoreCommentMessage, new String[0]);
}
public IEventDataSource openEventDataSource(String uriString, boolean isIgnoreCommentMessage, String... headerlines) throws IOException {
return openEventDataSource(uriString, isIgnoreCommentMessage, null, headerlines);
}
public IEventDataSource openEventDataSource(String uriString, IEventHandler webEventHandler, String... headerlines) throws IOException {
return new EventDataSource(this, uriString, true, webEventHandler, headerlines);
}
public IEventDataSource openEventDataSource(String uriString, boolean isIgnoreCommentMessage, IEventHandler webEventHandler, String... headerlines) throws IOException {
return new EventDataSource(this, uriString, isIgnoreCommentMessage, webEventHandler, headerlines);
}
public IWebSocketConnection openWebSocketConnection(String uriString) throws IOException {
return openWebSocketConnection(uriString, (String) null);
}
public IWebSocketConnection openWebSocketConnection(String uriString, String protocol) throws IOException {
return openWebSocketConnection(uriString, protocol, null);
}
public IWebSocketConnection openWebSocketConnection(String uriString, IWebSocketHandler webSocketHandler) throws IOException {
return openWebSocketConnection(uriString, null, webSocketHandler);
}
public IWebSocketConnection openWebSocketConnection(String uriString, String protocol, IWebSocketHandler webSocketHandler) throws IOException {
URI uri = URI.create(uriString);
int port = uri.getPort();
if (port == -1) {
if (uri.getScheme().toLowerCase().equals("wss")) {
port = 443;
} else {
port = 80;
}
}
return new WebSocketConnection(this, uri, protocol, webSocketHandler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy