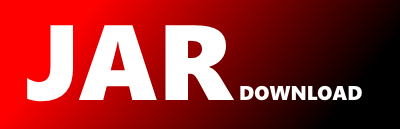
org.xlightweb.HttpResponseHeader Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
/**
* http response header
*
* @author [email protected]
*/
public final class HttpResponseHeader extends AbstractHttpHeader implements IHttpResponseHeader {
// response elements
private int statusCode = -1;
private String reason;
private boolean isSimpleResponse = false;
// cache
private String server;
private String date;
/**
* constructor
*
*/
HttpResponseHeader() {
setProtocolSchemeSilence("HTTP");
}
/**
* constructor
*
* @param statusCode the status code
*/
public HttpResponseHeader(int statusCode) {
this(statusCode, null, HttpUtils.getReason(statusCode));
}
/**
* constructor. The status will be set to 200
*
* @param contentType the content type
*/
public HttpResponseHeader(String contentType) {
this(200, contentType);
}
/**
* constructor
*
* @param statusCode the status code
* @param contentType the content type
*/
public HttpResponseHeader(int statusCode, String contentType) {
this(statusCode, contentType, HttpUtils.getReason(statusCode));
}
/**
* constructor
*
* @param statusCode the status code
* @param contentType the content type
* @param reason the reason
*/
public HttpResponseHeader(int statusCode, String contentType, String reason) {
this("1.1", statusCode, contentType, reason);
}
private HttpResponseHeader(String protocolVersion, int statusCode, String contentType, String reason) {
setProtocolSchemeSilence("HTTP");
setProtocolVersionSilence(protocolVersion);
this.statusCode = statusCode;
this.reason = reason;
if (contentType != null) {
setContentType(contentType);
}
}
/**
* sets if this response is a simple response
* @param isSimpleResponse flag, if this response is a simple response
*/
void setSimpleResponse(boolean isSimpleResponse) {
this.isSimpleResponse = isSimpleResponse;
if (isSimpleResponse) {
setProtocolVersionSilence("0.9");
}
}
/**
* {@inheritDoc}
*/
public void copyHeaderFrom(HttpResponseHeader otherHeader, String... upperExcludenames) {
super.copyHeaderFrom(otherHeader, upperExcludenames);
}
/**
* {@inheritDoc}
*/
public int getStatus() {
return statusCode;
}
/**
* {@inheritDoc}
*/
public void setStatus(int status) {
this.statusCode = status;
}
/**
* {@inheritDoc}
*/
public String getReason() {
return reason;
}
/**
* {@inheritDoc}
*/
public void setReason(String reason) {
this.reason = reason;
}
/**
* {@inheritDoc}
*/
public void setProtocol(String protocol) {
int idx = protocol.indexOf("/");
setProtocolSchemeSilence(protocol.substring(0, idx));
setProtocolVersionSilence(protocol.substring(idx + 1, protocol.length()));
}
/**
* {@inheritDoc}
*/
@Override
public void setDate(String date) {
this.date = date;
}
/**
* {@inheritDoc}
*/
@Override
public String getDate() {
return date;
}
/**
* {@inheritDoc}
*/
@Override
public void setServer(String server) {
this.server = server;
}
/**
* {@inheritDoc}
*/
@Override
public String getServer() {
return server;
}
/**
* {@inheritDoc}
*/
@Override
protected boolean onHeaderAdded(String headername, String headervalue) {
if (headername.equalsIgnoreCase("Server")) {
server = headervalue;
return true;
}
if (headername.equalsIgnoreCase("Date")) {
date = headervalue;
return true;
}
return super.onHeaderAdded(headername, headervalue);
}
/**
* {@inheritDoc}
*/
@Override
protected boolean onHeaderRemoved(String headername) {
if (headername.equalsIgnoreCase("Server")) {
server = null;
return true;
}
if (headername.equalsIgnoreCase("Date")) {
date = null;
return true;
}
return super.onHeaderRemoved(headername);
}
/**
* {@inheritDoc}
*/
public Set getHeaderNameSet() {
Set headerNames = super.getHeaderNameSet();
if (server != null) {
headerNames.add(SERVER);
}
if (date != null) {
headerNames.add(DATE);
}
return headerNames;
}
/**
* {@inheritDoc}
*/
public List getHeaderList(String headername) {
if (headername.equalsIgnoreCase(SERVER)) {
if (server == null) {
return null;
}
List result = new ArrayList();
result.add(server);
return Collections.unmodifiableList(result);
}
if (headername.equalsIgnoreCase(DATE)) {
if (date == null) {
return null;
}
List result = new ArrayList();
result.add(date);
return Collections.unmodifiableList(result);
}
return super.getHeaderList(headername);
}
/**
* {@inheritDoc}
*/
public String getHeader(String headername) {
if (headername.equalsIgnoreCase(SERVER)) {
if (server == null) {
return null;
}
return server;
}
if (headername.equalsIgnoreCase(DATE)) {
if (date == null) {
return null;
}
return date;
}
return super.getHeader(headername);
}
/**
* {@inheritDoc}
*/
public boolean containsHeader(String headername) {
if (headername.equalsIgnoreCase(SERVER)) {
return (server != null);
}
if (headername.equalsIgnoreCase(DATE)) {
return (date != null);
}
return super.containsHeader(headername);
}
/**
* {@inheritDoc}
*/
@Override
protected Object clone() throws CloneNotSupportedException {
HttpResponseHeader copy = (HttpResponseHeader) super.clone();
return copy;
}
/**
* {@inheritDoc}
*/
public IHttpResponseHeader copy() {
try {
return (IHttpResponseHeader) this.clone();
} catch (CloneNotSupportedException cnse) {
throw new RuntimeException(cnse.toString());
}
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
if (isSimpleResponse) {
return "";
}
StringBuilder sb = new StringBuilder(256);
if (getProtocolScheme() != null) {
sb.append(getProtocolScheme());
sb.append("/");
sb.append(getProtocolVersion());
sb.append(" ");
}
sb.append(statusCode);
if (reason != null) {
sb.append(" ");
sb.append(reason);
}
sb.append("\r\n");
if (server != null) {
sb.append("Server: ");
sb.append(server);
sb.append("\r\n");
}
if (date != null) {
sb.append("Date: ");
sb.append(date);
sb.append("\r\n");
}
writeHeadersTo(sb);
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy