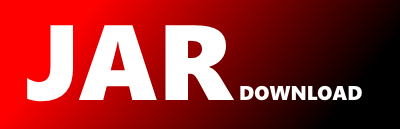
org.xlightweb.server.HttpServer Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008 - 2010. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb.server;
import java.io.IOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.net.ssl.SSLContext;
import org.xlightweb.HttpResponse;
import org.xlightweb.HttpUtils;
import org.xlightweb.IHttpConnectionHandler;
import org.xlightweb.IHttpRequestHandler;
import org.xlightweb.server.TransactionMonitor.Transaction;
import org.xlightweb.server.TransactionMonitor.TransactionLog;
import org.xsocket.connection.ConnectionUtils;
import org.xsocket.connection.NonBlockingConnection;
import org.xsocket.connection.Server;
/**
* A HttpServer. The http server accepts incoming connections and forwards the request to
* the assigned {@link IHttpRequestHandler}. Example:
*
*
*
* // defining a request handler
* class MyRequestHandler implements IHttpRequestHandler {
*
* public void onRequest(IHttpExchange exchange) throws IOException {
* IHttpRequest request = exchange.getRequest();
* //...
*
* exchange.send(new HttpResponse(200, "text/plain", "it works"));
* }
* }
*
*
* // creates a server
* IServer server = new HttpServer(8080, new MyRequestHandler());
*
* // setting some properties
* server.setMaxTransactions(400);
* server.setRequestTimeoutMillis(5 * 60 * 1000);
* //...
*
*
* // executing the server
* server.run(); // (blocks forever)
*
* // or server.start(); (blocks until the server has been started)
* //...
*
*
*
* @author [email protected]
*/
public class HttpServer extends Server implements IHttpServer {
// transaction monitor
private TransactionMonitor transactionMonitor = null;
private final TransactionLog transactionLog = new TransactionLog(0);
/**
* constructor
*
* @param requestHandler the requestHandler
* @throws IOException If some other I/O error occurs
* @throws UnknownHostException if the local host cannot determined
*/
public HttpServer(IHttpRequestHandler requestHandler) throws UnknownHostException, IOException {
this(new InetSocketAddress(0), new HashMap(), requestHandler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
* @param handler the handler to use
* @param options the socket options
* @throws IOException If some other I/O error occurs
* @throws UnknownHostException if the local host cannot determined
*/
public HttpServer(IHttpRequestHandler handler, Map options) throws UnknownHostException, IOException {
this(new InetSocketAddress(0), options, handler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
* @param port the local port
* @param handler the handler to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), new HashMap(), handler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
* @param port the local port
* @param handler the handler to use
* @param minPoolsize the min workerpool size
* @param maxPoolsize the max workerpool size
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler, int minPoolsize, int maxPoolsize) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), new HashMap(), handler, null, false, minPoolsize, maxPoolsize);
}
/**
* constructor
*
*
* @param port the local port
* @param handler the handler to use
* @param options the acceptor socket options
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler, Map options) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), options, handler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param address the local address
* @param port the local port
* @param handler the handler to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, IHttpRequestHandler handler) throws UnknownHostException, IOException {
this(address, port, handler, new HashMap(), null, false);
}
/**
* constructor
*
*
* @param host the local hodt name
* @param port the local port
* @param handler the handler to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String host, int port, IHttpRequestHandler handler) throws UnknownHostException, IOException {
this(new InetSocketAddress(host, port), new HashMap(), handler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param host the local host
* @param port the local port
* @param handler the handler to use
* @param options the socket options
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String host, int port, IHttpRequestHandler handler, Map options) throws UnknownHostException, IOException {
this(new InetSocketAddress(host, port), options, handler, null, false, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param port local port
* @param handler the handler to use
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), new HashMap(), handler, sslContext, sslOn, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param port local port
* @param handler the handler to use
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @param minPoolsize the min workerpool size
* @param maxPoolsize the max workerpool size
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler, SSLContext sslContext, boolean sslOn, int minPoolsize, int maxPoolsize) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), new HashMap(), handler, sslContext, sslOn, minPoolsize, maxPoolsize);
}
/**
* constructor
*
* @param port local port
* @param options the acceptor socket options
* @param handler the handler to use
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpRequestHandler handler, Map options, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(port), options, handler, sslContext, sslOn, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
* @param hostname local hostname
* @param port local port
* @param handler the handler to use
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String hostname, int port, IHttpRequestHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(hostname, port), new HashMap(), handler, sslContext, sslOn, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param hostname local hostname
* @param port local port
* @param options the acceptor socket options
* @param handler the handler to use
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String hostname, int port, IHttpRequestHandler handler, Map options, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(hostname, port), options, handler, sslContext, sslOn, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
/**
* constructor
*
*
* @param address local address
* @param port local port
* @param handler the request handler
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, IHttpRequestHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(address, port, handler, new HashMap(), sslContext, sslOn);
}
/**
* constructor
*
*
* @param address local address
* @param port local port
* @param options the socket options
* @param handler the request handler
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, IHttpRequestHandler handler, Map options, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(address, port), options, handler, sslContext, sslOn, MIN_SIZE_WORKER_POOL, SIZE_WORKER_POOL);
}
private HttpServer(InetSocketAddress address, Map options, IHttpRequestHandler requestHandler, SSLContext sslContext, boolean sslOn, int minPoolsize, int maxPoolsize) throws UnknownHostException, IOException {
this(address, options, requestHandler, null, sslContext, sslOn, minPoolsize, maxPoolsize);
}
HttpServer(InetSocketAddress address, Map options, IHttpRequestHandler requestHandler, IUpgradeHandler upgradeHandler, SSLContext sslContext, boolean sslOn, int minPoolsize, int maxPoolsize) throws UnknownHostException, IOException {
super(address, options, new HttpProtocolAdapter(requestHandler, upgradeHandler), sslContext, sslOn, 0, minPoolsize, maxPoolsize);
}
/**
* adds a connection handler
*
* @param connectionHandler the connection handler to add
*/
public void addConnectionHandler(IHttpConnectionHandler connectionHandler) {
((HttpProtocolAdapter) super.getHandler()).addConnectionHandler(connectionHandler);
}
protected final void onPreRejectConnection(NonBlockingConnection connection) throws IOException {
HttpResponse response = new HttpResponse(503);
response.setServer(ServerUtils.getComponentInfo());
response.setHeader("Connection", "close");
response.setHeader("Content-Length", "0");
connection.write(response.toString());
}
/**
* returns the implementation version
*
* @return the implementation version
*/
public String getImplementationVersion() {
return HttpUtils.getImplementationVersion();
}
int getNumHandledConnections() {
return ((HttpProtocolAdapter) super.getHandler()).getNumHandledConnections();
}
/**
* returns the implementation date
*
* @return the implementation date
*/
public String getImplementationDate() {
return HttpUtils.getImplementationDate();
}
/**
* {@inheritDoc}
*/
public IHttpRequestHandler getRequestHandler() {
return (IHttpRequestHandler) ((HttpProtocolAdapter) getHandler()).getRequestHandler();
}
/**
* returns the xSocket implementation version
*
* @return the xSocket implementation version
*/
String getXSocketImplementationVersion() {
return ConnectionUtils.getImplementationVersion();
}
/**
* returns the xScoket implementation date
*
* @return the xSocket implementation date
*/
String getXSocketImplementationDate() {
return ConnectionUtils.getImplementationDate();
}
/**
* {@inheritDoc}
*/
public void setRequestTimeoutMillis(long receivetimeout) {
((HttpProtocolAdapter) super.getHandler()).setRequestTimeoutMillis(receivetimeout);
}
/**
* {@inheritDoc}
*/
public void setAutoCompressThresholdBytes(int autocompressThresholdBytes) {
((HttpProtocolAdapter) super.getHandler()).setAutoCompressThresholdBytes(autocompressThresholdBytes);
}
/**
* {@inheritDoc}
*/
public int getAutoCompressThresholdBytes() {
return ((HttpProtocolAdapter) super.getHandler()).getAutoCompressThresholdBytes();
}
/**
* {@inheritDoc}
*/
public void setAutoUncompress(boolean isAutoUncompress) {
((HttpProtocolAdapter) super.getHandler()).setAutoUncompress(isAutoUncompress);
}
/**
* {@inheritDoc}
*/
public final boolean isAutoUncompress() {
return ((HttpProtocolAdapter) super.getHandler()).isAutoUncompress();
}
/**
*{@inheritDoc}
*/
public long getRequestTimeoutMillis() {
return ((HttpProtocolAdapter) super.getHandler()).getRequestTimeoutMillis();
}
/**
* {@inheritDoc}
*/
public final void setBodyDataReceiveTimeoutMillis(long bodyDataReceiveTimeoutMillis) {
((HttpProtocolAdapter) super.getHandler()).setBodyDataReceiveTimeoutMillis(bodyDataReceiveTimeoutMillis);
}
/**
* {@inheritDoc}
*/
public long getBodyDataReceiveTimeoutMillis() {
return ((HttpProtocolAdapter) super.getHandler()).getBodyDataReceiveTimeoutMillis();
}
/**
* {@inheritDoc}
*/
public void setSessionMaxInactiveIntervalSec(int sessionMaxInactiveIntervalSec) {
((HttpProtocolAdapter) super.getHandler()).setSessionMaxInactiveIntervalSec(sessionMaxInactiveIntervalSec);
}
/**
* {@inheritDoc}
*/
public int getSessionMaxInactiveIntervalSec() {
return ((HttpProtocolAdapter) super.getHandler()).getSessionMaxInactiveIntervalSec();
}
/**
* {@inheritDoc}
*/
public void setCloseOnSendingError(boolean isCloseOnSendingError) {
((HttpProtocolAdapter) super.getHandler()).setCloseOnSendingError(isCloseOnSendingError);
}
/**
* {@inheritDoc}
*/
public boolean isCloseOnSendingError() {
return ((HttpProtocolAdapter) super.getHandler()).isCloseOnSendingError();
}
/**
*{@inheritDoc}
*/
public void setRequestBodyDefaultEncoding(String defaultEncoding) {
((HttpProtocolAdapter) super.getHandler()).setRequestBodyDefaultEncoding(defaultEncoding);
}
/**
*{@inheritDoc}
*/
public String getRequestBodyDefaultEncoding() {
return ((HttpProtocolAdapter) super.getHandler()).getRequestBodyDefaultEncoding();
}
/**
* {@inheritDoc}
*/
public void setMaxTransactions(int maxTransactions) {
((HttpProtocolAdapter) getHandler()).setMaxTransactions(maxTransactions);
}
/**
* {@inheritDoc}
*/
public int getMaxTransactions() {
return ((HttpProtocolAdapter) getHandler()).getMaxTransactions();
}
/**
* {@inheritDoc}
*/
public void setSessionManager(ISessionManager sessionManager) {
((HttpProtocolAdapter) getHandler()).setSessionManager(sessionManager);
}
/**
* {@inheritDoc}
*/
public ISessionManager getSessionManager() {
return ((HttpProtocolAdapter) getHandler()).getSessionManager();
}
/**
* {@inheritDoc}
*/
public void setUsingCookies(boolean useCookies) {
((HttpProtocolAdapter) getHandler()).setUsingCookies(useCookies);
}
/**
* {@inheritDoc}
*/
public boolean isUsingCookies() {
return ((HttpProtocolAdapter) getHandler()).isUsingCookies();
}
/**
* returns the transaction log
* @return the transaction log
*/
List getTransactionInfos() {
List result = new ArrayList();
for (Transaction transaction : transactionLog.getTransactions()) {
result.add(transaction.toString());
}
return result;
}
/**
* sets the max size of the transaction log
*
* @param maxSize the max size of the transaction log
*/
void setTransactionLogMaxSize(int maxSize) {
transactionLog.setMaxSize(maxSize);
if (maxSize == 0) {
transactionMonitor = null;
} else {
transactionMonitor = new TransactionMonitor(transactionLog);
}
((HttpProtocolAdapter) getHandler()).setTransactionMonitor(transactionMonitor);
}
Integer getTransactionsPending() {
if (transactionMonitor != null) {
return transactionMonitor.getPendingTransactions();
} else {
return null;
}
}
/**
* get the max size of the transaction log
*
* @return the max size of the transaction log
*/
int getTransactionLogMaxSize() {
return transactionLog.getMaxSize();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy