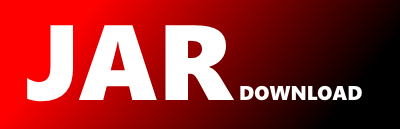
org.xlightweb.IHttpMessage Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb;
import java.io.IOException;
import java.util.Enumeration;
import java.util.List;
import java.util.Set;
/**
* Http Message
* @author [email protected]
*/
public interface IHttpMessage {
public static final String GET_METHOD = "GET";
public static final String POST_METHOD = "POST";
public static final String HEAD_METHOD = "HEAD";
public static final String PUT_METHOD = "PUT";
public static final String DELETE_METHOD = "DELETE";
public static final String TRACE_METHOD = "TRACE";
public static final String CONNECT_METHOD = "CONNECT";
public static final String OPTIONS_METHOD = "OPTIONS";
/**
* returns the non-blocking body
*
* @return the body or null
if message is bodyless
*/
NonBlockingBodyDataSource getNonBlockingBody() throws IOException;
/**
* returns the blocking body
*
* @return the body or null
if message is bodyless
*/
BlockingBodyDataSource getBlockingBody() throws IOException;
/**
* returns true if the message has a body
*
* @return true, if the message has a body
*/
boolean hasBody();
/**
* Adds a header with the given name and value.
* This method allows headers to have multiple values.
*
* @param headername the name of the header
* @param headervalue the additional header value. If it contains octet string, it should be encoded
* according to RFC 2047 (http://www.ietf.org/rfc/rfc2047.txt)
*/
void addHeader(String headername, String headervalue);
/**
* Sets a header with the given name and value.
* If the header had already been set, the new value overwrites the
* previous one.
*
* @param headername the name of the header
* @param headervalue the header value If it contains octet string,
* it should be encoded according to RFC 2047 (http://www.ietf.org/rfc/rfc2047.txt)
*/
void setHeader(String headername, String headervalue);
/**
* removes a header with the given name
*
* @param headername the name of the header
*/
void removeHeader(String headername);
/**
* Returns a boolean indicating whether the named header
* has already been set.
*
* @param name the header name
* @return true
if the named header has already been set; false
otherwise
*/
boolean containsHeader(String headername);
/**
* Returns an set of all the header names.
* If the message has no headers, this method
* returns an empty Set.
*
* @return an Set of all the header names
*
*/
Set getHeaderNameSet();
/**
* Returns an enumeration of all the header names.
* If the message has no headers, this method
* returns an empty enumeration.
*
* @return an enumeration of all the header names
*
*/
@SuppressWarnings("unchecked")
Enumeration getHeaderNames();
/**
* Returns all the values of the specified header as an
* List
of String
objects.
*
* Some headers, such as Accept-Language
can be sent
* by clients as several headers each with a different value rather than
* sending the header as a comma separated list.
*
*
* @param headername a String
specifying the header name
* @return an List
containing the values of the requested header.
* If the message does not have any headers of that name return an empty enumeration.
*/
List getHeaderList(String headername);
/**
* Returns all the values of the specified header as an
* Enumeration
of String
objects.
*
* Some headers, such as Accept-Language
can be sent
* by clients as several headers each with a different value rather than
* sending the header as a comma separated list.
*
*
* @param headername a String
specifying the header name
* @return an Enumeration
containing the values of the requested header.
* If the message does not have any headers of that name return an empty enumeration.
*/
@SuppressWarnings("unchecked")
Enumeration getHeaders(String headername);
/**
* adds a raw header line
*
* @param line the headerline
*/
void addHeaderLine(String line);
/**
* Returns the value of the specified header as a String
.
* If the message did not include a header of the specified name,
* this method returns null
.
*
* If there are multiple headers with the same name, this method
* returns the first head in the message.
*
* @param headername a String
specifying the header name
* @return a String
containing the value of the
* requested header, or null
if the message
* does not have a header of that name
*/
String getHeader(String headername);
/**
* Returns the length, in bytes, of the message body
* and made available by the input stream, or -1 if the
* length is not known.
*
* @return an integer containing the length of the message body or -1 if the length is not known
*/
int getContentLength();
/**
* sets the content length in bytes
*
* @param length the content length in bytes
*/
void setContentLength(int length);
/**
* sets the MIME type of the body of the message
*
* @param type the MIME type of the body of the message
*/
void setContentType(String type);
/**
* Returns the MIME type of the body of the messag, or
* null
if the type is not known.
*
* @return a String
containing the name of the MIME type of
* the message, or null if the type is not known
*/
String getContentType();
/**
* returns the Transfer-Encoding header parameter or null
if the header is not set
*
* @return the Transfer-Encoding header parameter or null
if the header is not set
*/
String getTransferEncoding();
/**
* sets the Transfer-Encoding parameter
*
* @param transferEncoding the Transfer-Encoding parameter
*/
void setTransferEncoding(String transferEncoding);
/**
* Returns the name of the character encoding used in the body of this
* message.
*
* @return a String
containing the name of the character encoding
*
*/
String getCharacterEncoding();
/**
* returns the message header
*
* @return the message header
*/
IHttpHeader getMessageHeader();
/**
* Returns the name and version of the protocol the message
* uses in the form protocol/majorVersion.minorVersion, for example, HTTP/1.1.
*
* @return a String containing the protocol name and version number
*/
String getProtocol();
/**
* Returns the version of the protocol the message
* uses in the form majorVersion.minorVersion, for example, 1.1.
*
* @return a String containing the protocol version number
*/
String getProtocolVersion();
/**
* Stores an attribute in this header. Attributes are reset between messages.
*
*
Attribute names should follow the same conventions as
* package names.
*
* @param name a String
specifying
* the name of the attribute
* @param o the Object
to be stored
*
*/
void setAttribute(String name, Object o);
/**
* Returns the value of the named attribute as an Object
,
* or null
if no attribute of the given name exists.
*
*
Attribute names should follow the same conventions as package
* names.
*
* @param name a String
specifying the name of the attribute
* @return an Object
containing the value of the attribute,
* or null
if the attribute does not exist
*/
Object getAttribute(String name);
/**
* Returns an Enumeration
containing the
* names of the attributes available to this message.
* This method returns an empty Enumeration
* if the message has no attributes available to it.
*
*
* @return an Enumeration
of strings containing the names
* of the message's attributes
*
*/
@SuppressWarnings("unchecked")
Enumeration getAttributeNames();
/**
* Returns an Set
containing the
* names of the attributes available to this messaget.
* This method returns an empty Set
* if the request has no attributes available to it.
*
*
* @return an Set
of strings
* containing the names of the message's attributes
*
*/
Set getAttributeNameSet();
}